El siguiente enfoque cubre cómo crear un diseño compartido animado usando framer-motion y ReactJS.
requisitos previos:
- Conocimiento de JavaScript (ES6)
- Conocimientos de HTML/CSS.
- Conocimientos básicos de ReactJS.
Creación de la aplicación React e instalación del módulo:
-
Paso 1: Cree una aplicación React usando el siguiente comando:
npx create-react-app animated-layout
-
Paso 2: después de crear la carpeta de su proyecto, es decir, el diseño animado, muévase a ella con el siguiente comando.
cd animated-layout
-
Paso 3: agregue los paquetes npm que necesitará durante el proyecto:
npm install framer-motion
Ahora abra la carpeta src y elimine los siguientes archivos y cree un archivo JavaScript llamado Item.js.
- logotipo.svg
- serviceWorker.js
- setupTests.js
- App.test.js (si corresponde)
- índice.css
Estructura del proyecto: el árbol de estructura de su proyecto debería verse así:
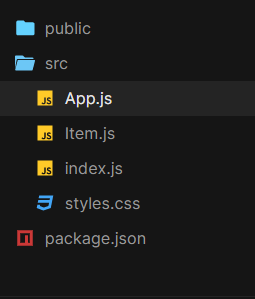
Estructura de carpetas
Ejemplo:
- Vamos a crear un componente Item que es el diseño animado usando el gancho de useState de reacción y los componentes de movimiento de fotogramas motion y AnimatePresence .
- El componente de contenido se utiliza para crear el contenido del elemento (diseño compartido animado) mediante la etiqueta HTML img y el componente de movimiento div y framer- motion .
- El toggleOpen es una función de utilidad para establecer el valor ‘isOpen’ no (!) de su último valor.
- InApp.js , itemList es para la cantidad de diseño compartido animado que queremos crear, en nuestro caso es 3.
- En App.js, vamos a utilizar el componente AnimatedSharedLayout de framer-motion para envolver el componente Item importado y mapear a través de la array ‘itemsList’ para representar diseños animados.
App.js
import React from "react"; import { AnimateSharedLayout } from "framer-motion"; import Item from "./Item"; import "./styles.css"; // This is an example of animating shared layouts // using react and framer-motion library. const itemsList = [ { index: 0, content: `Motion components are DOM primitives optimised for 60fps animation and gestures.` }, { index: 1, content: `Motion can animate: Numbers: 0, 10 etc. Strings containing numbers: "0vh", "10px" etc.` }, { index: 2, content: `Transform properties are accelerated by the GPU, and therefore animate smoothly. ` } ]; const App = () => { return ( // The framer-motion component to wrap Item component to animate it <AnimateSharedLayout> {/* Mapping through itemList array to render layouts*/} {itemsList.map((item) => ( <Item key={item.index} content={item.content} /> ))} </AnimateSharedLayout> ); }; export default App;
Item.js
import React, { useState } from "react"; import { motion, AnimatePresence } from "framer-motion"; const Content = ({ content }) => { const url = "https://media.geeksforgeeks.org/wp-content/cdn-uploads/" + "20200817185016/gfg_complete_logo_2x-min.png" return ( <motion.div layout initial={{ opacity: 0 }} animate={{ opacity: 1 }} exit={{ opacity: 0 }} > <img src={url} alt="geeksforgeeks" /> <div className="row">{content}</div> </motion.div> ); }; const Item = ({ content }) => { // React useState hook is used to manage the state of 'isOpen' // that in turn toggles shared layout, user clicks on const [isOpen, setIsOpen] = useState(false); // Utility function to set 'isOpen' '!'(not) of its last value const toggleOpen = () => setIsOpen(!isOpen); const url = "https://yt3.ggpht.com/ytc/AAUvwnjJqZG9PvGfC3GoV" + "27UlohMeBLxyUdhs9hUbc-Agw=s900-c-k-c0x00ffffff-no-rj" return ( <motion.li layout title="Click to reveal" onClick={toggleOpen} initial={{ borderRadius: [25] }} > <motion.div className="avatar" layout> {" "} <img src={url} alt="gfg" />{" "} </motion.div> <br /> <AnimatePresence>{isOpen && <Content content={content} />} </AnimatePresence> </motion.li> ); }; export default Item;
styles.css
body { min-height: 100vh; margin: 0; display: flex; justify-content: center; align-items: center; } * { box-sizing: border-box; } ul, li { list-style: none; margin: 0; padding: 0; } ul { width: 300px; display: flex; flex-direction: column; background: #fcfcfc; padding: 20px; border-radius: 25px; } li { background-color: rgba(214, 214, 214, 0.5); border-radius: 10px; padding: 20px; margin-bottom: 20px; overflow: hidden; cursor: pointer; width: 300px; } li:last-child { margin-bottom: 0px; } .avatar { width: 40px; height: 40px; border-radius: 20px; } .avatar img { width: 40px; border-radius: 100%; } .row { margin-top: 12px; } img { width: 250px; height: 40px; }
Paso para ejecutar la aplicación: ejecute la aplicación utilizando el siguiente comando desde el directorio raíz del proyecto:
npm start
Salida: Ahora abra su navegador y vaya a http://localhost:3000/ , verá la siguiente salida:
Publicación traducida automáticamente
Artículo escrito por jt9999709701 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA