En Hibernate, para obtener una colección ordenada de elementos, la mayoría de las veces List es la preferida. Junto con List, tenemos diferentes mapas de colección como Bag, Set, Map, SortedSet, SortedMap, etc., pero todavía en muchos lugares mapeo list es la forma preferida ya que tiene el elemento de índice y, por lo tanto, es más fácil realizar cualquier operación CRUD. Podemos ver esto aquí a través de los empleados y sus planes de ahorro. Un empleado puede hacer múltiples inversiones. podemos ver eso aquí.
Proyecto de ejemplo
Estructura del proyecto:
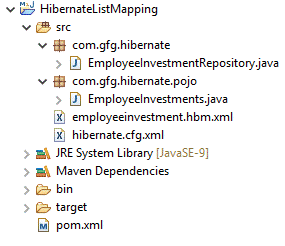
Este es un proyecto impulsado por maven.
pom.xml
XML
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>HibernateListMapping</groupId> <artifactId>HibernateListMapping</artifactId> <version>0.0.1-SNAPSHOT</version> <build> <sourceDirectory>src</sourceDirectory> <resources> <resource> <directory>src</directory> <excludes> <exclude>**/*.java</exclude> </excludes> </resource> </resources> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <release>9</release> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>5.4.15.Final</version> </dependency> <!-- As we are connecting with MySQL, this is needed --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.34</version> </dependency> </dependencies> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> </project>
Veamos los principales archivos de configuración
inversiónempleado.hbm.xml
XML
<?xml version='1.0' encoding='UTF-8'?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 5.3//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="com.gfg.hibernate.pojo.EmployeeInvestments" table="Employee"> <id name="employeeId"> <generator class="increment"></generator> </id> <property name="employeeName"></property> <!-- In list, we need to maintain the order. Hence we should maintain the index element. This is the major difference between bag and list. --> <list name="investments" table="Investments"> <!-- Foreign key connecting employee and investments --> <key column="employeeId"></key> <!-- This will maintain the order of investments, starts with 0 Because of this, it is easier to search and do crud operation --> <index column="type"></index> <element column="investment" type="string"></element></list> </class> </hibernate-mapping>
hibernate.cfg.xml
XML
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- As we are connecting mysql, those driver classes, database name, username and password are specified Please change the information as per your requirement --> <property name="hbm2ddl.auto">update</property> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/geeksforgeeks?serverTimezone=UTC</property> <property name="connection.username">root</property> <property name="connection.password">admin</property> <property name="show_sql">true</property> <mapping resource="employeeinvestment.hbm.xml" /> </session-factory> </hibernate-configuration>
Veamos la clase POJO ahora
InversionesEmpleado.java
Java
import java.util.List; public class EmployeeInvestments { // data member for employee private int employeeId; private String employeeName; // investments private List<String> investments; public int getEmployeeId() { return employeeId; } public void setEmployeeId(int employeeId) { this.employeeId = employeeId; } public String getEmployeeName() { return employeeName; } public void setEmployeeName(String employeeName) { this.employeeName = employeeName; } public List<String> getInvestments() { return investments; } public void setInvestments(List<String> investments) { this.investments = investments; } }
Archivo Java para agregar y enumerar los datos
EmployeeInvestmentRepository.java
Java
import com.gfg.hibernate.pojo.EmployeeInvestments; import java.util.ArrayList; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.boot.Metadata; import org.hibernate.boot.MetadataSources; import org.hibernate.boot.registry.StandardServiceRegistry; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; public class EmployeeInvestmentRepository { public static void main(String[] args) { StandardServiceRegistry standardServiceRegistry = new StandardServiceRegistryBuilder() .configure("hibernate.cfg.xml") .build(); Metadata meta = new MetadataSources(standardServiceRegistry) .getMetadataBuilder() .build(); SessionFactory sessionFactory = meta.buildSessionFactory(); Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); ArrayList<String> investmentList1 = new ArrayList<String>(); investmentList1.add("NSC"); investmentList1.add("PPF"); investmentList1.add("LIC"); ArrayList<String> investmentList2 = new ArrayList<String>(); investmentList2.add("HousingLoan"); investmentList2.add("CarLoan"); investmentList2.add("NSC"); EmployeeInvestments employeeInvestment1 = new EmployeeInvestments(); employeeInvestment1.setEmployeeName("EmployeeA"); employeeInvestment1.setInvestments(investmentList1); EmployeeInvestments employeeInvestment2 = new EmployeeInvestments(); employeeInvestment2.setEmployeeName("EmployeeB"); employeeInvestment2.setInvestments(investmentList2); session.persist(employeeInvestment1); session.persist(employeeInvestment2); transaction.commit(); session.close(); System.out.println( "success. We have seen List mapping here. Check the db data. We can see the index maintained there"); } }
Al ejecutar el proyecto, podemos ver el resultado a continuación.
Producción:
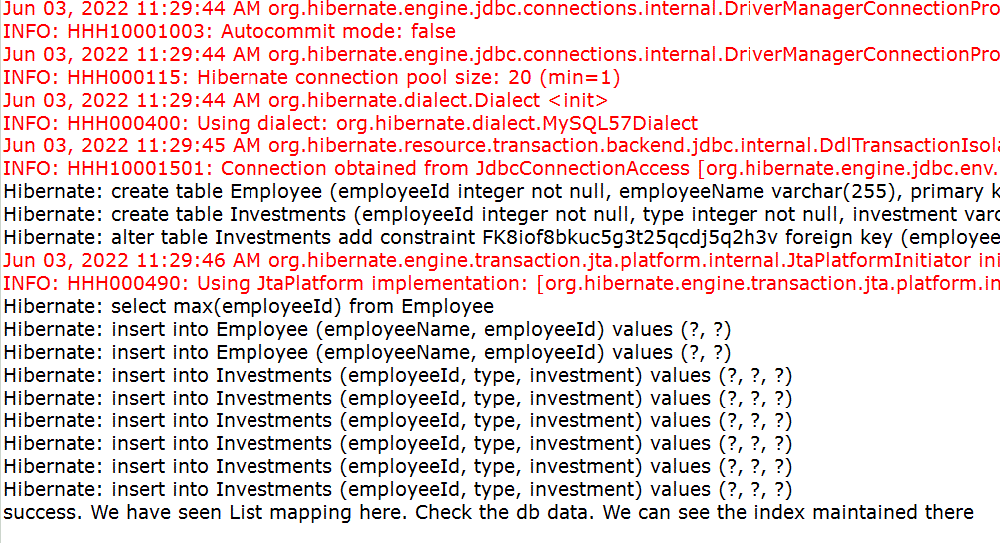
Veamos la consulta que se ejecutó.
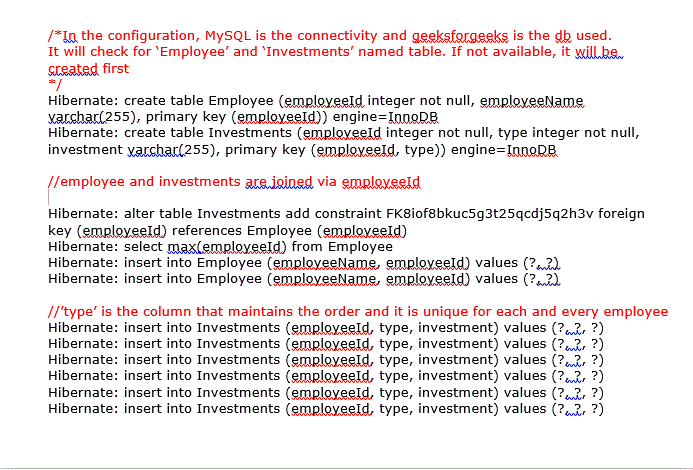
Revisemos los datos de MySQL DB
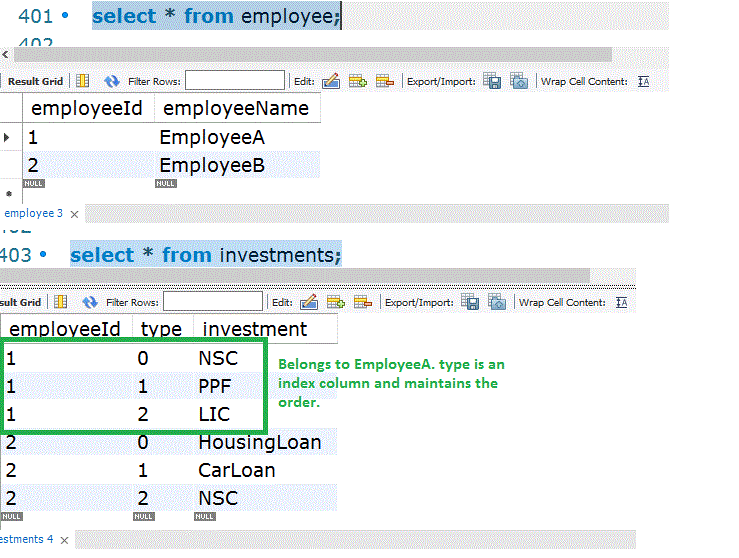
Conclusión
En lo anterior, hemos visto cómo usar la lista de mapeo en hibernación. Siempre que sea necesario ordenar, podemos optar por el mapeo de listas.
Publicación traducida automáticamente
Artículo escrito por priyarajtt y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA