Dada una array cuadrada, gírela 90 grados en sentido contrario a las agujas del reloj sin usar ningún espacio adicional.
Ejemplos:
Input: 1 2 3 4 5 6 7 8 9 Output: 3 6 9 2 5 8 1 4 7 Rotated the input matrix by 90 degrees in anti-clockwise direction. Input: 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 Output: 4 8 12 16 3 7 11 15 2 6 10 14 1 5 9 13 Rotated the input matrix by 90 degrees in anti-clockwise direction.
Un enfoque que requiere espacio adicional ya se analiza en un artículo diferente:
Array cuadrada rotada en el lugar 90 grados | Serie 1
Esta publicación analiza el mismo problema con un enfoque diferente que está optimizado para el espacio.
Enfoque: La idea es encontrar la transpuesta de la array y luego invertir las columnas de la array transpuesta.
Aquí hay un ejemplo para mostrar cómo funciona esto.
Algoritmo:
- Para resolver el problema dado hay dos tareas. El primero es encontrar la transposición y el segundo es invertir las columnas sin usar espacio adicional.
- Una transposición de una array es cuando la array se voltea sobre su diagonal, es decir, el índice de fila de un elemento se convierte en el índice de columna y viceversa. Entonces, para encontrar el intercambio transpuesto de los elementos en la posición (i, j) con (j, i). Ejecute dos bucles, el bucle exterior desde 0 hasta el recuento de filas y el bucle interior desde 0 hasta el índice del bucle exterior.
- Para invertir la columna de la array transpuesta, ejecute dos bucles anidados, el bucle exterior desde 0 hasta el número de columnas y el bucle interior desde 0 hasta el número de filas/2, intercambie elementos en (i, j) con (i, fila[cuenta- 1-j]), donde i y j son índices del bucle interior y exterior respectivamente.
Implementación:
C++
// C++ program for left // rotation of matrix by 90 degree // without using extra space #include <bits/stdc++.h> using namespace std; #define R 4 #define C 4 // After transpose we swap // elements of column // one by one for finding left // rotation of matrix // by 90 degree void reverseColumns(int arr[R][C]) { for (int i = 0; i < C; i++) for (int j = 0, k = C - 1; j < k; j++, k--) swap(arr[j][i], arr[k][i]); } // Function for do transpose of matrix void transpose(int arr[R][C]) { for (int i = 0; i < R; i++) for (int j = i; j < C; j++) swap(arr[i][j], arr[j][i]); } // Function for print matrix void printMatrix(int arr[R][C]) { for (int i = 0; i < R; i++) { for (int j = 0; j < C; j++) cout << arr[i][j] << " "; cout << '\n'; } } // Function to anticlockwise // rotate matrix by 90 degree void rotate90(int arr[R][C]) { transpose(arr); reverseColumns(arr); } // Driven code int main() { int arr[R][C] = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; rotate90(arr); printMatrix(arr); return 0; }
Java
// JAVA Code for left Rotation of a // matrix by 90 degree without using // any extra space import java.util.*; class GFG { // After transpose we swap elements of // column one by one for finding left // rotation of matrix by 90 degree static void reverseColumns(int arr[][]) { for (int i = 0; i < arr[0].length; i++) for (int j = 0, k = arr[0].length - 1; j < k; j++, k--) { int temp = arr[j][i]; arr[j][i] = arr[k][i]; arr[k][i] = temp; } } // Function for do transpose of matrix static void transpose(int arr[][]) { for (int i = 0; i < arr.length; i++) for (int j = i; j < arr[0].length; j++) { int temp = arr[j][i]; arr[j][i] = arr[i][j]; arr[i][j] = temp; } } // Function for print matrix static void printMatrix(int arr[][]) { for (int i = 0; i < arr.length; i++) { for (int j = 0; j < arr[0].length; j++) System.out.print(arr[i][j] + " "); System.out.println(""); } } // Function to anticlockwise rotate // matrix by 90 degree static void rotate90(int arr[][]) { transpose(arr); reverseColumns(arr); } /* Driver program to test above function */ public static void main(String[] args) { int arr[][] = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; rotate90(arr); printMatrix(arr); } } // This code is contributed by Arnav Kr. Mandal.
C#
// C# program for left rotation // of matrix by 90 degree // without using extra space using System; class GFG { static int R = 4; static int C = 4; // After transpose we swap // elements of column one // by one for finding left // rotation of matrix by // 90 degree static void reverseColumns(int[, ] arr) { for (int i = 0; i < C; i++) for (int j = 0, k = C - 1; j < k; j++, k--) { int temp = arr[j, i]; arr[j, i] = arr[k, i]; arr[k, i] = temp; } } // Function for do // transpose of matrix static void transpose(int[, ] arr) { for (int i = 0; i < R; i++) for (int j = i; j < C; j++) { int temp = arr[j, i]; arr[j, i] = arr[i, j]; arr[i, j] = temp; } } // Function for print matrix static void printMatrix(int[, ] arr) { for (int i = 0; i < R; i++) { for (int j = 0; j < C; j++) Console.Write(arr[i, j] + " "); Console.WriteLine(""); } } // Function to anticlockwise // rotate matrix by 90 degree static void rotate90(int[, ] arr) { transpose(arr); reverseColumns(arr); } // Driver code static void Main() { int[, ] arr = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; rotate90(arr); printMatrix(arr); } // This code is contributed // by Sam007 }
Python3
# Python 3 program for left rotation of matrix by 90 # degree without using extra space R = 4 C = 4 # After transpose we swap elements of column # one by one for finding left rotation of matrix # by 90 degree def reverseColumns(arr): for i in range(C): j = 0 k = C-1 while j < k: t = arr[j][i] arr[j][i] = arr[k][i] arr[k][i] = t j += 1 k -= 1 # Function for do transpose of matrix def transpose(arr): for i in range(R): for j in range(i, C): t = arr[i][j] arr[i][j] = arr[j][i] arr[j][i] = t # Function for print matrix def printMatrix(arr): for i in range(R): for j in range(C): print(str(arr[i][j]), end=" ") print() # Function to anticlockwise rotate matrix # by 90 degree def rotate90(arr): transpose(arr) reverseColumns(arr) # Driven code arr = [[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16] ] rotate90(arr) printMatrix(arr)
PHP
<?php // PHP program for left rotation of matrix by 90 $R = 4; $C = 4; // Function to rotate the matrix by 90 degree function reverseColumns(&$arr) { global $C; for ($i = 0; $i < $C; $i++) { for ($j = 0, $k = $C - 1; $j < $k; $j++, $k--) { $t = $arr[$j][$i]; $arr[$j][$i] = $arr[$k][$i]; $arr[$k][$i] = $t; } } } // Function for transpose of matrix function transpose(&$arr) { global $R, $C; for ($i = 0; $i < $R; $i++) { for ($j = $i; $j < $C; $j++) { $t = $arr[$i][$j]; $arr[$i][$j] = $arr[$j][$i]; $arr[$j][$i] = $t; } } } // Function for display the matrix function printMatrix(&$arr) { global $R, $C; for ($i = 0; $i < $R; $i++) { for ($j = 0; $j < $C; $j++) echo $arr[$i][$j]." "; echo "\n"; } } // Function to anticlockwise rotate matrix // by 90 degree function rotate90(&$arr) { transpose($arr); reverseColumns($arr); } // Driven code $arr = array( array( 1, 2, 3, 4 ), array( 5, 6, 7, 8 ), array( 9, 10, 11, 12 ), array( 13, 14, 15, 16 ) ); rotate90($arr); printMatrix($arr); return 0; ?>
Javascript
<script> // JAVASCRIPT Code for left Rotation of a // matrix by 90 degree without using // any extra space // After transpose we swap elements of // column one by one for finding left // rotation of matrix by 90 degree function reverseColumns(arr) { for (let i = 0; i < arr[0].length; i++) for (let j = 0, k = arr[0].length - 1; j < k; j++, k--) { let temp = arr[j][i]; arr[j][i] = arr[k][i]; arr[k][i] = temp; } } // Function for do transpose of matrix function transpose(arr) { for (let i = 0; i < arr.length; i++) for (let j = i; j < arr[0].length; j++) { let temp = arr[j][i]; arr[j][i] = arr[i][j]; arr[i][j] = temp; } } // Function for print matrix function printMatrix(arr) { for (let i = 0; i < arr.length; i++) { for (let j = 0; j < arr[0].length; j++) document.write(arr[i][j] + " "); document.write("<br>"); } } // Function to anticlockwise rotate // matrix by 90 degree function rotate90(arr) { transpose(arr); reverseColumns(arr); } /* Driver program to test above function */ let arr = [[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16] ]; rotate90(arr) printMatrix(arr) // This code is contributed by rag2127 </script>
4 8 12 16 3 7 11 15 2 6 10 14 1 5 9 13
Análisis de Complejidad:
- Complejidad del tiempo :O(R*C).
La array se recorre dos veces, por lo que la complejidad es O(R*C). - Complejidad espacial :O(1).
La complejidad del espacio es constante ya que no se requiere espacio extra.
Otro enfoque utilizando un solo recorrido de la array:
La idea es atravesar los límites de la array y desplazar las posiciones de los elementos en 90 0 sentido antihorario en cada límite. Hay tales (n/2-1) límites en la array.
Algoritmo:
- Iterar sobre todos los límites de la array. Hay límites totales (n/2-1)
- Para cada límite, tome los 4 elementos de las esquinas e intercámbielos de manera que los 4 elementos de las esquinas giren en sentido contrario a las agujas del reloj. Luego tome los siguientes 4 elementos a lo largo de los bordes (izquierda, derecha, arriba, abajo) e intercámbielos en sentido contrario a las agujas del reloj. Continúe mientras todos los elementos en ese límite en particular se giren 90 0 en sentido contrario a las agujas del reloj.
- Luego pase al siguiente límite interno y continúe el proceso mientras toda la array se gira en 90 0 en dirección contraria a las manecillas del reloj.
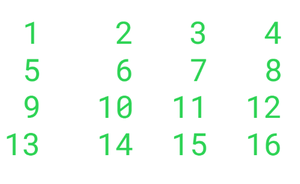
array original
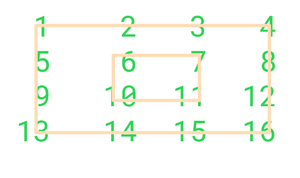
límites totales n/2-1

para el límite más exterior
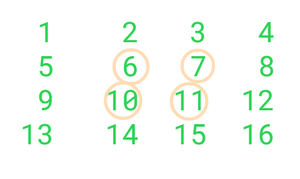
para el siguiente límite interior
Implementación:
C++14
// C++ program for left rotation of matrix // by 90 degree without using extra space #include <bits/stdc++.h> using namespace std; #define R 4 #define C 4 // Function to rotate matrix anticlockwise by 90 degrees. void rotateby90(int arr[R][C]) { int n = R; // n=size of the square matrix int a = 0, b = 0, c = 0, d = 0; // iterate over all the boundaries of the matrix for (int i = 0; i <= n / 2 - 1; i++) { // for each boundary, keep on taking 4 elements (one // each along the 4 edges) and swap them in // anticlockwise manner for (int j = 0; j <= n - 2 * i - 2; j++) { a = arr[i + j][i]; b = arr[n - 1 - i][i + j]; c = arr[n - 1 - i - j][n - 1 - i]; d = arr[i][n - 1 - i - j]; arr[i + j][i] = d; arr[n - 1 - i][i + j] = a; arr[n - 1 - i - j][n - 1 - i] = b; arr[i][n - 1 - i - j] = c; } } } // Function for print matrix void printMatrix(int arr[R][C]) { for (int i = 0; i < R; i++) { for (int j = 0; j < C; j++) cout << arr[i][j] << " "; cout << '\n'; } } // Driven code int main() { int arr[R][C] = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; rotateby90(arr); printMatrix(arr); return 0; } // This code is contributed by Md. Enjamum // Hossain(enja_2001)
Java
// JAVA Code for left Rotation of a matrix // by 90 degree without using any extra space import java.util.*; class GFG { // Function to rotate matrix anticlockwise by 90 // degrees. static void rotateby90(int arr[][]) { int n = arr.length; int a = 0, b = 0, c = 0, d = 0; // iterate over all the boundaries of the matrix for (int i = 0; i <= n / 2 - 1; i++) { // for each boundary, keep on taking 4 elements // (one each along the 4 edges) and swap them in // anticlockwise manner for (int j = 0; j <= n - 2 * i - 2; j++) { a = arr[i + j][i]; b = arr[n - 1 - i][i + j]; c = arr[n - 1 - i - j][n - 1 - i]; d = arr[i][n - 1 - i - j]; arr[i + j][i] = d; arr[n - 1 - i][i + j] = a; arr[n - 1 - i - j][n - 1 - i] = b; arr[i][n - 1 - i - j] = c; } } } // Function for print matrix static void printMatrix(int arr[][]) { for (int i = 0; i < arr.length; i++) { for (int j = 0; j < arr[0].length; j++) System.out.print(arr[i][j] + " "); System.out.println(""); } } /* Driver program to test above function */ public static void main(String[] args) { int arr[][] = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; rotateby90(arr); printMatrix(arr); } } // This code is contributed by Md. Enjamum // Hossain(enja_2001)
Python3
# Function to rotate matrix anticlockwise by 90 degrees. def rotateby90(arr): n = len(arr) a,b,c,d = 0,0,0,0 # iterate over all the boundaries of the matrix for i in range(n // 2): # for each boundary, keep on taking 4 elements # (one each along the 4 edges) and swap them in # anticlockwise manner for j in range(n - 2 * i - 1): a = arr[i + j][i] b = arr[n - 1 - i][i + j] c = arr[n - 1 - i - j][n - 1 - i] d = arr[i][n - 1 - i - j] arr[i + j][i] = d arr[n - 1 - i][i + j] = a arr[n - 1 - i - j][n - 1 - i] = b arr[i][n - 1 - i - j] = c # Function for print matrix def printMatrix(arr): for i in range(len(arr)): for j in range(len(arr[0])): print(arr[i][j] ,end = " ") print() # Driver program to test above function arr=[[ 1, 2, 3, 4 ], [ 5, 6, 7, 8 ], [ 9, 10, 11, 12 ], [ 13, 14, 15, 16 ]] rotateby90(arr) printMatrix(arr) # this code is contributed by shinjanpatra
C#
// C# Code for left Rotation of a matrix // by 90 degree without using any extra space using System; using System.Collections.Generic; public class GFG { // Function to rotate matrix anticlockwise by 90 // degrees. static void rotateby90(int [,]arr) { int n = arr.GetLength(0); int a = 0, b = 0, c = 0, d = 0; // iterate over all the boundaries of the matrix for (int i = 0; i <= n / 2 - 1; i++) { // for each boundary, keep on taking 4 elements // (one each along the 4 edges) and swap them in // anticlockwise manner for (int j = 0; j <= n - 2 * i - 2; j++) { a = arr[i + j,i]; b = arr[n - 1 - i,i + j]; c = arr[n - 1 - i - j,n - 1 - i]; d = arr[i,n - 1 - i - j]; arr[i + j,i] = d; arr[n - 1 - i,i + j] = a; arr[n - 1 - i - j,n - 1 - i] = b; arr[i,n - 1 - i - j] = c; } } } // Function for print matrix static void printMatrix(int [,]arr) { for (int i = 0; i < arr.GetLength(0); i++) { for (int j = 0; j < arr.GetLength(1); j++) Console.Write(arr[i,j] + " "); Console.WriteLine(""); } } /* Driver program to test above function */ public static void Main(String[] args) { int [,]arr = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; rotateby90(arr); printMatrix(arr); } } // This code is contributed by umadevi9616
Javascript
<script> // JavaScript Code for left Rotation of a matrix // by 90 degree without using any extra space // Function to rotate matrix anticlockwise by 90 // degrees. function rotateby90(arr) { let n = arr.length; let a = 0, b = 0, c = 0, d = 0; // iterate over all the boundaries of the matrix for (let i = 0; i <= n / 2 - 1; i++) { // for each boundary, keep on taking 4 elements // (one each along the 4 edges) and swap them in // anticlockwise manner for (let j = 0; j <= n - 2 * i - 2; j++) { a = arr[i + j][i]; b = arr[n - 1 - i][i + j]; c = arr[n - 1 - i - j][n - 1 - i]; d = arr[i][n - 1 - i - j]; arr[i + j][i] = d; arr[n - 1 - i][i + j] = a; arr[n - 1 - i - j][n - 1 - i] = b; arr[i][n - 1 - i - j] = c; } } } // Function for print matrix function printMatrix(arr) { for (let i = 0; i < arr.length; i++) { for (let j = 0; j < arr[0].length; j++) document.write(arr[i][j] + " "); document.write("<br>"); } } /* Driver program to test above function */ let arr=[[ 1, 2, 3, 4 ], [ 5, 6, 7, 8 ], [ 9, 10, 11, 12 ], [ 13, 14, 15, 16 ]]; rotateby90(arr); printMatrix(arr); // This code is contributed by avanitrachhadiya2155 </script>
4 8 12 16 3 7 11 15 2 6 10 14 1 5 9 13
Análisis de Complejidad:
Complejidad del tiempo :O(R*C). La array se recorre una sola vez, por lo que la complejidad temporal es O(R*C).
Complejidad espacial :O(1) . La complejidad del espacio es O(1) ya que no se requiere espacio extra.
Implementación : veamos un método de Python numpy que se puede usar para llegar a la solución particular.
Python3
# Alternative implementation using numpy import numpy # Driven code arr = [[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16] ] # Define flip algorithm (Note numpy.flip is a builtin f # function for versions > v.1.12.0) def flip(m, axis): if not hasattr(m, 'ndim'): m = asarray(m) indexer = [slice(None)] * m.ndim try: indexer[axis] = slice(None, None, -1) except IndexError: raise ValueError("axis =% i is invalid for the % i-dimensional input array" % (axis, m.ndim)) return m[tuple(indexer)] # Transpose the matrix trans = numpy.transpose(arr) # Flip the matrix anti-clockwise (1 for clockwise) flipmat = flip(trans, 0) print("\nnumpy implementation\n") print(flipmat)
Producción:
numpy implementation [[ 4 8 12 16] [ 3 7 11 15] [ 2 6 10 14] [ 1 5 9 13]]
Nota: Los pasos/programas anteriores giran hacia la izquierda (o hacia la izquierda). Veamos cómo hacer la rotación correcta o la rotación en el sentido de las agujas del reloj. El enfoque sería similar. Encuentre la transpuesta de la array y luego invierta las filas de la array transpuesta.
Así es como se hace.
Este artículo es una contribución de DANISH_RAZA . Si te gusta GeeksforGeeks y te gustaría contribuir, también puedes escribir un artículo usando write.geeksforgeeks.org o enviar tu artículo por correo a review-team@geeksforgeeks.org. Vea su artículo que aparece en la página principal de GeeksforGeeks y ayude a otros Geeks.
Girando a lo largo de los límites
Podemos comenzar en las primeras 4 esquinas de la array dada y luego seguir incrementando los índices de fila y columna para movernos.
En cualquier momento tendremos cuatro esquinas lu (izquierda arriba), ld (izquierda abajo), ru (derecha arriba), rd (derecha abajo).
Para girar a la izquierda, primero intercambiaremos ru y ld, luego lu y ld y, por último, ru y rd.
tu | Lu | |
rd | viejo |
Implementación:
C++
#include <bits/stdc++.h> using namespace std; //Function to rotate matrix anticlockwise by 90 degrees. void rotateby90(vector<vector<int> >& matrix) { int n=matrix.size(); int mid; if(n%2==0) mid=n/2-1; else mid=n/2; for(int i=0,j=n-1;i<=mid;i++,j--) { for(int k=0;k<j-i;k++) { swap(matrix[i][j-k],matrix[j][i+k]); //ru ld swap(matrix[i+k][i],matrix[j][i+k]); //lu ld swap(matrix[i][j-k],matrix[j-k][j]); //ru rd } } } void printMatrix(vector<vector<int>>& arr) { int n=arr.size(); for(int i=0;i<n;i++) { for(int j=0;j<n;j++) { cout<<arr[i][j]<<" "; } cout<<endl; } } int main() { vector<vector<int>> arr = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; rotateby90(arr); printMatrix(arr); return 0; }
Java
import java.util.*; class GFG{ private static int[][] swap(int[][] matrix, int x1,int x2,int y1, int y2) { int temp = matrix[x1][x2] ; matrix[x1][x2] = matrix[y1][y2]; matrix[y1][y2] = temp; return matrix; } //Function to rotate matrix anticlockwise by 90 degrees. static int[][] rotateby90(int[][] matrix) { int n=matrix.length; int mid; if(n % 2 == 0) mid = n / 2 - 1; else mid = n / 2; for(int i = 0, j = n - 1; i <= mid; i++, j--) { for(int k = 0; k < j - i; k++) { matrix= swap(matrix, i, j - k, j, i + k); //ru ld matrix= swap(matrix, i + k, i, j, i + k); //lu ld matrix= swap(matrix, i, j - k, j - k, j); //ru rd } } return matrix; } static void printMatrix(int[][] arr) { int n = arr.length; for(int i = 0; i < n; i++) { for(int j = 0; j < n; j++) { System.out.print(arr[i][j] + " "); } System.out.println(); } } public static void main(String[] args) { int[][] arr = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; arr = rotateby90(arr); printMatrix(arr); } } // This code is contributed by umadevi9616
C#
using System; public class GFG{ private static int[,] swap(int[,] matrix, int x1,int x2,int y1, int y2) { int temp = matrix[x1,x2] ; matrix[x1,x2] = matrix[y1,y2]; matrix[y1,y2] = temp; return matrix; } //Function to rotate matrix anticlockwise by 90 degrees. static int[,] rotateby90(int[,] matrix) { int n=matrix.GetLength(0); int mid; if(n % 2 == 0) mid = (n / 2 - 1); else mid = (n / 2); for(int i = 0, j = n - 1; i <= mid; i++, j--) { for(int k = 0; k < j - i; k++) { matrix= swap(matrix, i, j - k, j, i + k); //ru ld matrix= swap(matrix, i + k, i, j, i + k); //lu ld matrix= swap(matrix, i, j - k, j - k, j); //ru rd } } return matrix; } static void printMatrix(int[,] arr) { int n = arr.GetLength(0); for(int i = 0; i < n; i++) { for(int j = 0; j < n; j++) { Console.Write(arr[i,j] + " "); } Console.WriteLine(); } } public static void Main(String[] args) { int[,] arr = { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; arr = rotateby90(arr); printMatrix(arr); } } // This code is contributed by Rajput-Ji
Javascript
<script> function swap(matrix , x1 , x2 , y1 , y2) { var temp = matrix[x1][x2]; matrix[x1][x2] = matrix[y1][y2]; matrix[y1][y2] = temp; return matrix; } // Function to rotate matrix anticlockwise by 90 degrees. function rotateby90(matrix) { var n = matrix.length; var mid; if (n % 2 == 0) mid = n / 2 - 1; else mid = n / 2; for (i = 0, j = n - 1; i <= mid; i++, j--) { for (k = 0; k < j - i; k++) { matrix = swap(matrix, i, j - k, j, i + k); // ru ld matrix = swap(matrix, i + k, i, j, i + k); // lu ld matrix = swap(matrix, i, j - k, j - k, j); // ru rd } } return matrix; } function printMatrix(arr) { var n = arr.length; for (i = 0; i < n; i++) { for (j = 0; j < n; j++) { document.write(arr[i][j] + " "); } document.write("<br/>"); } } var arr = [ [ 1, 2, 3, 4 ], [ 5, 6, 7, 8 ], [ 9, 10, 11, 12 ], [ 13, 14, 15, 16 ] ]; arr = rotateby90(arr); printMatrix(arr); // This code is contributed by Rajput-Ji </script>
Python3
# Function to rotate matrix anticlockwise by 90 degrees. def rotateby90(arr): n = len(arr) if(n%2 == 0): mid = n//2-1 else: mid = n/2 j=n-1 # iterate over all the boundaries of the matrix for i in range(mid+1): for k in range(j-i): arr[i][j-k],arr[j][i+k] = arr[j][i+k],arr[i][j-k] arr[i+k][i],arr[j][i+k] = arr[j][i+k],arr[i+k][i] arr[i][j-k],arr[j-k][j] = arr[j-k][j],arr[i][j-k] j=j-1 # Function for print matrix def printMatrix(arr): for i in range(len(arr)): for j in range(len(arr[0])): print(arr[i][j] ,end = " ") print() # Driver program to test above function arr=[[ 1, 2, 3, 4 ], [ 5, 6, 7, 8 ], [ 9, 10, 11, 12 ], [ 13, 14, 15, 16 ]] rotateby90(arr) printMatrix(arr) # this code is contributed by CodeWithMini
4 8 12 16 3 7 11 15 2 6 10 14 1 5 9 13
Complejidad de tiempo: O(n^2)
Complejidad de espacio: O(1)
Publicación traducida automáticamente
Artículo escrito por GeeksforGeeks-1 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA