Según Wikipedia, la compresión de datos o la compresión de archivos se puede entender como un proceso de reducción del tamaño de un archivo/carpeta/cualquier dato en particular mientras se conservan los datos originales. Un tamaño de archivo menor tiene muchos beneficios, ya que ocupará un área de almacenamiento menor, lo que le brinda más espacio para que lo ocupen otros datos, transfiera el archivo más rápido ya que el tamaño es menor y varias otras ventajas se desbloquean al comprimir el archivo. Los archivos comprimidos se almacenan en forma de carpetas de extensión de archivo comprimido como » .zip «, » .rar «, » .tar.gz «, » .arj » y » .tgz «.“. La compresión reduce el tamaño del archivo al tamaño máximo de compresión. Si no se puede comprimir más, el tamaño seguirá siendo el mismo y no menor.
A continuación se muestran algunos ejemplos para ver cómo funciona la compresión en Golang. ¿Cuáles son los métodos incorporados que podemos usar? ¿Cómo se puede rastrear un archivo usando su ruta? ¿Cómo podemos abrir el archivo? ¿Cómo funciona la compresión? Veamos el primer ejemplo.
Ejemplo 1:
- Para comprimir un archivo en Golang, usamos el comando gzip.
- Luego crea una ruta para rastrear nuestro archivo.
- Luego deje el comando para leer nuestro archivo.
A continuación el programa lee en un archivo. Utiliza ioutil.ReadAll para obtener todos los bytes del archivo. Después, creará un nuevo archivo, es decir, un archivo comprimido, reemplazando la extensión con » gz «.
Nota: Para verificar, puede abrir el archivo comprimido usando WinRar o 7-ZIp, etc.
Go
package main // Declare all the libraries needed import ( "bufio" "compress/gzip" "fmt" "io/ioutil" "os" "strings" ) func main() { // Compressing a file takes many steps // In this example we will see how to open // a file // Then read all its components // Create out own file with a gz extension // Read all bytes and copy it into our new file // Close our new file // Let us start now by checking and verifying // each step in detail // Open file on disk. // Mention the name of the text file in quoted marks // Here we have mentioned the name of the variable // which checks the file name as "name_of_file" name_of_file := "Gfg.txt" // After checking this, we would now trace the file path. // Now we would use os.Open command // This command would help us to open the file // This command takes in the path of the file as input. f, _ := os.Open("C://ProgramData//" + name_of_file) // Now let use read the bytes of the document we opened. // Create a Reader to get all the bytes from the file. read := bufio.NewReader(f) // Now we would use the variable Read All to get all the bytes // So we just used variable data which will read all the bytes data, _ := ioutil.ReadAll(read) // Now we would use the extension method // Now with the help of replace command we can // Replace txt file with gz extension // So we would now use the file name to give // this command a boost name_of_file = strings.Replace(name_of_file, ".txt", ".gz", -1) // Open file for writing // Now using the Os.create method we would use the // To store thr information of the file gz extension f, _ = os.Create("C://ProgramData//" + name_of_file) // Write compresses Data // We would use NewWriter to basically // copy all the compressed data w := gzip.NewWriter(f) // With the help of the Writer method, we would // write all the bytes in the data variable // copied from the original file w.Write(data) // We would now close the file. w.Close() // Now we would see a file with gz extension in the below path // This gz extension file we have to open using 7zip tool }
Producción:
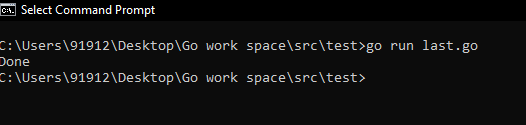
Ejecutando el Programa

Archivo de salida con extensión gz
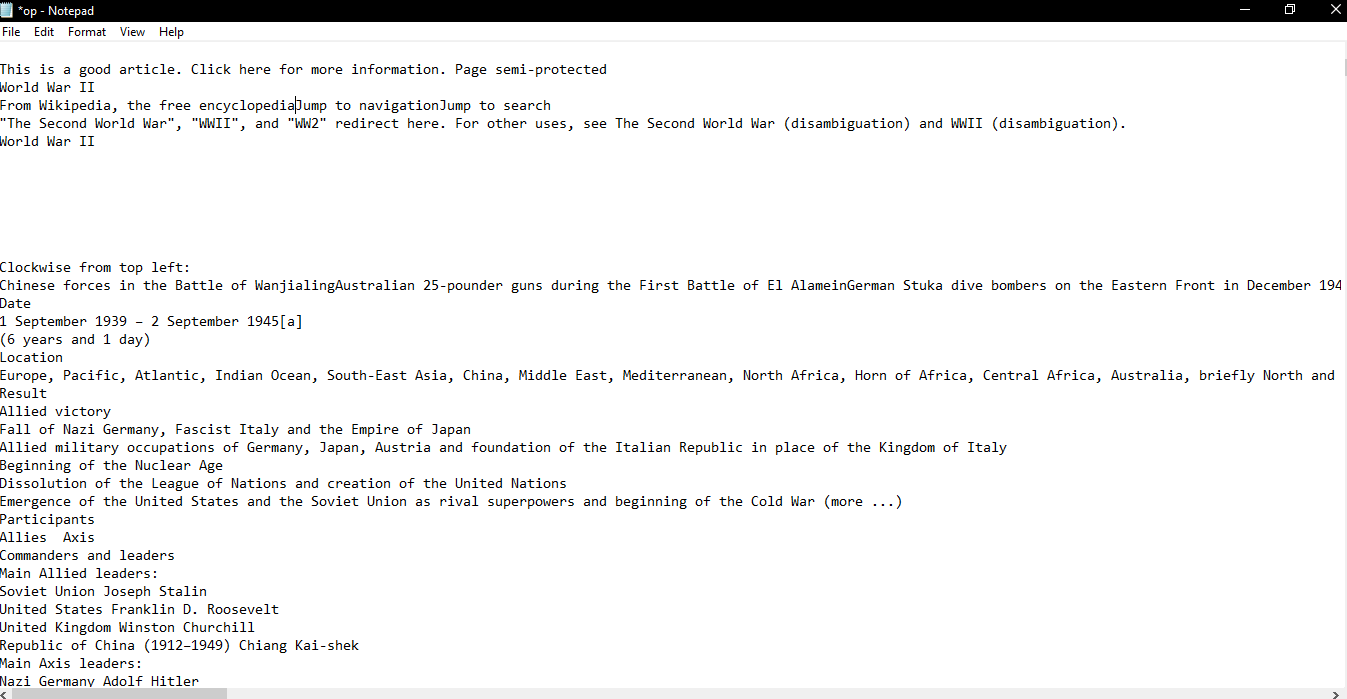
Abriendo el archivo comprimido usando 7-Zip
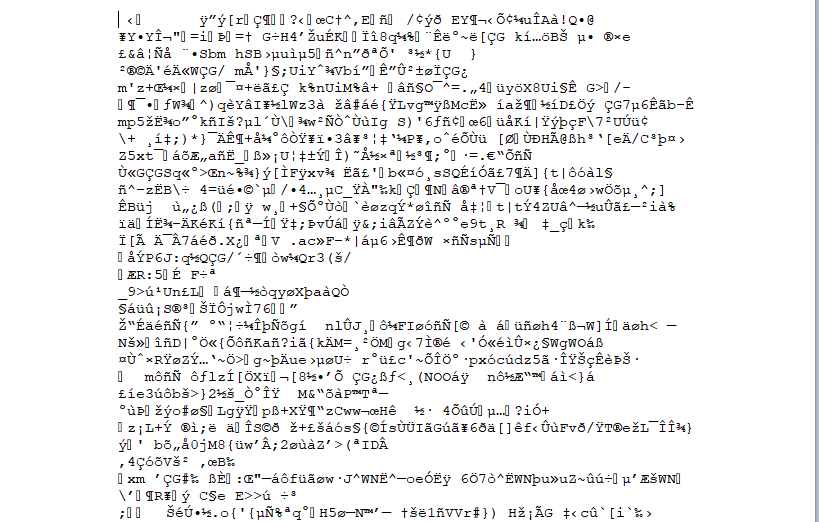
Contenido del archivo comprimido: apertura en el bloc de notas directamente
Ejemplo 2:
Aquí tenemos una string aleatoria. Básicamente, aquí el usuario quiere escribir una versión comprimida de esta string en un archivo en el disco. Así que primero tiene que crear un archivo con os.Create . Ahora use NewWriter para crear un escritor gzip dirigido a ese archivo que creamos. Ahora llama a Write() para escribir bytes. Convierta la string en un valor de byte utilizando la expresión de conversión.
Go
package main // Declare all the libraries which are needed. import ( "compress/gzip" "fmt" "os" ) func main() { // Here we use random text // We would now put that random text into a file we created. // Once we create a random file we created, we would use // that for compression // And then we would check its output. // Now first let us create a random string variable named text // This random text will store some characters. text := "Geeks for geeks" // With the help of os.Create command let us // first open a file named "file.gz" // Open a file for writing f, _ := os.Create("C:\\ProgramData\\file.gz") // Create a gzip writer // Now we the help of the NewWriter command we simply try // to copy all the files into variable "f" // Let us name this variable p p := gzip.NewWriter(f) // Now with the help of the Write command we will // use all the bytes to write it in the file // named text. p.Write([]byte(text)) // Once we are done copying all the files // we would leave the command "close". // Close the file p.Close() // Now once we are done. To,notify our work // We would print the statement "Done" fmt.Println("Done") }
Producción:
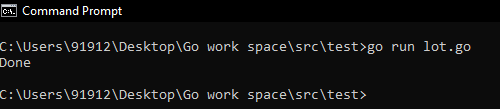
Ejecutando el código

Archivo tomado
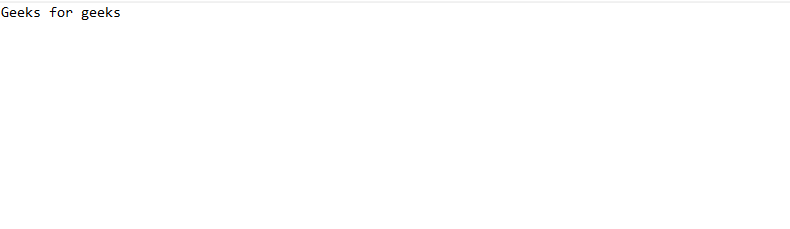
Salida de archivo comprimido usando 7 Zip Tool

Abrir el archivo comprimido en el Bloc de notas
Publicación traducida automáticamente
Artículo escrito por saransh9342 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA