Podemos agregar un texto de búsqueda predeterminado al cuadro de búsqueda mediante el método JavaScript DOM getElementById() y los eventos onfocus y onblur .
- getElementById(): el método DOM getElementById() se utiliza para devolver valores a la identificación única de la etiqueta de entrada utilizada en HTML. Es uno de los métodos DOM HTML más utilizados cuando queremos manipular y obtener información de su documento. Supongamos que si existe más de un elemento con la misma identificación, volverá al primer elemento del código. Si la identificación especificada no existe en el código, devolverá un valor nulo.
Sintaxis:
document.getElementById('element_id');
- evento onfocus: el evento onfocus se usa principalmente con las etiquetas de entrada, selección y anclaje, cada vez que el usuario hace clic en las etiquetas de entrada, el evento onfocus entra en juego. Se usa principalmente junto con el evento onblur.
Sintaxis:
<element onfocus = "script">
- Evento onblur: el evento onblur se usa junto con el evento onfocus. Entra en juego cuando el elemento pierde el foco, también se usa con las etiquetas de entrada, selección y anclaje.
Sintaxis:
<element onblur = "script">
Aquí hemos usado dos ejemplos para agregar texto predeterminado a un cuadro de búsqueda, el primer ejemplo usa los eventos onfocus y onblur y el segundo ejemplo usa los eventos onfocus y onblur junto con el atributo de marcador de posición.
Ejemplo 1: en el siguiente código, hemos utilizado los atributos de evento onfocus y onblur de JavaScript para agregar un valor predeterminado al cuadro de búsqueda en HTML.
HTML
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Search box with default text</title> </head> <body> <h1 style="color:green;"> GeeksforGeeks </h1> <p>Search word in HTML using JavaScript</p> <form> <input type='text' name='Search' id='Search' /> <input type='submit' name='submit' value='Search' /> </form> <script> // Text to be displayed on search box by default const defaultTextValue = 'Search...'; // Here input id should match with the // parameter of getElementById let searchBox = document.getElementById('Search'); // Default text to be displayed searchBox.value = defaultTextValue; // Search box on focus searchBox.onfocus = function () { if (this.value == defaultTextValue) { // Clears the search box this.value = ''; } } // Search box when clicked outside searchBox.onblur = function () { if (this.value == '') { // Restores the search box with default value this.value = defaultTextValue; } } </script> </body> </html>
Producción:
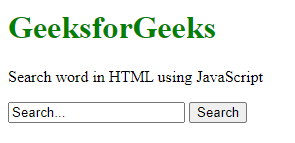
Caja de búsqeda
Ejemplo 2: cuando hace clic en el cuadro de búsqueda, el marcador de posición mostrará el texto «Buscar …» utilizando los eventos onfocus y onblur de JavaScript , podemos agregar el texto de búsqueda predeterminado.
HTML
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Search box with default text</title> </head> <body> <h1 style="color:green;"> GeeksforGeeks </h1> <p>Search word in HTML using JavaScript</p> <form> <input type='text' name='Search' id='Search' placeholder='Search...' /> <input type="submit" name='submit' value='Search' /> </form> <script> // Text to be displayed on search box by default const defaultTextValue = 'Geek'; // Here input id should match with the //parameter of getElementById let searchBox = document.getElementById('Search'); // Default text to be displayed searchBox.value = defaultTextValue; // Search box on focus searchBox.onfocus = function () { if (this.value == defaultTextValue) { // Clears the search box this.value = ''; } } // Search box when clicked outside searchBox.onblur = function () { if (this.value == '') { // Restores the search box with default value this.value = defaultTextValue; } } </script> </body> </html>
Producción:
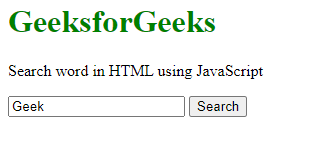
Caja de búsqeda
Publicación traducida automáticamente
Artículo escrito por subodhray5221 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA