En este artículo, veremos cómo agregar el interruptor en nuestra aplicación usando KivyMD en Python.
MDSwitch: el interruptor es una especie de botón de alternar que se usa principalmente en las aplicaciones de Android para activar/desactivar funciones. Tiene el siguiente aspecto:
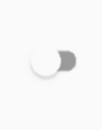
Instalación:
Para instalar los módulos, escriba el siguiente comando en la terminal.
pip instalar kivy
pip instalar kivymd
Método 1: Usando lenguaje kv:
Paso 1. Importe los paquetes necesarios.
Para esto, necesitaremos Builder de kivy y MDApp del paquete kivymd.
Nota: No importaremos MDFloatLayout y MDSwitch porque estamos diseñando nuestra pantalla usando el lenguaje kv.
Python3
# import packages from kivy.lang import Builder from kivymd.app import MDApp
Paso 2. Disposición del diseño.
Estaremos diseñando nuestro diseño usando lenguaje kv.
Primero, declararemos la clase de widget de diseño llamada MDFloatLayout y luego la clase de widget secundaria llamada MDSwitch. No pasaremos ningún parámetro a MDFloatLayout y lo mantendremos predeterminado.
Para MDSwitch, pasaremos su ubicación en forma de coordenadas x, y. center_x se usa para la coordenada x mientras que center_y se usa para la coordenada y.
Python3
# writing kv lang KV = ''' # declaring layout MDFloatLayout: # this will create a switch MDSwitch: # giving position to the switch on screen pos_hint: {'center_x': .5, 'center_y': .5} '''
Paso 3. Escribiendo el programa principal.
Para ejecutar el archivo kv, usaremos load_string() y le pasaremos nuestro idioma kv. Por lo tanto, definiremos una función para este nombre build() y, de guardia, cargará kv y devolverá la pantalla. run() se usa para ejecutar la clase y no requiere ningún parámetro.
Python3
# app class class Test(MDApp): def build(self): # this will load kv lang screen = Builder.load_string(KV) # returning screen return screen # running app Test().run()
Agregando los pasos anteriores:
Python3
# importing packages from kivy.lang import Builder from kivymd.app import MDApp # writing kv lang KV = ''' # declaring layout MDFloatLayout: # this will create a switch MDSwitch: # giving position to the switch on screen pos_hint: {'center_x': .5, 'center_y': .5} ''' # app class class Test(MDApp): def build(self): # this will load kv lang screen = Builder.load_string(KV) # returning screen return screen # running app Test().run()
Producción:
Método 2. Sin lenguaje kv:
Paso 1. Importe los paquetes necesarios.
Para esto, necesitaremos Screen, MDSwitch y MDApp del paquete kivymd.
Python3
# import packages from kivymd.app import MDApp from kivymd.uix.screen import Screen from kivymd.uix.selectioncontrol import MDSwitch
Paso 2. Escribiendo el programa principal.
Para diseñar un diseño primero, necesitaremos un diseño en negro, para lo cual usaremos screen(). Ahora necesitamos definir MDSwitch. Podemos hacerlo usando MDSwitch(). En este método, usamos el mismo parámetro usado en el método 1 que se usará para definir su ubicación en la pantalla usando las coordenadas x, y. Después de todo esto, tenemos que MDSwitch widget a la pantalla y para hacerlo usaremos add.widget(), donde los parámetros serán el widget que queremos agregar a la pantalla. Y eso es todo, ahora ejecutaremos la clase de la aplicación usando run().
Python3
# App class class MainApp(MDApp): def build(self): # defining blank screen/layout. screen = Screen() # defining MDSwitch widget and storing in a variable wid = MDSwitch(pos_hint={'center_x': 0.5, 'center_y': 0.5}) # adding widget to the screen screen.add_widget(wid) # returns screen/layout return screen # running app MainApp().run()
Agregando los pasos anteriores:
Python3
# import packages from kivymd.app import MDApp from kivymd.uix.screen import Screen from kivymd.uix.selectioncontrol import MDSwitch # App class class MainApp(MDApp): def build(self): # defining blank screen/layout. screen = Screen() # defining MDSwitch widget and storing in a variable wid = MDSwitch(pos_hint={'center_x': 0.5, 'center_y': 0.5}) # adding widget to the screen screen.add_widget(wid) # returns screen/layout return screen # running app MainApp().run()
Producción:
Publicación traducida automáticamente
Artículo escrito por vinamrayadav y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA