En Java, podemos copiar el contenido de un archivo a otro archivo. Esto se puede hacer mediante las clases FileInputStream y FileOutputStream .
Clase FileInputStream
Es una clase de flujo de entrada de bytes que ayuda a leer los bytes de un archivo. Proporciona diferentes métodos para leer los datos de un archivo.
FileInputStream fin = new FileInputStream(nombre de archivo);
Esto crea un objeto FileInputStream al que se le asigna un nombre de archivo desde donde copiará el contenido o realizará la operación de lectura.
Métodos utilizados:
1. read(): este método se utiliza para leer un byte de datos. Devuelve ese byte como un valor entero o devuelve -1 si se llega al final del archivo.
2. close(): este método se utiliza para cerrar FileInputStream.
Clase FileOutputStream
Es una clase de flujo de salida de bytes que ayuda a escribir los bytes en un archivo. Proporciona diferentes funciones para escribir los datos en un archivo.
FileOutputStream fout = new FileOutputStream(nombre de archivo);
Esto crea un objeto FileOutputStream dado un nombre de archivo en el que escribirá el contenido leído.
Métodos utilizados:
1. write(): este método se utiliza para escribir un byte de datos en FileOutputStream.
2. close(): este método se utiliza para cerrar FileInputStream.
Clase de archivo
Archivo f = nuevo archivo (nombre de archivo);
Esto crea un objeto de archivo llamado f, dado un objeto de string que es el nombre del archivo o la ruta de ubicación de ese archivo al que se debe acceder.
Nota: si el nombre de archivo proporcionado aquí no existe, la clase File creará un nuevo archivo con ese nombre.
Ejemplo:
Java
// Java program to copy content from // one file to another import java.io.*; import java.util.*; public class CopyFromFileaToFileb { public static void copyContent(File a, File b) throws Exception { FileInputStream in = new FileInputStream(a); FileOutputStream out = new FileOutputStream(b); try { int n; // read() function to read the // byte of data while ((n = in.read()) != -1) { // write() function to write // the byte of data out.write(n); } } finally { if (in != null) { // close() function to close the // stream in.close(); } // close() function to close // the stream if (out != null) { out.close(); } } System.out.println("File Copied"); } public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); // get the source file name System.out.println( "Enter the source filename from where you have to read/copy :"); String a = sc.nextLine(); // source file File x = new File(a); // get the destination file name System.out.println( "Enter the destination filename where you have to write/paste :"); String b = sc.nextLine(); // destination file File y = new File(b); // method called to copy the // contents from x to y copyContent(x, y); } }
Producción
Enter the source filename from where you have to read/copy : sourcefile.txt Enter the destination filename where you have to write/paste : destinationfile.txt File Copied
Archivo fuente
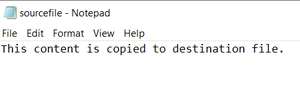
Archivo fuente
Archivo de destino
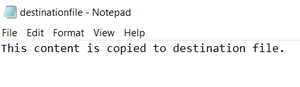
Archivo de destino
Publicación traducida automáticamente
Artículo escrito por yashgupta2808 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA