En esta publicación, aprenderemos la lógica detrás de los botones de compartir que se muestran en los sitios web que se pueden usar para compartir una publicación o la URL completa del sitio en otras plataformas de redes sociales. Revisaremos esto en varios pasos para comprenderlo.
Paso 1: Primero, necesitamos crear un archivo HTML que muestre todo en el navegador.
html
<!DOCTYPE html> <html> <head> <style type="text/css" media="all"> /* This CSS is optional */ #share-button { padding: 10px; font-size: 24px; } </style> </head> <body> <!-- The share button --> <button id="share-button">Share</button> <!-- These line breaks are optional --> <br/> <br/> <br/> <!-- The anchor tag for the sharing link --> <a href="#"></a> <script type="text/javascript" charset="utf-8"> // We will write the javascript code here </script> </body> </html>
Producción:
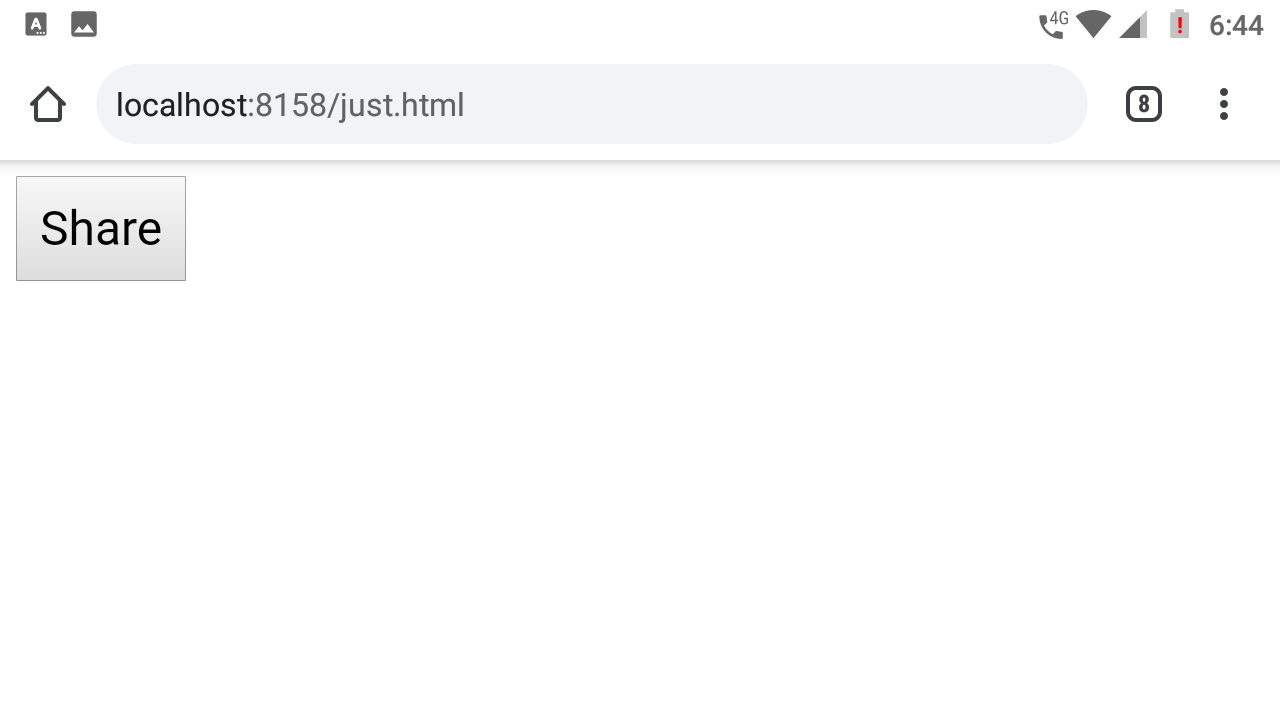
Resultado para el código HTML.
Arriba está el código HTML y vamos a escribir sólo la parte de JavaScript a partir de este punto .
Paso 2: en este paso, agregaremos el código JavaScript que mostrará la URL de la página web actual como un enlace.
- Aquí, la ‘ ventana ‘ es un objeto global que consta de varias propiedades, de las cuales la ubicación es una de esas propiedades y la propiedad href nos proporciona la URL completa con la ruta y el protocolo utilizado.
- Pero no necesitamos el ‘https://’ (protocolo), por lo que lo eliminamos usando el método de división de JavaScript.
HTML
<!DOCTYPE html> <html> <head> <style type="text/css" media="all"> /* This CSS is optional */ #share-button { padding: 10px; font-size: 24px; } </style> </head> <body> <!-- The share button --> <button id="share-button">Share</button> <!-- These line breaks are optional --> <br/> <br/> <br/> <!-- The anchor tag for the sharing link --> <a href="#"></a> <script type="text/javascript" charset="utf-8"> // Make sure you write this code inside the // script tag of your HTML file // Storing the URL of the current webpage const URL = window.location.href.slice(7); // We used the slice method to remove // the 'http://' from the prefix // Displaying the current webpage link // on the browser window const link = document.querySelector('a'); link.textContent = URL; link.href = URL; // Displaying in the console console.log(URL); </script> </body> </html>
Producción:
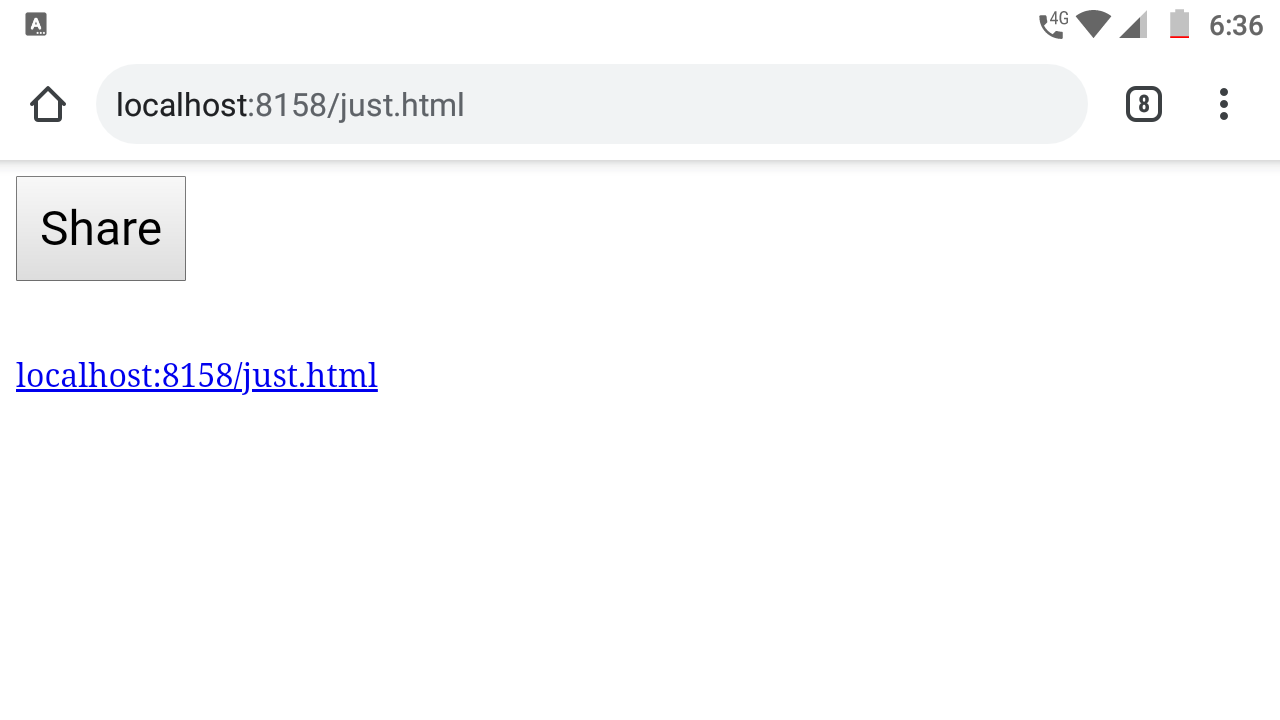
Resultado del Paso: 2
Paso 3: No queremos que se muestre la URL tan pronto como se cargue la ventana del navegador, sino que queremos que se muestre cuando hagamos clic en el botón. Por lo tanto, agregaremos un detector de eventos al botón y luego mostraremos la URL cuando se haga clic en el botón.
HTML
<!DOCTYPE html> <html> <head> <style type="text/css" media="all"> /* This CSS is optional */ #share-button { padding: 10px; font-size: 24px; } </style> </head> <body> <!-- The share button --> <button id="share-button">Share</button> <!-- These line breaks are optional --> <br/> <br/> <br/> <!-- The anchor tag for the sharing link --> <a href="#"></a> <script type="text/javascript" charset="utf-8"> // Make sure you write this code inside the // script tag of your HTML file // Storing the URL of the current webpage const URL = window.location.href.slice(7); // We used the slice method to remove // the 'http://' from the prefix // Displaying the current webpage link // on the browser window const link = document.querySelector('a'); const button = document.querySelector('#share-button'); // Adding a mouse click event listener to the button button.addEventListener('click', () => { // Displaying the current webpage link // on the browser window link.textContent = URL; link.href = URL; // Displaying in the console console.log(URL); }); </script> </body> </html>
Producción:
Paso 4: ahora el enlace se muestra cuando se hace clic en el botón, pero no necesitamos un enlace a la página actual, sino un enlace que comparta la página web actual en una plataforma de redes sociales. Así que actualizamos el código de la siguiente manera.
HTML
<!DOCTYPE html> <html> <head> <style type="text/css" media="all"> /* This CSS is optional */ #share-button { padding: 10px; font-size: 24px; } </style> </head> <body> <!-- The share button --> <button id="share-button">Share</button> <!-- These line breaks are optional --> <br/> <br/> <br/> <!-- The anchor tag for the sharing link --> <a href="#"></a> <script type="text/javascript" charset="utf-8"> // Make sure you write this code inside the // script tag of your HTML file // Facebook share url const fbShare = "https://www.facebook.com/sharer/sharer.php?u=" // Storing the URL of the current webpage const URL = window.location.href.slice(7); // We used the slice method to remove // the 'http://' from the prefix // Displaying the current webpage link // on the browser window const link = document.querySelector('a'); const button = document.querySelector('#share-button'); // Adding a mouse click event listener to the button button.addEventListener('click', () => { // Displaying the current webpage link // on the browser window link.textContent = fbShare+URL; link.href = fbShare+URL; // Displaying in the console console.log(fbShare+URL); }); </script> </body> </html>
Producción:
Así es como podemos obtener una URL para compartir, en lugar de mostrar la URL para compartir, podemos usarla dentro del botón para redirigir directamente al usuario a la plataforma de redes sociales en particular. Además, he usado la URL para compartir de Facebook y hay URL similares disponibles para casi todas las plataformas de redes sociales y siéntase libre de experimentar con ellas y crear su propio botón para compartir. Esta publicación ha cubierto todos los aspectos esenciales sobre cómo compartir la URL de la página web actual usando JavaScript.
Publicación traducida automáticamente
Artículo escrito por haridarshanc y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA