A veces, cuando hacemos clic en un elemento web en particular, se abre una nueva ventana. Para ubicar los elementos web en la página web de la nueva ventana, debemos cambiar el enfoque de Selenium de la página actual (página principal) a la nueva página. En este artículo, intentaremos cambiar el enfoque de Selenium de una ventana a otra ventana nueva. Aquí usaremos el navegador chrome para lo cual requerimos ChromeDriver puedes descargarlo desde el sitio oficial de selenium. Usaremos el sitio web geeksforgeeks.org para la práctica de automatización.
Pasos
- Visite el sitio oficial de geeksforgeeks.org
- Haga clic en cursos en GeeksofGeeks desde enlaces rápidos
- Seleccione un curso en particular (Abrir en una nueva ventana)
- Haga clic en el elemento de la nueva ventana
Resultado esperado: debe hacer clic en el elemento de la nueva ventana
Implementación
Programa 1: Haga clic en el elemento de la nueva ventana sin cambiar el foco de selenium
Java
import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class GFGWindowHandling { public static void main(String[] args) throws InterruptedException { // we are using chrome driver System.setProperty("webdriver.chrome.driver", "Path of chromedriver.exe"); WebDriver driver = new ChromeDriver(); // etering the URL driver.get("https:// www.geeksforgeeks.org/"); // to maximize the window driver.manage().window().maximize(); // to delete all cookies driver.manage().deleteAllCookies(); // to Scroll the screen to locate element JavascriptExecutor je = (JavascriptExecutor)driver; je.executeScript("window.scrollBy(0, 200)"); driver.findElement(By.xpath("(// span[text()='Courses at GeeksforGeeks'])[2]")).click(); // to select a particular course Thread.sleep(2000); driver.findElement(By.xpath("(// h4[text()='Data Structures and Algorithms - Self Paced'])[1]")).click(); // it will open with new tab // to click on (Read more here) on new window driver.findElement(By.xpath("(// a[text()='(Read more here)'])[1]")).click(); // statement to understand that operation is performed on new window System.out.println("operation is performed on new window"); } }
Salida de la consola:
org.openqa.selenium.NoSuchElementException: no such element: Unable to locate element: {"method":"xpath", "selector":"(//a)[1]"}
Esta excepción se debe a que cuando hacemos clic en un curso en particular, se abre una nueva ventana (ventana secundaria) y cuando tratamos de hacer clic en Leer más aquí, en ese momento el foco de Selenium está en la ventana principal (ventana principal). Así que usaremos algún método para cambiar el enfoque de selenium de una ventana a otra.
Sintaxis:
controlador.switchTo().window(ID);
Donde ID: ID de una ventana en particular en la que necesita cambiar el foco de selenium.
Para obtener el ID de Windows usaremos el siguiente método.
- driver.getWindowHandle() : para obtener el ID de la ventana principal (ventana principal)
- driver.getWindowHandles() : para obtener el ID de las ventanas secundarias (ventana nueva)
Observemos cómo los ID de dos ventanas diferentes son diferentes,
Programa 2: Para obtener los ID de diferentes ventanas
Java
import java.util.Set; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class GFGIDsOfWindows { public static void main(String[] args) throws InterruptedException { // we are using chrome driver System.setProperty("webdriver.chrome.driver", "Path of chromedriver.exe"); WebDriver driver = new ChromeDriver(); // etering the URL driver.get("https:// www.geeksforgeeks.org/"); // to maximize the window driver.manage().window().maximize(); // to delete all cookies driver.manage().deleteAllCookies(); // to Scroll the screen to locate element JavascriptExecutor je = (JavascriptExecutor)driver; je.executeScript("window.scrollBy(0, 200)"); driver.findElement(By.xpath("(// span[text()='Courses at GeeksforGeeks'])[2]")).click(); // to select a particular course Thread.sleep(2000); driver.findElement(By.xpath("(// h4[text()='Data Structures and Algorithms - Self Paced'])[1]")).click(); // it will open with new tab // getWindowHandle method to get ID of main window(parent window) String Parent_id = driver.getWindowHandle(); System.out.println(Parent_id); // getWindowHandle method to get ID of new window (child window) Set<String> Child_id = driver.getWindowHandles(); // for each loop for (String a : Child_id) { // it will print IDs of both window System.out.println(a); } } }
Salida de la consola:
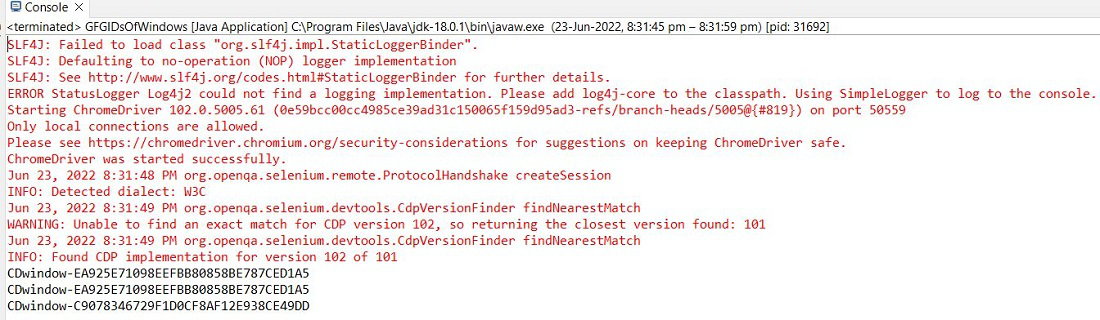
Aquí puede observar que los ID de las ventanas son diferentes.
CDwindow-EA925E71098EEFBB80858BE787CED1A5 (ID of main window) CDwindow-C9078346729F1D0CF8AF12E938CE49DD (ID of new window)
Entonces, para cambiar el enfoque de Selenium de una ventana a otra, usaremos For each loop y proporcionaremos la condición if-else. Para cada bucle
for( Datatype variable : collection ) { Statements; }
Programa 3: Haga clic en el elemento de la nueva ventana cambiando el foco de selenium
Java
import java.util.Set; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class GFGWindowHandlingEx { public static void main(String[] args) throws InterruptedException { // we are using chrome driver System.setProperty("webdriver.chrome.driver", "Path of chromedriver.exe"); WebDriver driver = new ChromeDriver(); // etering the URL driver.get("https:// www.geeksforgeeks.org/"); // to maximize the window driver.manage().window().maximize(); // to delete all cookies driver.manage().deleteAllCookies(); // to Scroll the screen to locate element JavascriptExecutor je = (JavascriptExecutor)driver; je.executeScript("window.scrollBy(0, 200)"); driver.findElement(By.xpath("(// span[text()='Courses at GeeksforGeeks'])[2]")).click(); // to select a particular course Thread.sleep(2000); driver.findElement(By.xpath("(// h4[text()='Data Structures and Algorithms - Self Paced'])[1]")).click(); // it will open with new tab // getWindowHandle method to get ID of main window(parent window) String Parent_id = driver.getWindowHandle(); System.out.println(Parent_id); // getWindowHandle method to get ID of new window (child window) Set<String> Child_id = driver.getWindowHandles(); // for each loop for (String a : Child_id) { // it will print IDs of both window System.out.println(a); // condition to change the focus of selenium if (Parent_id.equals(a)) { } else { // to change focus on new window driver.switchTo().window(a); Thread.sleep(2000); // to handle the popup regarding cookies driver.findElement(By.xpath("// button[text()='Got it!']")).click(); // to click on (Read more here) je.executeScript("window.scrollBy(0, 600)"); driver.findElement(By.xpath("(// a[text()='(Read more here)'])[1]")).click(); // statement to understand that operation is performed on new window System.out.println("operation is performed on new window"); } } } }
Salida de la consola:
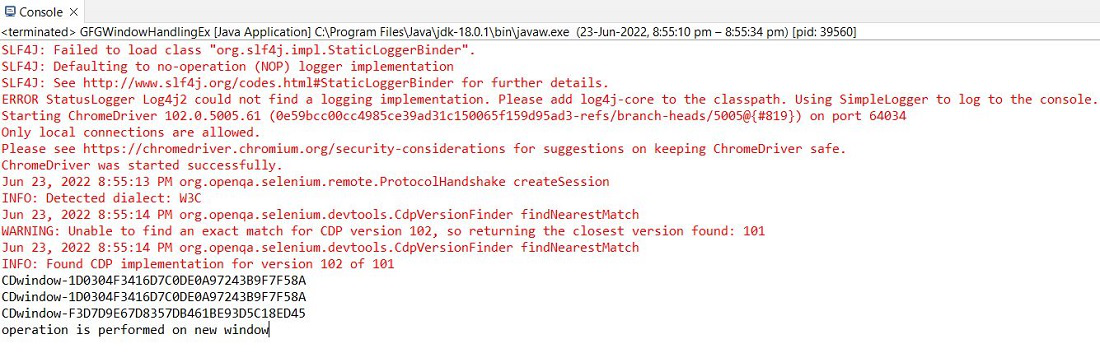
Vídeo de salida
Publicación traducida automáticamente
Artículo escrito por badesuraj9 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA