Requisitos previos: Matplotlib
Dada una imagen, la tarea aquí es redactar un programa de python usando matplotlib para dibujar un rectángulo en él. Matplotlib es útil con la función de rectángulo() que se puede usar para nuestro requisito.
Sintaxis: Rectángulo(xy, ancho, alto, ángulo=0.0, **kwargs)
Parámetros:
- xy: punto inferior izquierdo para iniciar el trazado del rectángulo
- ancho : ancho del rectángulo
- altura: Altura del rectángulo.
- ángulo: Ángulo de rotación del rectángulo.
Acercarse
- Importe las bibliotecas necesarias.
- Insertar y mostrar la imagen.
- Crear la figura y los ejes de la trama.
- Añadir el parche a los Ejes
- Mostrar la imagen
Ejemplo 1: dibujar un rectángulo en una imagen
Python3
import matplotlib.pyplot as plt import matplotlib.patches as patches from PIL import Image import numpy as np x = np.array(Image.open('geek.png'), dtype=np.uint8) plt.imshow(x) # Create figure and axes fig, ax = plt.subplots(1) # Display the image ax.imshow(x) # Create a Rectangle patch rect = patches.Rectangle((50, 100), 40, 30, linewidth=1, edgecolor='r', facecolor="none") # Add the patch to the Axes ax.add_patch(rect) plt.show()
Producción:
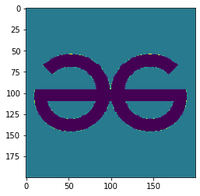
imagen original
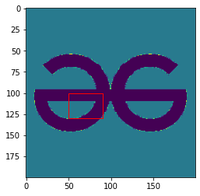
imagen con rectángulo
Ejemplo 2: dibujar un rectángulo relleno
Python3
import matplotlib.pyplot as plt import matplotlib.patches as patches from PIL import Image import numpy as np x = np.array(Image.open('geek.png'), dtype=np.uint8) plt.imshow(x) # Create figure and axes fig, ax = plt.subplots(1) # Display the image ax.imshow(x) # Create a Rectangle patch rect = patches.Rectangle((50, 100), 40, 30, linewidth=1, edgecolor='r', facecolor="g") # Add the patch to the Axes ax.add_patch(rect) plt.show()
Producción:
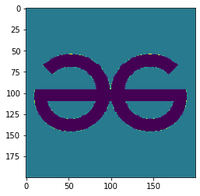
imagen original
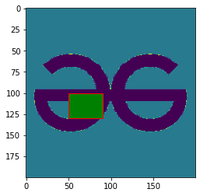
imagen con rectángulo
Publicación traducida automáticamente
Artículo escrito por yashisrivastav y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA