Analicemos cómo cambiar las dimensiones de una array. En NumPy , esto se puede lograr de muchas maneras. Analicemos cada uno de ellos.
Método #1: Usar Forma()
Sintaxis:
array_name.shape()
Python3
# importing numpy import numpy as np def main(): # initialising array print('Initialised array') gfg = np.array([1, 2, 3, 4]) print(gfg) # checking current shape print('current shape of the array') print(gfg.shape) # modifying array according to new dimensions print('changing shape to 2,3') gfg.shape = (2, 2) print(gfg) if __name__ == "__main__": main()
Producción:
Initialised array [1 2 3 4] current shape of the array (4,) changing shape to 2,3 [[1 2] [3 4]]
Método #2: Usar reformar()
El parámetro de orden de la función reformar() es avanzado y opcional. La salida difiere cuando usamos C y F debido a la diferencia en la forma en que NumPy cambia el índice de la array resultante. El pedido A hace que NumPy elija el mejor orden posible de C o F según el tamaño disponible en un bloque de memoria.
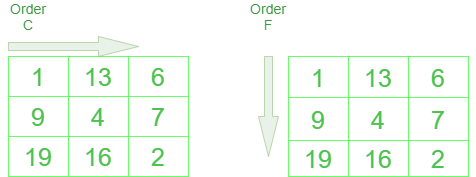
Diferencia entre Orden C y F
Sintaxis:
numpy.reshape(array_name, newshape, order= 'C' or 'F' or 'A')
Python3
# importing numpy import numpy as np def main(): # initialising array gfg = np.arange(1, 10) print('initialised array') print(gfg) # reshaping array into a 3x3 with order C print('3x3 order C array') print(np.reshape(gfg, (3, 3), order='C')) # reshaping array into a 3x3 with order F print('3x3 order F array') print(np.reshape(gfg, (3, 3), order='F')) # reshaping array into a 3x3 with order A print('3x3 order A array') print(np.reshape(gfg, (3, 3), order='A')) if __name__ == "__main__": main()
Producción :
initialised array [1 2 3 4 5 6 7 8 9] 3x3 order C array [[1 2 3] [4 5 6] [7 8 9]] 3x3 order F array [[1 4 7] [2 5 8] [3 6 9]] 3x3 order A array [[1 2 3] [4 5 6] [7 8 9]]
Método #3: Usar redimensionar()
La forma de la array también se puede cambiar usando el método resize(). Si la dimensión especificada es mayor que la array real, los espacios adicionales en la nueva array se llenarán con copias repetidas de la array original.
Sintaxis:
numpy.resize(a, new_shape)
Python3
# importing numpy import numpy as np def main(): # initialise array gfg = np.arange(1, 10) print('initialised array') print(gfg) # resezed array with dimensions in # range of original array np.resize(gfg, (3, 3)) print('3x3 array') print(gfg) # re array with dimensions larger than # original array np.resize(gfg, (4, 4)) # extra spaces will be filled with repeated # copies of original array print('4x4 array') print(gfg) # resize array with dimensions larger than # original array gfg.resize(5, 5) # extra spaces will be filled with zeros print('5x5 array') print(gfg) if __name__ == "__main__": main()
Producción :
initialised array [1 2 3 4 5 6 7 8 9] 3x3 array [1 2 3 4 5 6 7 8 9] 4x4 array [1 2 3 4 5 6 7 8 9] 5x5 array [[1 2 3 4 5] [6 7 8 9 0] [0 0 0 0 0] [0 0 0 0 0] [0 0 0 0 0]]
Publicación traducida automáticamente
Artículo escrito por technikue20 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA