En este artículo, estaríamos discutiendo el objeto Map proporcionado por ES6 . El mapa es una colección de elementos donde cada elemento se almacena como un par clave, valor . El objeto de mapa puede contener objetos y valores primitivos como clave o valor. Cuando iteramos sobre el objeto del mapa, devuelve el par clave, valor en el mismo orden en que se insertó.
Sintaxis:
new Map([it]) Parameter: it - It is any iterable object whose values are stored as key, value pair, If the parameter is not specified then a new map is created is Empty Returns: A new Map object
Ahora vamos a crear un Mapa usando el objeto Mapa
Javascript
// map1 contains // 1 => 2 // 2 => 3 // 4 -> 5 var map1 = new Map([[1 , 2], [2 ,3 ] ,[4, 5]]); console.log("Map1"); console.log(map1); // map2 contains // firstname => sumit // lastname => ghosh // website => geeksforgeeks var map2 = new Map([["firstname" ,"sumit"], ["lastname", "ghosh"], ["website", "geeksforgeeks"]]); console.log("Map2"); console.log(map2); // map3 contains // Whole number => [1, 2, 3, 4] // Decimal number => [1.1, 1.2, 1.3, 1.4] // Negative number => [-1, -2, -3, -4] var map3 = new Map([["whole numbers", [1 ,2 ,3 ,4]], ["Decimal numbers" , [1.1, 1.2, 1.3, 1.4]], ["negative numbers", [-1, -2, -3, -4]]]); console.log("Map3"); console.log(map3); // map 4 contains // storing arrays both as key and value // "first name ", "Last name" => "sumit", "ghosh" // "friend 1", "sourav" => "friend 2", "gourav" var map4 = new Map([[["first name", "last name"], ["sumit", "ghosh"]], [["friend 1", "friend 2"], ["sourav","gourav"]]]); console.log("Map4"); console.log(map4);
Producción:
Propiedades:
Map.prototype.size – Devuelve el número de elementos o los pares clave-valor en el mapa.
Métodos:
1. Map.prototype.set() : agrega la clave y el valor al objeto de mapa.
Sintaxis:
map1.set(k, v); Parameters: k - Key of the element to be added to the Map v - value of the element to be added to the Map Returns: It returns a Map object
2. Map.prototype.has() : devuelve un valor booleano dependiendo de si la clave especificada está presente o no.
Sintaxis:
map1.has(k); Parameters: k - Key of the element to checked Returns: true if the element with the specified key is present or else returns false.
3. Map.prototype.get() – Devuelve el valor de la clave correspondiente .
Sintaxis:
map1.get(k); Parameters: k - Key, whose value is to be returned Returns: The value associated with the key, if it is present in Map, otherwise returns undefined
4. Map.prototype.delete() : elimina tanto la clave como un valor del mapa.
Sintaxis:
map1.delete(k); Parameters: k - Key which is to be deleted from the map Returns: true if the value is found and deleted from the map otherwise, it returns false
5. Map.prototype.clear() : elimina todos los elementos del objeto Map.
Sintaxis:
map1.clear(); Parameters: No parameters Returns: undefined
Usemos todos los métodos descritos anteriormente:
Ejemplo:
Javascript
// Using Map.prototype.set(k, v) // creating an empty map var map1 = new Map(); // adding some elements to the map map1.set("first name", "sumit"); map1.set("last name", "ghosh"); map1.set("website", "geeksforgeeks") .set("friend 1","gourav") .set("friend 2","sourav"); // map1 contains // "first name" => "sumit" // "last name" => "ghosh" // "website" => "geeksforgeeks" // "friend 1" => "gourav" // "friend 2" => "sourav" console.log(map1); // Using Map.prototype.has(k) // returns true console.log("map1 has website ? "+ map1.has("website")); // return false console.log("map1 has friend 3 ? " + map1.has("friend 3")); // Using Map.prototype.get(k) // returns geeksforgeeks console.log("get value for key website "+ map1.get("website")); // returns undefined console.log("get value for key friend 3 "+ map1.get("friend 3")); // Using Map.prototype.delete(k) // removes key "website" and its value from // the map // it prints the value of the key console.log("delete element with key website " + map1.delete("website")); // as the value is deleted from // the map hence it returns false console.log("map1 has website ? "+ map1.has("website")); // returns false as this key is not in the list console.log("delete element with key website " + map1.delete("friend 3")); // Using Map.prototype.clear() // removing all values from map1 map1.clear(); // map1 is empty console.log(map1);
Producción:
6. Map.prototype.entries() : devuelve un objeto iterador que contiene un par clave/valor para cada elemento presente en el objeto Map.
Sintaxis:
map1.entries(); Parameters: No parameters Returns: It returns an iterator object
7. Map.prototype.keys() : devuelve un objeto iterador que contiene todas las claves presentes en el objeto de mapa.
Sintaxis:
map1.keys(); Parameters: No parameter Returns: An iterator object
8. Map.prototype.values() : devuelve un objeto iterador que contiene todos los valores presentes en el objeto de mapa.
Sintaxis:
map1.values(); Parameters: No parameter Returns: An iterator object
Usemos todos los métodos descritos anteriormente:
Ejemplo:
Javascript
// creating an empty map var map1 = new Map(); // adding some elements to the map map1.set("first name", "sumit"); map1.set("last name", "ghosh"); map1.set("website", "geeksforgeeks") .set("friend 1","gourav") .set("friend 2","sourav"); // Using Map.prototype.entries() // getting all the entries of the map var get_entries = map1.entries(); // it prints // ["first name", "sumit"] // ["last name", "ghosh"] // ["website", "geeksforgeeks"] // ["friend 1", "gourav"] // ["friend 2", "sourav"] console.log("----------entries---------"); for(var ele of get_entries) console.log(ele); // Using Map.prototype.keys() // getting all the keys of the map var get_keys = map1.keys(); // it prints // "first name", "last name", // "website", "friend 1", "friend 2" console.log("--------keys----------"); for(var ele of get_keys) console.log(ele); // Using Map.prototype.values() // getting all the values of the map var get_values = map1.values(); // it prints all the values // "sumit", "ghosh", "geeksforgeeks" // "gourav", "sourav" console.log("----------values------------"); for(var ele of get_values) console.log(ele);
Producción:
9. Map.prototype.forEach() : ejecuta la función de devolución de llamada una vez para cada par clave/valor en el mapa, en el orden de inserción.
Sintaxis:
map1.forEach(callback, [thisArgument]); Parameters: callback - It is a function that is to be executed for each element of the Map. thisargument - Value to be used as this when executing the callback. Returns: undefined
La función de devolución de llamada se proporciona con tres parámetros de la siguiente manera:
- la clave del elemento
- el valor del elemento
- el objeto Mapa a recorrer
Ejemplo:
Javascript
// using Map.prototype.forEach() // creating an empty map var map1 = new Map(); // adding some elements to the map map1.set("first name", "sumit"); map1.set("last name", "ghosh"); map1.set("website", "geeksforgeeks") .set("friend 1", "gourav") .set("friend 2", "sourav"); // Declaring a call back function // we are using only one parameter value // so it will ignore other two . function printOne(values) { console.log(values); } // It prints value of all the element // of the set console.log("-----one parameter-----"); map1.forEach(printOne); // Declaring a call back function // we are using two parameter value // so it will ignore last one function printTwo(values, key) { console.log(key + " " + values); } // As key and values are same in set // so it will print values twice console.log("-----two parameter-----"); map1.forEach(printTwo); // Declaring a call back function // we are using all three parameter value function printThree(values, key, map) { // it will print key and value // and the set object console.log(key + " " + values); console.log(map); } // It prints key and value of each // element and the entire Map object console.log("-----three parameter-----"); map1.forEach(printThree);
Producción:
Nota: En el ejemplo anterior, usamos una función de devolución de llamada simple que solo imprime un elemento en la consola, puede diseñarse para realizar cualquier operación compleja según los requisitos.
10. Map.prototype[@@iterator]() : devuelve una función de iterador de mapa que es el método de entradas() del objeto de mapa de forma predeterminada.
Sintaxis:
map1[Symbol.iterator] Parameters: No parameters Returns: Returns an map iterator object and it is entries() by default
Ejemplo:
Javascript
// using Map.prototype[@@iterator]() // creating an empty map var map1 = new Map(); // adding some elements to the map map1.set("first name", "sumit"); map1.set("last name", "ghosh"); map1.set("website", "geeksforgeeks") .set("friend 1", "gourav") .set("friend 2", "sourav"); // By default this method returns the // same iterator object return by entries methods var getit = map1[Symbol.iterator](); // it prints // ["first name", "sumit"] // ["last name", "ghosh"] // ["website", "geeksforgeeks"] // ["friend 1", "gourav"] // ["friend 2", "sourav"] for(var elem of getit) console.log(elem);
Producción:
11. Mapa utilizado para iterar sobre arreglos
Sintaxis:
arr.map(function(value,index){}) Parameters: value = array element index = index of array Return: Modified valueo of elements
Ejemplo:
Javascript
let arr = [1,2,3,4,5] let arr1 = []; //iterate over array and store in arr1 arr1 = arr.map((value,idx)=>{ console.log("value "+value,"idx "+idx); return value*2; }) console.log(); //original array console.log("arr= "+arr); //modified array console.log("arr1= "+arr1);
Producción:
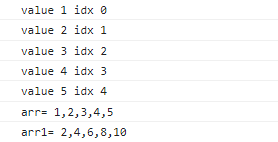
Nota: – Podemos crear una definición de usuario iterable en lugar de usar la predeterminada.
Referencia:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map
JavaScript es mejor conocido por el desarrollo de páginas web, pero también se usa en una variedad de entornos que no son de navegador. Puede aprender JavaScript desde cero siguiendo este tutorial de JavaScript y ejemplos de JavaScript .
Publicación traducida automáticamente
Artículo escrito por GeeksforGeeks-1 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA