En este artículo, vamos a utilizar la API de geolocalización de Promisify en la API basada en Promise.
Requisito previo: Promesa de JavaScript
Enfoque: como sabemos, navigator.geolocation.getCurrentPosition es una API basada en devolución de llamada, por lo que podemos convertirla fácilmente en una API basada en Promise. Para prometer la API de geolocalización, obtendremos la posición actual del usuario desde el navegador. Resolveremos la promesa o la rechazaremos en función de si obtenemos la posición actual.
Ejemplo:
HTML
<!DOCTYPE html> <html> <head> <meta charset="UTF-8" /> <style> body { text-align: center; } h1 { color: green; } h5 { color: black; } #geeks { font-size: 16px; font-weight: bold; } #gfg { color: green; font-size: 20px; font-weight: bold; } </style> </head> <body> <h1>GeeksforGeeks</h1> <p id="geeks"></p> <button onClick="getLocation()">Get My Location</button> <p id="gfg"></p> <script> let s = `Promisifying the Geo Location API`; document.getElementById("geeks").innerHTML = ` <p>${s}</p> `; // Logic start here let getLocationPromise = () => { return new Promise(function (resolve, reject) { // Promisifying the geolocation API navigator.geolocation.getCurrentPosition( (position) => resolve(position), (error) => reject(error) ); }); }; function getLocation() { getLocationPromise() .then((res) => { // If promise get resolved const { coords } = res; document.getElementById("gfg").innerHTML = ` <p> <strong>You are Located at :</strong> </p> <h5>latitude : ${coords.latitude}</h5> <h5>longitude : ${coords.longitude}</h5> `; }) .catch((error) => { // If promise get rejected document.getElementById("gfg") .innerHTML = ` <p>${error}</p> `; }); } </script> </body> </html>
Producción:
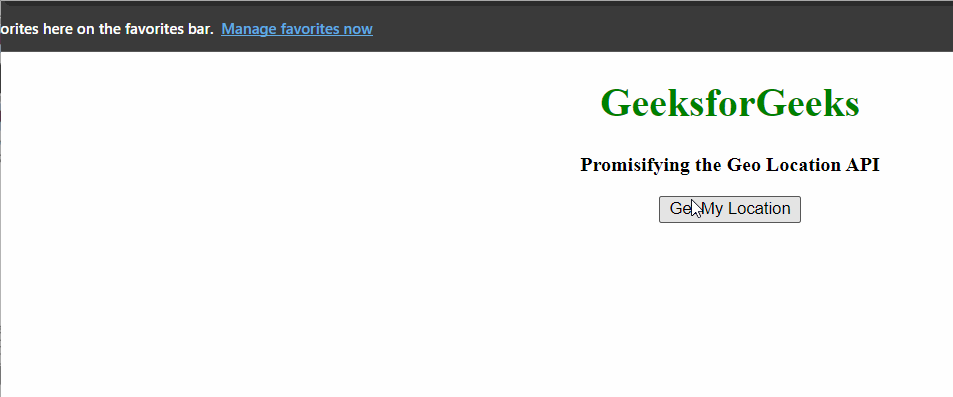
Promete la API de geolocalización
Ejemplo 2: Incluso podemos simplificar el código anterior, para hacerlo mejor. Como sabemos, navigator.geolocation.getCurrentPosition(devolución de llamada, error) llama automáticamente a la función de devolución de llamada y pasa la posición.
HTML
<!DOCTYPE html> <html> <head> <meta charset="UTF-8" /> <style> body { text-align: center; } h1 { color: green; } h5 { color: black; } #geeks { font-size: 16px; font-weight: bold; } #gfg { color: green; font-size: 20px; font-weight: bold; } </style> </head> <body> <h1>GeeksforGeeks</h1> <p id="geeks"></p> <button onClick="getLocation()">Get My Location</button> <p id="gfg"></p> <script> let s = `Promisifying the Geo Location API`; document.getElementById("geeks") .innerHTML = ` <p>${s}</p> `; let getLocationPromise = () => { return new Promise(function (resolve, reject) { // Automatically passes the position // to the callback navigator.geolocation .getCurrentPosition(resolve, reject); }); }; function getLocation() { getLocationPromise() .then((res) => { // If promise get resolved const { coords } = res; document.getElementById("gfg").innerHTML = ` <p> <strong>You are Located at :</strong> </p> <h5>latitude : ${coords.latitude}</h5> <h5>longitude : ${coords.longitude}</h5> `; }) .catch((error) => { // If promise get rejected document.getElementById("gfg").innerHTML = ` <p>${error}</p> `; // Console.error(error); }); } </script> </body> </html>
Producción:
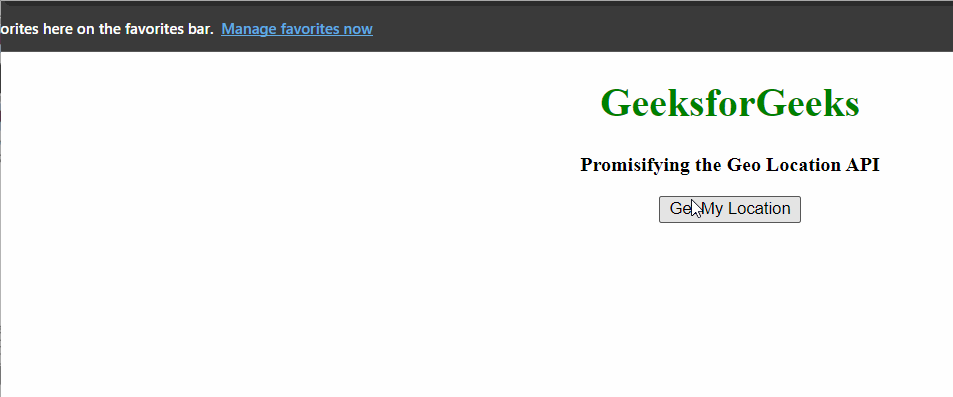
Promete la API de geolocalización