La animación de explosión se puede crear usando lienzo en HTML.
Enfoque: Al principio, tenemos que crear un elemento de lienzo en HTML y obtener su referencia en JavaScript. Todos los elementos y animaciones se controlan mediante funciones de JavaScript. Algunos pasos requeridos son:
- Utilice los métodos de lienzo para obtener el lienzo y el contexto.
- Decida el color, el patrón y el degradado del texto y el objeto de partículas.
- Decida y dibuje la forma para la animación o la representación de fotogramas
- Limpia el lienzo anterior.
- Guarde el lienzo después de las transformaciones para el estado original
- Restaure el lienzo antes de dibujar un nuevo marco
. Podemos crear muchas partículas y animarlas en direcciones aleatorias que se desvanecen después de un tiempo.
Código HTML:
HTML
<!DOCTYPE html> <html> <head> <style> * { padding: 0; margin: 0; box-sizing: border-box; ; } body { overflow: hidden; } </style> </head> <body> <canvas id="myCanvas"></canvas> <script> /* Get the canvas */ var canvas = document.getElementById("myCanvas"); /* Get the height and width of the window */ canvas.height = window.innerHeight; canvas.width = window.innerWidth; /* Get the 2D context of the canvas */ var ctx = canvas.getContext("2d"); /* Fills or sets the color,gradient,pattern */ ctx.fillStyle = "white"; ctx.fillRect(0, 0, canvas.width, canvas.height); ctx.font = "50px Arial"; ctx.fillStyle = "green"; /* Writes the required text */ ctx.fillText("GFG", 500, 350) let particles = []; /* Initialize particle object */ class Particle { constructor(x, y, radius, dx, dy) { this.x = x; this.y = y; this.radius = radius; this.dx = dx; this.dy = dy; this.alpha = 1; } draw() { ctx.save(); ctx.globalAlpha = this.alpha; ctx.fillStyle = 'green'; /* Begins or reset the path for the arc created */ ctx.beginPath(); /* Some curve is created*/ ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false); ctx.fill(); /* Restore the recent canvas context*/ ctx.restore(); } update() { this.draw(); this.alpha -= 0.01; this.x += this.dx; this.y += this.dy; } } /* Timer is set for particle push execution in intervals*/ setTimeout(() => { for (i = 0; i <= 150; i++) { let dx = (Math.random() - 0.5) * (Math.random() * 6); let dy = (Math.random() - 0.5) * (Math.random() * 6); let radius = Math.random() * 3; let particle = new Particle(575, 375, radius, dx, dy); /* Adds new items like particle*/ particles.push(particle); } explode(); }, 3000); /* Particle explosion function */ function explode() { /* Clears the given pixels in the rectangle */ ctx.clearRect(0, 0, canvas.width, canvas.height); ctx.fillStyle = "white"; ctx.fillRect(0, 0, canvas.width, canvas.height); particles.forEach((particle, i) => { if (particle.alpha <= 0) { particles.splice(i, 1); } else particle.update() }) /* Performs a animation after request*/ requestAnimationFrame(explode); } </script> </body> </html>
Producción:
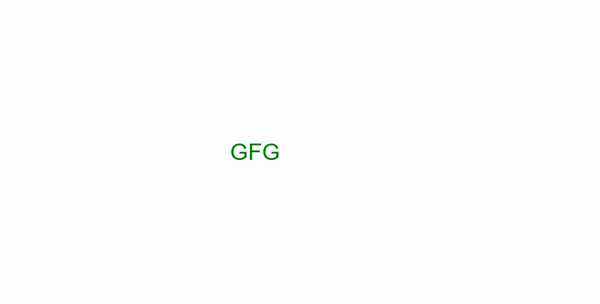
Animación de explosión
Publicación traducida automáticamente
Artículo escrito por vivshubham y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA