La función ortho() en p5.js se usa para establecer la proyección ortográfica de un boceto 3D. En esta proyección, todos los objetos con la misma dimensión aparecen del mismo tamaño, independientemente de su distancia a la cámara. El método p5.ortho() se encuentra en la sección de la cámara en la biblioteca p5.js. Los parámetros opcionales se pueden utilizar para especificar el tronco de la cámara en todos los planos.
Sintaxis:
ortho([left], [right], [bottom], [top], [near], [far])
Parámetros: esta función acepta seis parámetros, como se mencionó anteriormente y se describe a continuación:
- izquierda: Es un número que define el plano izquierdo troncocónico de la cámara. Este es un parámetro opcional.
- right: Es un número que define el plano tronco-derecho de la cámara. Este es un parámetro opcional.
- bottom: Es un número que define el plano inferior troncocónico de la cámara. Este es un parámetro opcional.
- top: Es un número que define el plano superior troncocónico de la cámara. Este es un parámetro opcional.
- cerca: Es un número que define el tronco de la cámara cerca del plano. Este es un parámetro opcional.
- far: Es un número que define el plano lejano frustum de la cámara. Este es un parámetro opcional.
Nota: Aquí, los parámetros entre corchetes son opcionales. Y si no se proporcionaron parámetros, los parámetros predeterminados utilizados en ortho() son:
ortho(-width/2, width/2, -height/2, height/2)
Los siguientes ejemplos ilustran la función ortho() en p5.js:
Ejemplo 1: Sin usar proyección ortográfica.
Código:
Javascript
function setup() { // Set the canvas createCanvas(600, 600, WEBGL); } function draw() { // Set the background background(175); // Set the point light of the sketch pointLight(255, 255, 255, 0, -200, 200); // Create multiple cubes in the plane for (let x = -200; x < 200; x += 50) { push(); translate(x, 0, x - 200); rotateZ(frameCount * 0.02); rotateX(frameCount * 0.02); normalMaterial(); box(50); pop(); } }
Producción:
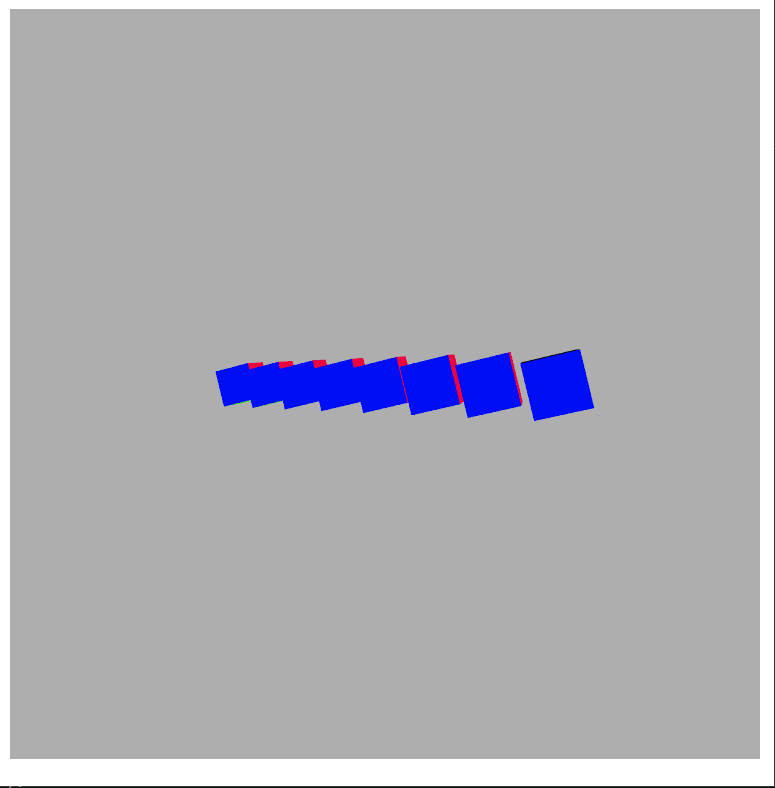
Sin usar proyección ortográfica
Ejemplo 2: Uso de una proyección ortográfica con la función ortho().
Código:
Javascript
function setup() { // Set the canvas createCanvas(600, 600, WEBGL); } function draw() { // Set the background background(175); // Use the orthographic projection ortho(-200, 200, 200, -200, 0.1, 1000); pointLight(255, 255, 255, 0, -200, 200); // Create multiple cubes in the plane for (let x = -200; x < 200; x += 50) { push(); translate(x, 0, x - 200); rotateZ(frameCount * 0.02); rotateX(frameCount * 0.02); normalMaterial(); box(50); pop(); } }
Producción:
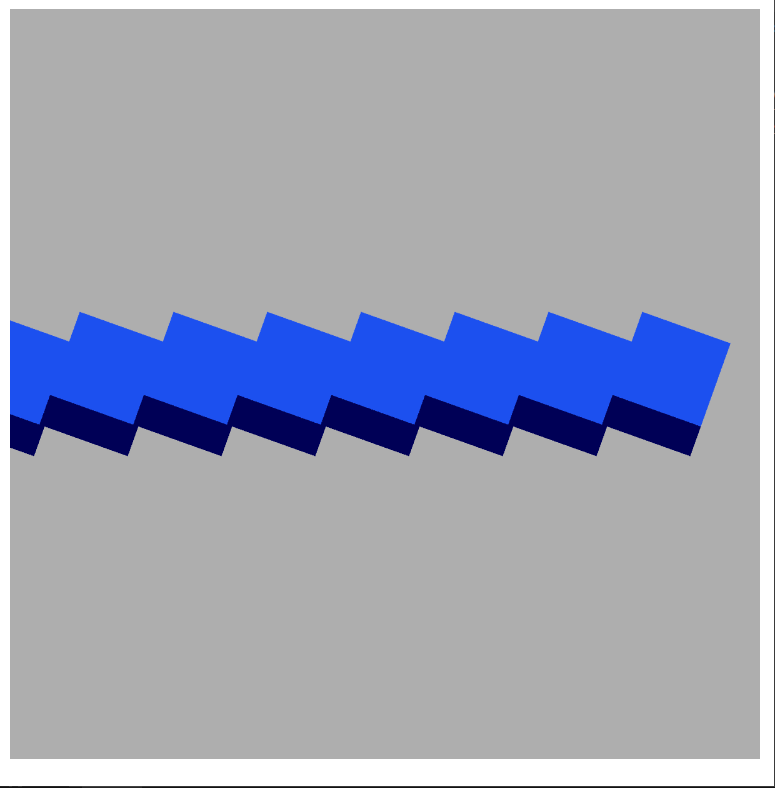
Uso de la proyección ortográfica
Ejemplo 3:
Código:
Javascript
function setup() { // creating canvas with given dimensions createCanvas(400, 400,WEBGL); // calling ortho method with default parameters ortho(); } function draw() { // creating a window with white background background(255); // used to control the objects in projection orbitControl(); // it will the fill the object with colors, // so we can easily distinguish between two objects normalMaterial(); // push will save the current drawing push(); // translate will transform // the object by given width and height translate(30, 0); // box will draw box on the screen // with width,height and depth of 100 box(100); // pop will load the saved drawing pop(); // push will save the current drawing push(); // translate will transform // the object by given width and height translate(-150,0); // box will draw box on the screen // with width,height and depth of 100 box(50); // pop will load the saved drawing pop(); }
Producción:
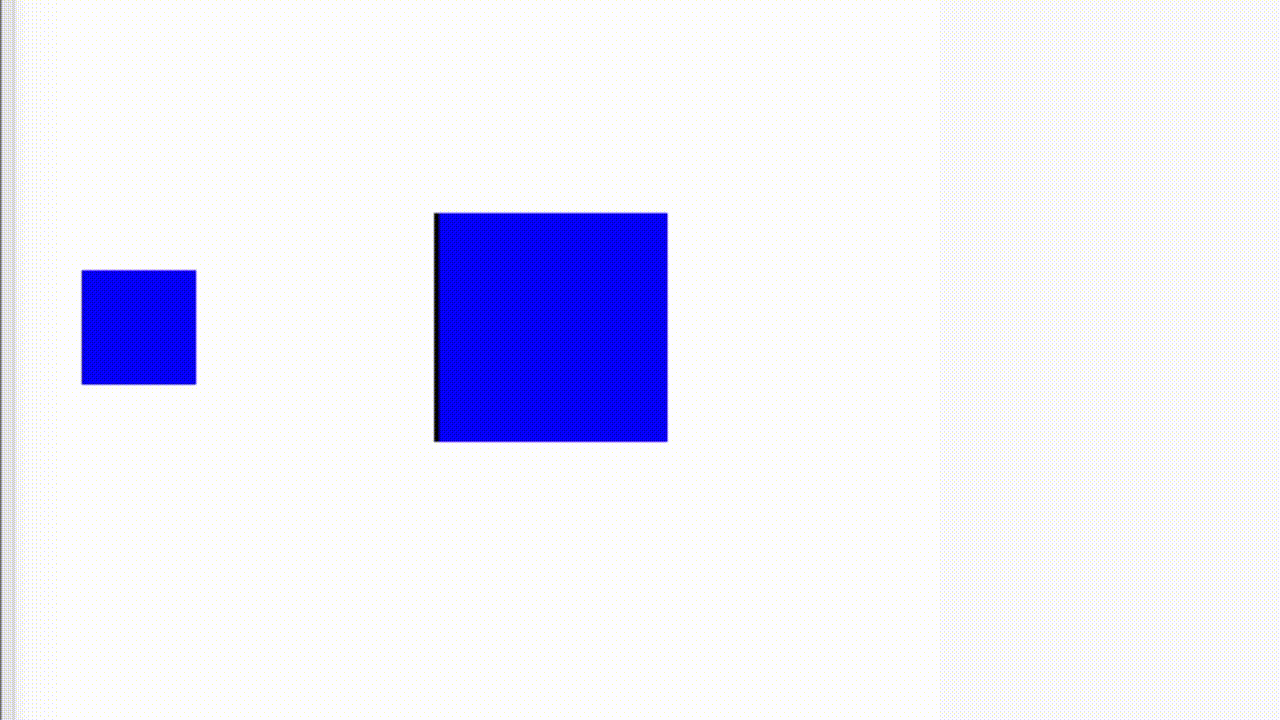
Uso de la proyección ortográfica
Referencia: https://p5js.org/reference/#/p5/ortho
Publicación traducida automáticamente
Artículo escrito por _sh_pallavi y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA