Python es un lenguaje de programación muy versátil. Python se está utilizando en casi todas las tecnologías principales y se puede desarrollar literalmente cualquier aplicación con él. Veamos un programa en Python para convertir la moneda de un país a la de otro país. Para usar este servicio, se necesita la clave API, que se puede obtener desde aquí . Usaremos la API de reparación para obtener las tasas de conversión en vivo y convertir la cantidad correspondiente.
Caso 1
Módulos necesarios:
1.1: requests: este módulo no se integra con python. Para instalarlo, escriba el siguiente comando en la terminal o cmd.
pip install requests
A continuación se muestra la implementación:
Python3
# Python program to convert the currency # of one country to that of another country # Import the modules needed import requests class Currency_convertor: # empty dict to store the conversion rates rates = {} def __init__(self, url): data = requests.get(url).json() # Extracting only the rates from the json data self.rates = data["rates"] # function to do a simple cross multiplication between # the amount and the conversion rates def convert(self, from_currency, to_currency, amount): initial_amount = amount if from_currency != 'EUR' : amount = amount / self.rates[from_currency] # limiting the precision to 2 decimal places amount = round(amount * self.rates[to_currency], 2) print('{} {} = {} {}'.format(initial_amount, from_currency, amount, to_currency)) # Driver code if __name__ == "__main__": # YOUR_ACCESS_KEY = 'GET YOUR ACCESS KEY FROM fixer.io' url = str.__add__('http://data.fixer.io/api/latest?access_key=', YOUR_ACCESS_KEY) c = Currency_convertor(url) from_country = input("From Country: ") to_country = input("TO Country: ") amount = int(input("Amount: ")) c.convert(from_country, to_country, amount)
Aporte :
From Country: USD TO Country: INR Amount: 1
Producción :
1 USD = 70.69 INR
Caso 2
Módulos necesarios:
2.1: tkinter : Facilita interfaces gráficas de usuario (GUIs).
pip install tkinter
2.2: forex_python: es una biblioteca gratuita de tipos de cambio y conversión de divisas .
pip install forex_python
Implementación:
Python
#pip install tkinter import tkinter as tk from tkinter import * import tkinter.messagebox #GUI root = tk.Tk() root.title("Currency converter:GeeksForGeeks") Tops = Frame(root, bg = '#e6e5e5', pady=2, width=1850, height=100, relief="ridge") Tops.grid(row=0, column=0) headlabel = tk.Label(Tops, font=('lato black', 19, 'bold'), text='Currency converter :GeeksForGeeks ', bg='#e6e5e5', fg='black') headlabel.grid(row=1, column=0, sticky=W) variable1 = tk.StringVar(root) variable2 = tk.StringVar(root) variable1.set("currency") variable2.set("currency") #Function To For Real Time Currency Conversion def RealTimeCurrencyConversion(): from forex_python.converter import CurrencyRates c = CurrencyRates() from_currency = variable1.get() to_currency = variable2.get() if (Amount1_field.get() == ""): tkinter.messagebox.showinfo("Error !!", "Amount Not Entered.\n Please a valid amount.") elif (from_currency == "currency" or to_currency == "currency"): tkinter.messagebox.showinfo("Error !!", "Currency Not Selected.\n Please select FROM and TO Currency form menu.") else: new_amt = c.convert(from_currency, to_currency, float(Amount1_field.get())) new_amount = float("{:.4f}".format(new_amt)) Amount2_field.insert(0, str(new_amount)) #clearing all the data entered by the user def clear_all(): Amount1_field.delete(0, tk.END) Amount2_field.delete(0, tk.END) CurrenyCode_list = ["INR", "USD", "CAD", "CNY", "DKK", "EUR"] root.configure(background='#e6e5e5') root.geometry("700x400") Label_1 = Label(root, font=('lato black', 27, 'bold'), text="", padx=2, pady=2, bg="#e6e5e5", fg="black") Label_1.grid(row=1, column=0, sticky=W) label1 = tk.Label(root, font=('lato black', 15, 'bold'), text="\t Amount : ", bg="#e6e5e5", fg="black") label1.grid(row=2, column=0, sticky=W) label1 = tk.Label(root, font=('lato black', 15, 'bold'), text="\t From Currency : ", bg="#e6e5e5", fg="black") label1.grid(row=3, column=0, sticky=W) label1 = tk.Label(root, font=('lato black', 15, 'bold'), text="\t To Currency : ", bg="#e6e5e5", fg="black") label1.grid(row=4, column=0, sticky=W) label1 = tk.Label(root, font=('lato black', 15, 'bold'), text="\t Converted Amount : ", bg="#e6e5e5", fg="black") label1.grid(row=8, column=0, sticky=W) Label_1 = Label(root, font=('lato black', 7, 'bold'), text="", padx=2, pady=2, bg="#e6e5e5", fg="black") Label_1.grid(row=5, column=0, sticky=W) Label_1 = Label(root, font=('lato black', 7, 'bold'), text="", padx=2, pady=2, bg="#e6e5e5", fg="black") Label_1.grid(row=7, column=0, sticky=W) FromCurrency_option = tk.OptionMenu(root, variable1, *CurrenyCode_list) ToCurrency_option = tk.OptionMenu(root, variable2, *CurrenyCode_list) FromCurrency_option.grid(row=3, column=0, ipadx=45, sticky=E) ToCurrency_option.grid(row=4, column=0, ipadx=45, sticky=E) Amount1_field = tk.Entry(root) Amount1_field.grid(row=2, column=0, ipadx=28, sticky=E) Amount2_field = tk.Entry(root) Amount2_field.grid(row=8, column=0, ipadx=31, sticky=E) Label_9 = Button(root, font=('arial', 15, 'bold'), text=" Convert ", padx=2, pady=2, bg="lightblue", fg="white", command=RealTimeCurrencyConversion) Label_9.grid(row=6, column=0) Label_1 = Label(root, font=('lato black', 7, 'bold'), text="", padx=2, pady=2, bg="#e6e5e5", fg="black") Label_1.grid(row=9, column=0, sticky=W) Label_9 = Button(root, font=('arial', 15, 'bold'), text=" Clear All ", padx=2, pady=2, bg="lightblue", fg="white", command=clear_all) Label_9.grid(row=10, column=0) root.mainloop()
Producción:
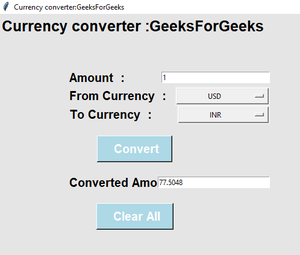
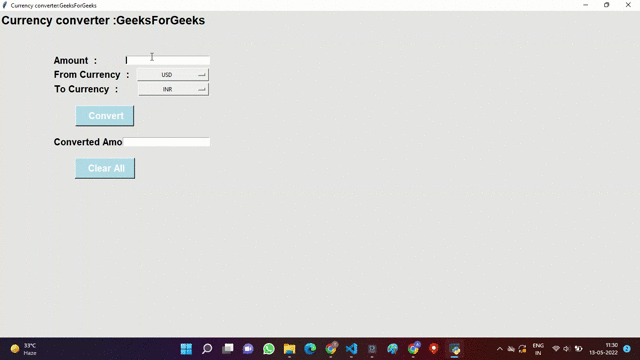
Publicación traducida automáticamente
Artículo escrito por TsaiDeepak y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA