La creación de un elemento <div> con una etiqueta de estilo usando jQuery se puede realizar en los siguientes pasos.
Pasos:
- Cree un nuevo elemento <div> .
- Crear un objeto con todos los estilos que queramos aplicar.
- Elija un elemento principal, donde colocar este elemento recién creado.
- Coloque el elemento div creado en el elemento principal.
Ejemplo 1: este ejemplo creará un elemento <div> y usará el método append() para agregar el elemento al final del elemento principal.
HTML
<!DOCTYPE html> <html> <head> <script src="https://code.jquery.com/jquery-git.js"> </script> <style> .divClass { height: 40px; width: 200px; border: 1px solid blue; color: white } </style> </head> <body> <h2 style="color:green">GeeksforGeeks</h2> <div id="parent"> This is parent div </div> <div style="height:10px;"></div> <!-- JavaScript function addText() is called to add to parent div--> <input type="button" value="Add <div> Text" onclick="addText()" /> <script> <!-- Function to add text in a div element with above styles--> function addText() { $(document).ready(function() { var object = { id: "divID", class: "divClass", css: { "color": "Red" } }; var addition = $("<div>", object); addition.html("Added text GFG"); $("#parent").append(addition); }); } </script> </body> </html>
Producción:
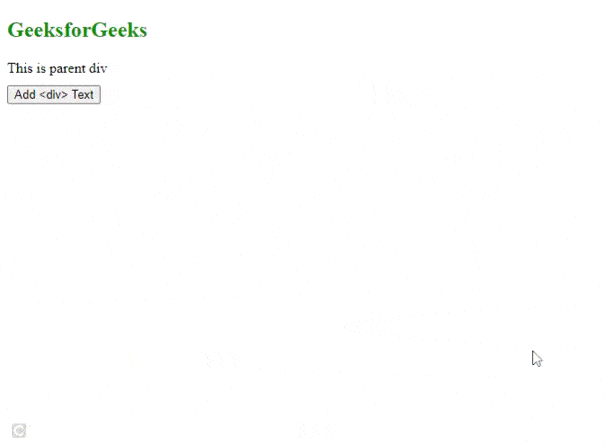
adjuntar
Ejemplo 2: este ejemplo creará un elemento <div> y usa el método prependTo() para agregar el elemento al comienzo del elemento principal.
HTML
<!DOCTYPE html> <html> <head> <script src="https://code.jquery.com/jquery-git.js"> </script> <style> .divClass { height: 40px; width: 200px; border: 1px solid blue; color: white } </style> </head> <body> <h2 style="color:green">GeeksforGeeks</h2> <div style="height:10px;"></div> <div id='parent'> This is parent div </div> <div style="height:10px;"></div> <input type="button" value="Prepend text div " onclick="prependDiv()" /> <script> function prependDiv() { $(document).ready(function() { var object = { id: "divID", class: "divClass", css: { "color": "Red" } }; <!--Prepend object is created before the parent div--> var prependObject = $("<div>", object); prependObject.html("Prepend text is GFG"); $(prependObject).prependTo($('#parent')); }); } </script> </body> </html>
Producción:
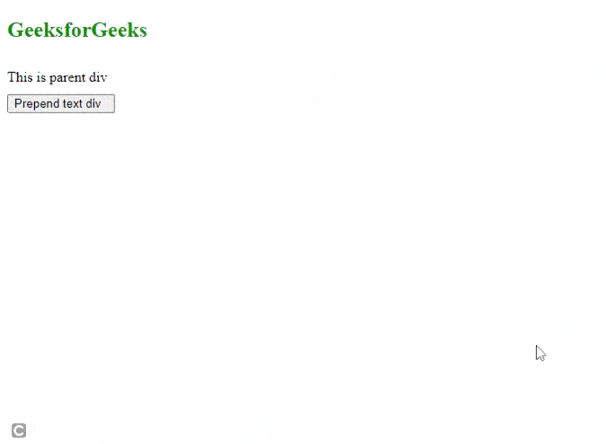
Anteponer texto
Publicación traducida automáticamente
Artículo escrito por KapilChhipa y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA