En este artículo, discutiremos cómo crear un botón Activar/Desactivar usando PHP. Cuando se establece un estado en particular, el mismo se actualiza en la base de datos.
Enfoque: para ilustrar el ejemplo, digamos que hay algunos cursos que se pueden configurar como activos o inactivos y deben tener botones de colores apropiados para el mismo. La implementación se realiza mediante HTML, PHP y CSS.
Requisitos:
- Base de datos MySQL con una tabla que contiene al menos el nombre del curso y un valor de estado booleano.
- Archivos PHP y HTML.
- XAMPP (o cualquier alternativa)
Creación de base de datos:
- Abra el panel de control de XAMPP e inicie los módulos de Apache y MySQL.
haga clic en los botones de inicio
- Abra “localhost/phpmyadmin” en su navegador y haga clic en el botón nuevo para crear una nueva base de datos.
- Asigne un nombre a su base de datos y haga clic en crear.
- En la pestaña SQL, ingrese el siguiente código SQL y haga clic en Ir.
Para lograr el resultado deseado, creemos una base de datos llamada «cursos» y configuremos algunos cursos usando el siguiente código SQL.
Table structure for table `courses` Course_name String and status boolean fields CREATE TABLE `courses` ( `Course_name` varchar(50) NOT NULL, `status` tinyint(1) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4; Sample data for table `courses` INSERT INTO `courses` (`Course_name`, `status`) VALUES ('C++', 0), ('Java', 1), ('Data Structures', 1), ('SQL', 0); ALTER TABLE `courses` ADD `id` INT NOT NULL AUTO_INCREMENT FIRST, ADD PRIMARY KEY (`id`);
En esta tabla, debemos saber que el estado es un número entero que representa inactivo como 0 y activo como 1.
Creación de página web: cree una carpeta en htdocs llamada cursos y almacene el siguiente archivo como «course-page.php «.
course-page.php
<?php // Connect to database $con = mysqli_connect("localhost","root","","courses"); // Get all the courses from courses table // execute the query // Store the result $sql = "SELECT * FROM `courses`"; $Sql_query = mysqli_query($con,$sql); $All_courses = mysqli_fetch_all($Sql_query,MYSQLI_ASSOC); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <!-- Using internal/embedded css --> <style> .btn{ background-color: red; border: none; color: white; padding: 5px 5px; text-align: center; text-decoration: none; display: inline-block; font-size: 20px; margin: 4px 2px; cursor: pointer; border-radius: 20px; } .green{ background-color: #199319; } .red{ background-color: red; } table,th{ border-style : solid; border-width : 1; text-align :center; } td{ text-align :center; } </style> </head> <body> <h2>Courses Table</h2> <table> <!-- TABLE TOP ROW HEADINGS--> <tr> <th>Course Name</th> <th>Course Status</th> <th>Toggle</th> </tr> <?php // Use foreach to access all the courses data foreach ($All_courses as $course) { ?> <tr> <td><?php echo $course['Course_name']; ?></td> <td><?php // Usage of if-else statement to translate the // tinyint status value into some common terms // 0-Inactive // 1-Active if($course['status']=="1") echo "Active"; else echo "Inactive"; ?> </td> <td> <?php if($course['status']=="1") // if a course is active i.e. status is 1 // the toggle button must be able to deactivate // we echo the hyperlink to the page "deactivate.php" // in order to make it look like a button // we use the appropriate css // red-deactivate // green- activate echo "<a href=deactivate.php?id=".$course['id']." class='btn red'>Deactivate</a>"; else echo "<a href=activate.php?id=".$course['id']." class='btn green'>Activate</a>"; ?> </tr> <?php } // End the foreach loop ?> </table> </body> </html>
Producción:
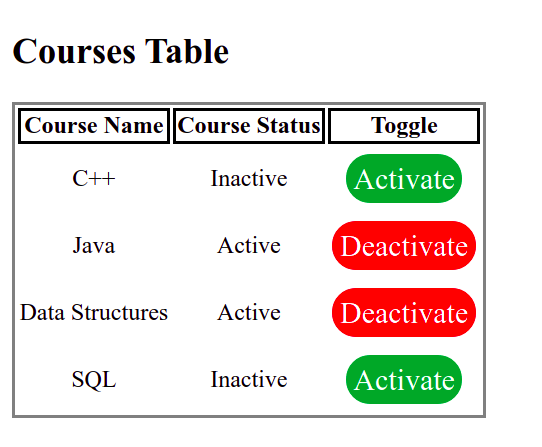
Se crea una mesa de aspecto decente.
En realidad, no estamos usando botones, sino hipervínculos que enlazan con archivos PHP que activan la variable de estado de la tabla «cursos». Así que necesitamos crear estos archivos también.
activate.php
<?php // Connect to database $con=mysqli_connect("localhost","root","","courses"); // Check if id is set or not if true toggle, // else simply go back to the page if (isset($_GET['id'])){ // Store the value from get to a // local variable "course_id" $course_id=$_GET['id']; // SQL query that sets the status // to 1 to indicate activation. $sql="UPDATE `courses` SET `status`=1 WHERE id='$course_id'"; // Execute the query mysqli_query($con,$sql); } // Go back to course-page.php header('location: course-page.php'); ?>
deactivate.php
<?php // Connect to database $con=mysqli_connect("localhost","root","","courses"); // Check if id is set or not, if true, // toggle else simply go back to the page if (isset($_GET['id'])){ // Store the value from get to // a local variable "course_id" $course_id=$_GET['id']; // SQL query that sets the status to // 0 to indicate deactivation. $sql="UPDATE `courses` SET `status`=0 WHERE id='$course_id'"; // Execute the query mysqli_query($con,$sql); } // Go back to course-page.php header('location: course-page.php'); ?>
Salida: hemos creado una página con botones en los que se puede hacer clic y cuando hacemos clic en los botones, el estado cambia en la base de datos.
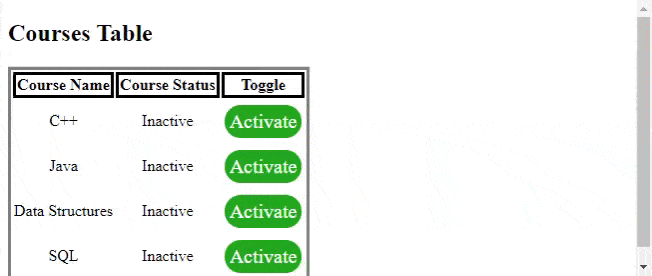
activar y desactivar
Publicación traducida automáticamente
Artículo escrito por arshdeepdgreat y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA