La interfaz set está presente en el paquete java.util y amplía la interfaz Collection, que es una colección desordenada de objetos en los que no se pueden almacenar valores duplicados. Es una interfaz que implementa el conjunto matemático. Esta interfaz contiene los métodos heredados de la interfaz Collection y agrega una función que restringe la inserción de elementos duplicados. Hay dos interfaces que amplían la implementación del conjunto, a saber, SortedSet y NavigableSet .
Java
// Java program Illustrating Set Interface // Importing utility classes import java.util.*; // Main class public class GFG { // Main driver method public static void main(String[] args) { // Demonstrating Set using HashSet // Declaring object of type String Set<String> hash_Set = new HashSet<String>(); // Adding elements to the Set // using add() method hash_Set.add("Geeks"); hash_Set.add("For"); hash_Set.add("Geeks"); hash_Set.add("Example"); hash_Set.add("Set"); // Printing elements of HashSet object System.out.println(hash_Set); } }
Java
// Java Program Demonstrating Operations on the Set // such as Union, Intersection and Difference operations // Importing all utility classes import java.util.*; // Main class public class SetExample { // Main driver method public static void main(String args[]) { // Creating an object of Set class // Declaring object of Integer type Set<Integer> a = new HashSet<Integer>(); // Adding all elements to List a.addAll(Arrays.asList( new Integer[] { 1, 3, 2, 4, 8, 9, 0 })); // Again declaring object of Set class // with reference to HashSet Set<Integer> b = new HashSet<Integer>(); b.addAll(Arrays.asList( new Integer[] { 1, 3, 7, 5, 4, 0, 7, 5 })); // To find union Set<Integer> union = new HashSet<Integer>(a); union.addAll(b); System.out.print("Union of the two Set"); System.out.println(union); // To find intersection Set<Integer> intersection = new HashSet<Integer>(a); intersection.retainAll(b); System.out.print("Intersection of the two Set"); System.out.println(intersection); // To find the symmetric difference Set<Integer> difference = new HashSet<Integer>(a); difference.removeAll(b); System.out.print("Difference of the two Set"); System.out.println(difference); } }
Union of the two Set[0, 1, 2, 3, 4, 5, 7, 8, 9] Intersection of the two Set[0, 1, 3, 4] Difference of the two Set[2, 8, 9]
Realización de varias operaciones en SortedSet
Después de la introducción de Generics en Java 1.5, es posible restringir el tipo de objeto que se puede almacenar en el Conjunto. Dado que Set es una interfaz, solo se puede usar con una clase que implemente esta interfaz. HashSet es una de las clases más utilizadas que implementa la interfaz Set. Ahora, veamos cómo realizar algunas operaciones de uso frecuente en HashSet. Vamos a realizar las siguientes operaciones de la siguiente manera:
- Agregar elementos
- Acceder a elementos
- Quitar elementos
- Elementos de iteración
- Iterando a través de Set
Ahora analicemos estas operaciones individualmente de la siguiente manera:
Operaciones 1: Adición de elementos
Para agregar un elemento al Conjunto, podemos usar el método add() . Sin embargo, el orden de inserción no se conserva en el Conjunto. Internamente, para cada elemento, se genera un hash y se almacenan los valores con respecto al hash generado. los valores se comparan y clasifican en orden ascendente. Debemos tener en cuenta que los elementos duplicados no están permitidos y todos los elementos duplicados se ignoran. Y también, los valores nulos son aceptados por el conjunto.
Ejemplo
Java
// Java Program Demonstrating Working of Set by // Adding elements using add() method // Importing all utility classes import java.util.*; // Main class class GFG { // Main driver method public static void main(String[] args) { // Creating an object of Set and // declaring object of type String Set<String> hs = new HashSet<String>(); // Adding elements to above object // using add() method hs.add("B"); hs.add("B"); hs.add("C"); hs.add("A"); // Printing the elements inside the Set object System.out.println(hs); } }
[A, B, C]
Operación 2: Acceso a los Elementos
Después de agregar los elementos, si deseamos acceder a los elementos, podemos usar métodos incorporados como contains() .
Ejemplo
Java
// Java code to demonstrate Working of Set by // Accessing the Elements of the Set object // Importing all utility classes import java.util.*; // Main class class GFG { // Main driver method public static void main(String[] args) { // Creating an object of Set and // declaring object of type String Set<String> hs = new HashSet<String>(); // Elements are added using add() method // Later onwards we will show accessing the same // Custom input elements hs.add("A"); hs.add("B"); hs.add("C"); hs.add("A"); // Print the Set object elements System.out.println("Set is " + hs); // Declaring a string String check = "D"; // Check if the above string exists in // the SortedSet or not // using contains() method System.out.println("Contains " + check + " " + hs.contains(check)); } }
Set is [A, B, C] Contains D false
Operación 3: Eliminación de los valores
Los valores se pueden eliminar del Conjunto mediante el método remove() .
Ejemplo
Java
// Java Program Demonstrating Working of Set by // Removing Element/s from the Set // Importing all utility classes import java.util.*; // Main class class GFG { // Main driver method public static void main(String[] args) { // Declaring object of Set of type String Set<String> hs = new HashSet<String>(); // Elements are added // using add() method // Custom input elements hs.add("A"); hs.add("B"); hs.add("C"); hs.add("B"); hs.add("D"); hs.add("E"); // Printing initial Set elements System.out.println("Initial HashSet " + hs); // Removing custom element // using remove() method hs.remove("B"); // Printing Set elements after removing an element // and printing updated Set elements System.out.println("After removing element " + hs); } }
Initial HashSet [A, B, C, D, E] After removing element [A, C, D, E]
Operación 4: Iterando a través del Conjunto
Hay varias formas de iterar a través del Conjunto. El más famoso es usar el bucle for mejorado.
Ejemplo
Java
// Java Program to Demonstrate Working of Set by // Iterating through the Elements // Importing utility classes import java.util.*; // Main class class GFG { // Main driver method public static void main(String[] args) { // Creating object of Set and declaring String type Set<String> hs = new HashSet<String>(); // Adding elements to Set // using add() method // Custom input elements hs.add("A"); hs.add("B"); hs.add("C"); hs.add("B"); hs.add("D"); hs.add("E"); // Iterating through the Set // via for-each loop for (String value : hs) // Printing all the values inside the object System.out.print(value + ", "); System.out.println(); } }
A, B, C, D, E,
Las clases que implementan la interfaz Set en Java Collections se pueden percibir fácilmente en la siguiente imagen y se enumeran de la siguiente manera:
- HashSet
- EnumSet
- LinkedHashSet
- ÁrbolConjunto
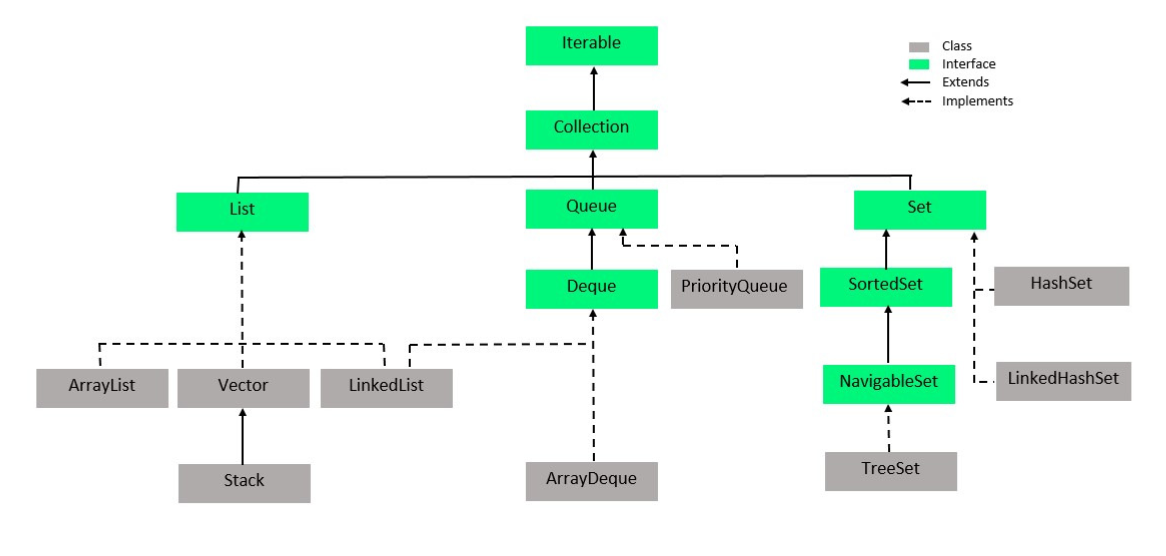
Clase 1: HashSet
La clase HashSet que se implementa en el marco de la colección es una implementación inherente de la estructura de datos de la tabla hash . Los objetos que insertamos en el HashSet no garantizan que se inserten en el mismo orden. Los objetos se insertan en función de su código hash. Esta clase también permite la inserción de elementos NULL. Veamos cómo crear un objeto de conjunto usando esta clase.
Ejemplo
Java
// Java program Demonstrating Creation of Set object // Using the Hashset class // Importing utility classes import java.util.*; // Main class class GFG { // Main driver method public static void main(String[] args) { // Creating object of Set of type String Set<String> h = new HashSet<String>(); // Adding elements into the HashSet // using add() method // Custom input elements h.add("India"); h.add("Australia"); h.add("South Africa"); // Adding the duplicate element h.add("India"); // Displaying the HashSet System.out.println(h); // Removing items from HashSet // using remove() method h.remove("Australia"); System.out.println("Set after removing " + "Australia:" + h); // Iterating over hash set items System.out.println("Iterating over set:"); // Iterating through iterators Iterator<String> i = h.iterator(); // It holds true till there is a single element // remaining in the object while (i.hasNext()) System.out.println(i.next()); } }
[South Africa, Australia, India] Set after removing Australia:[South Africa, India] Iterating over set: South Africa India
Clase 2: EnumSet
La clase EnumSet que se implementa en el marco de las colecciones es una de las implementaciones especializadas de la interfaz Set para usar con el tipo de enumeración . Es una implementación de conjuntos de alto rendimiento, mucho más rápida que HashSet. Todos los elementos de un conjunto de enumeración deben provenir de un único tipo de enumeración que se especifica cuando el conjunto se crea de forma explícita o implícita. Veamos cómo crear un objeto de conjunto usando esta clase.
Ejemplo
Java
// Java program to demonstrate the // creation of the set object // using the EnumSet class import java.util.*; enum Gfg { CODE, LEARN, CONTRIBUTE, QUIZ, MCQ } ; public class GFG { public static void main(String[] args) { // Creating a set Set<Gfg> set1; // Adding the elements set1 = EnumSet.of(Gfg.QUIZ, Gfg.CONTRIBUTE, Gfg.LEARN, Gfg.CODE); System.out.println("Set 1: " + set1); } }
Set 1: [CODE, LEARN, CONTRIBUTE, QUIZ]
Clase 3: LinkedHashSet
La clase LinkedHashSet que se implementa en el marco de las colecciones es una versión ordenada de HashSet que mantiene una lista doblemente vinculada en todos los elementos. Cuando se necesita mantener el orden de iteración, se utiliza esta clase. Al iterar a través de un HashSet, el orden es impredecible, mientras que un LinkedHashSet nos permite iterar a través de los elementos en el orden en que se insertaron. Veamos cómo crear un objeto de conjunto usando esta clase.
Ejemplo
Java
// Java program to demonstrate the // creation of Set object using // the LinkedHashset class import java.util.*; class GFG { public static void main(String[] args) { Set<String> lh = new LinkedHashSet<String>(); // Adding elements into the LinkedHashSet // using add() lh.add("India"); lh.add("Australia"); lh.add("South Africa"); // Adding the duplicate // element lh.add("India"); // Displaying the LinkedHashSet System.out.println(lh); // Removing items from LinkedHashSet // using remove() lh.remove("Australia"); System.out.println("Set after removing " + "Australia:" + lh); // Iterating over linked hash set items System.out.println("Iterating over set:"); Iterator<String> i = lh.iterator(); while (i.hasNext()) System.out.println(i.next()); } }
[India, Australia, South Africa] Set after removing Australia:[India, South Africa] Iterating over set: India South Africa
Clase 4: Conjunto de árboles
La clase TreeSet que se implementa en el marco de las colecciones y la implementación de la interfaz SortedSet y SortedSet amplía la interfaz Set. Se comporta como un conjunto simple con la excepción de que almacena elementos en un formato ordenado. TreeSet utiliza una estructura de datos de árbol para el almacenamiento. Los objetos se almacenan en orden ascendente ordenado. Pero podemos iterar en orden descendente usando el método TreeSet.descendingIterator(). Veamos cómo crear un objeto de conjunto usando esta clase.
Ejemplo
Java
// Java Program Demonstrating Creation of Set object // Using the TreeSet class // Importing utility classes import java.util.*; // Main class class GFG { // Main driver method public static void main(String[] args) { // Creating a Set object and declaring it of String // type // with reference to TreeSet Set<String> ts = new TreeSet<String>(); // Adding elements into the TreeSet // using add() ts.add("India"); ts.add("Australia"); ts.add("South Africa"); // Adding the duplicate // element ts.add("India"); // Displaying the TreeSet System.out.println(ts); // Removing items from TreeSet // using remove() ts.remove("Australia"); System.out.println("Set after removing " + "Australia:" + ts); // Iterating over Tree set items System.out.println("Iterating over set:"); Iterator<String> i = ts.iterator(); while (i.hasNext()) System.out.println(i.next()); } }
[Australia, India, South Africa] Set after removing Australia:[India, South Africa] Iterating over set: India South Africa
Publicación traducida automáticamente
Artículo escrito por GeeksforGeeks-1 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA