En este artículo, vamos a contar valores en el marco de datos de Pandas. Primero, crearemos un marco de datos y luego contaremos los valores de diferentes atributos.
Sintaxis: DataFrame.count(axis=0, level=Ninguno, numeric_only=False)
Parámetros:
- eje {0 o ‘índice’, 1 o ‘columnas’}: predeterminado 0 Se generan recuentos para cada columna si eje=0 o eje=’índice’ y se generan recuentos para cada fila si eje=1 o eje=”columnas” .
- level (nt o str, opcional): si el eje es un MultiIndex, cuente a lo largo de un nivel particular, colapsando en un DataFrame. Una string especifica el nombre del nivel.
- numeric_only (booleano, predeterminado Falso): Incluye solo valor int, float o booleano.
Devoluciones: devuelve el recuento de valores no nulos y, si se usa el nivel, devuelve el marco de datos
Enfoque paso a paso:
Paso 1: Importación de bibliotecas.
Python3
# importing libraries import numpy as np import pandas as pd
Paso 2: Crear marco de datos
Python3
# Creating dataframe with # some missing values NaN = np.nan dataframe = pd.DataFrame({'Name': ['Shobhit', 'Vaibhav', 'Vimal', 'Sourabh', 'Rahul', 'Shobhit'], 'Physics': [11, 12, 13, 14, NaN, 11], 'Chemistry': [10, 14, NaN, 18, 20, 10], 'Math': [13, 10, 15, NaN, NaN, 13]}) display(dataframe)
Producción:
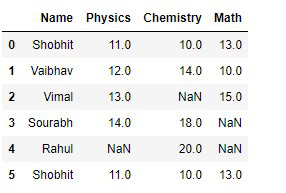
Marco de datos creado
Paso 3: En este paso, simplemente usamos la función .count() para contar todos los valores de diferentes columnas.
Python3
# using dataframe.count() # to count all values dataframe.count()
Producción:
Podemos ver que hay una diferencia en el valor de conteo ya que nos faltan valores. Hay 5 valores en la columna Nombre, 4 en Física y Química y 3 en Matemáticas. En este caso, usa su argumento con sus valores predeterminados.
Paso 4: si queremos contar todos los valores con respecto a la fila, debemos pasar eje = 1 o ‘columnas’.
Python3
# we can pass either axis=1 or # axos='columns' to count with respect to row print(dataframe.count(axis = 1)) print(dataframe.count(axis = 'columns'))
Producción:
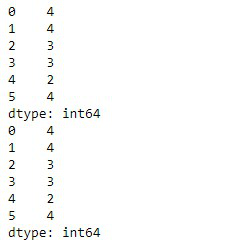
contar con respecto a la fila
Paso 5: Ahora, si queremos contar valores nulos en nuestro marco de datos.
Python3
# it will give the count # of individual columns count of null values print(dataframe.isnull().sum()) # it will give the total null # values present in our dataframe print("Total Null values count: ", dataframe.isnull().sum().sum())
Producción:
Paso 6: . Algunos ejemplos para usar .count()
Ahora queremos contar ninguno de los estudiantes cuyas calificaciones en física sean mayores de 11.
Python3
# count of student with greater # than 11 marks in physics print("Count of students with physics marks greater than 11 is->", dataframe[dataframe['Physics'] > 11]['Name'].count()) # resultant of above dataframe dataframe[dataframe['Physics']>11]
Producción:
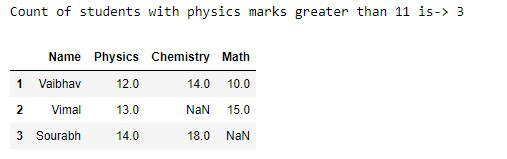
Física>11
Conteo de estudiantes cuyas calificaciones en física son mayores a 10, calificaciones en química mayores a 11 y calificaciones en matemáticas mayores a 9.
Python3
# Count of students whose physics marks # are greater than 10,chemistry marks are # greater than 11 and math marks are greater than 9. print("Count of students ->", dataframe[(dataframe['Physics'] > 10) & (dataframe['Chemistry'] > 11) & (dataframe['Math'] > 9)]['Name'].count()) # dataframe of above result dataframe[(dataframe['Physics'] > 10 ) & (dataframe['Chemistry'] > 11 ) & (dataframe['Math'] > 9 )]
Producción:
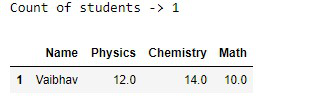
Física>10, Química>11, Matemáticas>9
A continuación se muestra la implementación completa:
Python3
# importing Libraries import pandas as pd import numpy as np # Creating dataframe using dictionary NaN = np.nan dataframe = pd.DataFrame({'Name': ['Shobhit', 'Vaibhav', 'Vimal', 'Sourabh', 'Rahul', 'Shobhit'], 'Physics': [11, 12, 13, 14, NaN, 11], 'Chemistry': [10, 14, NaN, 18, 20, 10], 'Math': [13, 10, 15, NaN, NaN, 13]}) print("Created Dataframe") print(dataframe) # finding Count of all columns print("Count of all values wrt columns") print(dataframe.count()) # Count according to rows print("Count of all values wrt rows") print(dataframe.count(axis=1)) print(dataframe.count(axis='columns')) # count of null values print("Null Values counts ") print(dataframe.isnull().sum()) print("Total null values", dataframe.isnull().sum().sum()) # count of student with greater # than 11 marks in physics print("Count of students with physics marks greater than 11 is->", dataframe[dataframe['Physics'] > 11]['Name'].count()) # resultant of above dataframe print(dataframe[dataframe['Physics'] > 11]) print("Count of students ->", dataframe[(dataframe['Physics'] > 10) & (dataframe['Chemistry'] > 11) & (dataframe['Math'] > 9)]['Name'].count()) print(dataframe[(dataframe['Physics'] > 10) & (dataframe['Chemistry'] > 11) & (dataframe['Math'] > 9)])
Producción:
Publicación traducida automáticamente
Artículo escrito por dpsvnshob130491 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA