Prerrequisito : Programación de sockets en Java
Primero, entendemos los conceptos básicos de la programación de sockets Java. Java Socket se utiliza para comunicarse entre dos JRE diferentes. El socket de Java puede estar orientado a la conexión o sin conexión. En java, tenemos un paquete llamado “java.net”. En este paquete, tenemos dos clases, clase de socket y clase de servidor. Esas clases se utilizan para crear programas orientados a la conexión o sin conexión. En este artículo, veremos cómo el servidor Java realizará las operaciones básicas y devolverá el resultado al cliente Java.
Cliente Java
Primero, creamos y escribimos código de socket del lado del cliente. En el programa de socket del cliente debe saber dos información
- Dirección IP del servidor y,
- Número de puerto
Java
// A Java program for a Client import java.io.*; import java.net.*; import java.util.*; public class Client { private Socket s = null; public Client(String address, int port) { try { // In this Code Input Getting from a user Scanner sc = new Scanner(System.in); s = new Socket(address, port); System.out.println("Connected"); // Create two objects first is dis and dos for // input and output DataInputStream dis = new DataInputStream(s.getInputStream()); DataOutputStream dos = new DataOutputStream(s.getOutputStream()); // Making a Loop while (true) { System.out.println( "Enter the operation in the form operand operator operand"); System.out.println("Example 3 + 5 "); String inp = sc.nextLine(); // Check the user input if user enter over // then // connect is stopped by server and user if (inp.equals("Over")) break; dos.writeUTF(inp); String ans = dis.readUTF(); System.out.println("Answer = " + ans); } } catch (Exception e) { System.out.println("Error in Connection"); } } public static void main(String args[]) { // Connection With Server port 5000 Client client = new Client("127.0.0.1", 5000); } }
Servidor Java
En este servidor Java, estamos realizando cálculos simples, como sumas, restas, etc. Primero en este código, recibimos información del lado del cliente. Pasamos esta información a la «clase Stringtokenizer», esta clase ayuda a extraer el «operando y la operación» exactos para realizar la operación. cuando el resultado está listo, el servidor Java lo envía de vuelta al cliente de resultados
Java
// A Java program for a Server import java.io.*; import java.net.*; import java.util.*; public class Server { // initialize socket and input stream private Socket socket = null; // constructor with port public Server(int port) { try { // Making a ServerSocket object // for receiving Client Request ServerSocket ss = new ServerSocket(port); Socket s = ss.accept(); // dis and dos object for receiving // input from client send output to client DataInputStream dis = new DataInputStream(s.getInputStream()); DataOutputStream dos = new DataOutputStream(s.getOutputStream()); while (true) { String input = dis.readUTF(); if (input.equals("bye")) break; System.out.println("Equation Received"); int result = 0; StringTokenizer st = new StringTokenizer(input); int oprnd1 = Integer.parseInt(st.nextToken()); String operation = st.nextToken(); int oprnd2 = Integer.parseInt(st.nextToken()); // Calculator Operation Perform By Server if (operation.equals("+")) { result = oprnd1 + oprnd2; } else if (operation.equals("-")) { result = oprnd1 - oprnd2; } else if (operation.equals("/")) { result = oprnd1 / oprnd2; } else if (operation.equals("*")) { result = oprnd1 * oprnd2; } System.out.println("Sending the Result"); dos.writeUTF(Integer.toString(result)); } } catch (Exception e) { System.out.println("Error"); } } public static void main(String args[]) { // Server Object and set port number 5000 Server server = new Server(5000); } }
Producción:
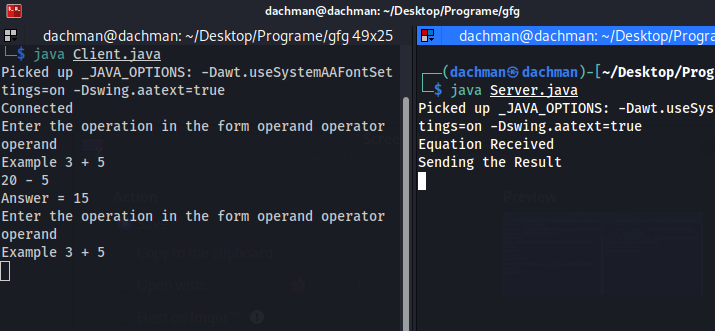
Publicación traducida automáticamente
Artículo escrito por neeraj3304 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA