Estaremos explicando los pasos involucrados en la integración de Spring MVC y MySQL . En MySQL, tendremos los datos necesarios que son la columna vertebral de la aplicación. Tomemos una aplicación de muestra que contiene los datos de algunos estudiantes y sus notas NEET. La aplicación Spring MVC interactuará con MySQL y recuperará los datos según sea necesario.
Pasos involucrados en la creación de la base de datos y la tabla
Paso 1: Crear la base de datos
1.1: Crear una base de datos de prueba.
-- test is the name of the database here
Paso 2: Hacer que la prueba de la base de datos sea activa
use test;
Paso 3: Crea la tabla
create table studentsdetails(id int auto_increment primary key, name varchar(25),caste varchar(25),neetmarks int,gender varchar(10));
Paso 4: inserte registros en él
-- insert into studentsdetails(name,caste,neetmarks,gender) values ('Geek1','OBC',600,'Female'); insert into studentsdetails(name,caste,neetmarks,gender) values ('Geek2','General',700,'Female'); insert into studentsdetails(name,caste,neetmarks,gender) values ('Geek3','General',600,'Male'); insert into studentsdetails(name,caste,neetmarks,gender) values ('Geek4','OBC',670,'Male'); insert into studentsdetails(name,caste,neetmarks,gender) values ('Geek5','SC',600,'Female'); insert into studentsdetails(name,caste,neetmarks,gender) values ('Geek6','SC',500,'Male');
Paso 5: seleccione los datos de él
select * from studentsdetails;
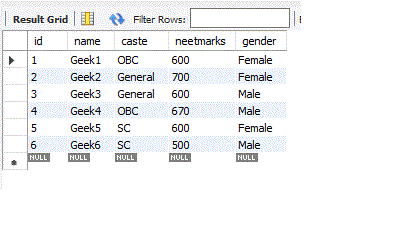
datos de detalles de los estudiantes
Ahora hagamos los pasos necesarios en la aplicación Spring MVC, la estructura del proyecto es la siguiente:
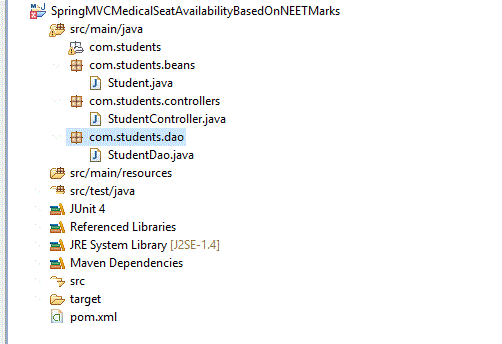
Archivo: pom.xml
XML
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.students</groupId> <artifactId>SpringMVCMedicalSeatAvailabilityBasedOnNEETMarks</artifactId> <packaging>war</packaging> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <version>0.0.1-SNAPSHOT</version> <name>SpringMVCMedicalSeatAvailabilityBasedOnNEETMarks Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework/spring-webmvc --> <dependency> <groupId>org.mockito</groupId> <artifactId>mockito-all</artifactId> <version>1.9.5</version> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.1.1.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.1.1.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-test</artifactId> <version>5.1.1.RELEASE</version> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <version>2.4.1</version> <scope>test</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.tomcat/tomcat-jasper --> <dependency> <groupId>org.apache.tomcat</groupId> <artifactId>tomcat-jasper</artifactId> <version>9.0.12</version> </dependency> <!-- https://mvnrepository.com/artifact/javax.servlet/javax.servlet-api --> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>3.0-alpha-1</version> </dependency> <!-- https://mvnrepository.com/artifact/javax.servlet/jstl --> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> <!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java --> <!-- This is much required to connect to MySQL --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.11</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework/spring-jdbc --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>5.1.1.RELEASE</version> </dependency> </dependencies> <build> <finalName>SpringMVCMedicalSeatAvailabilityBasedOnNEETMarks</finalName> <sourceDirectory>src/main/java</sourceDirectory> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>3.0.0-M3</version> <configuration> <testFailureIgnore>true</testFailureIgnore> <shutdown>kill</shutdown> <!-- Use it if required--> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <version>3.3.2</version> </plugin> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>tomcat-maven-plugin</artifactId> <version>1.0-beta-1</version> </plugin> </plugins> </build> </project>
Archivo: spring-servlet.xml
Este es el archivo más necesario para comunicarse con MySQL
XML
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd"> <context:component-scan base-package="com.students.controllers"></context:component-scan> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/jsp/"></property> <property name="suffix" value=".jsp"></property> </bean> <!-- According to the username and password that we use, the changes need to be done below --> <!-- Generally username will be root and password will be empty or will come with a password Hence accordingly change the data here --> <bean id="ds" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name="driverClassName" value="com.mysql.jdbc.Driver"></property> <!-- <property name="url" value="jdbc:mysql://localhost:3306/test"></property> --> <!-- Here test is the name of the database --> <property name="url" value="jdbc:mysql://localhost:3306/test?user=root&password=password&serverTimezone=UTC"></property> <!-- <property name="username" value="root"></property> <property name="password" value="password"></property> --> </bean> <bean id="jt" class="org.springframework.jdbc.core.JdbcTemplate"> <property name="dataSource" ref="ds"></property> </bean> <bean id="dao" class="com.students.dao.StudentDao"> <property name="template" ref="jt"></property> </bean> </beans>
Pasemos ahora a la ‘ clase de frijoles ‘. Los campos de esta clase de bean deben ser equivalentes a la estructura de la tabla MySQL . Solo así será más fácil y efectivo comunicarse.
Archivo: Student.java
Java
// Java Program to Illustrate Student Class // Class public class Student { // Class data members // Map to studentsdetails.id private int id; // Map to studentsdetails.name private String name; // Map to studentsdetails.caste private String caste; // Map to studentsdetails.neetMarks private int neetMarks; // Map to studentsdetails.gender private String gender; // Getter and setter methods // Getter public int getNeetMarks() { return neetMarks; } // Setter public void setNeetMarks(int neetMarks) { this.neetMarks = neetMarks; } // Getter public String getGender() { return gender; } // Setter public void setGender(String gender) { this.gender = gender; } // Getter public int getId() { return id; } // Setter public void setId(int id) { this.id = id; } // Getter public String getName() { return name; } // Setter public void setName(String name) { this.name = name; } // Getter public String getCaste() { return caste; } // Setter public void setCaste(String caste) { this.caste = caste; } }
Ahora para hacer las operaciones de la base de datos, necesitamos el archivo java DAO
Archivo: StudentDao.java
Java
// Java Program to Illustrate StudentDao Class // Importing required classes import com.students.beans.Student; import java.sql.SQLException; import org.springframework.jdbc.core.BeanPropertyRowMapper; import org.springframework.jdbc.core.JdbcTemplate; // Class public class StudentDao { JdbcTemplate template; public void setTemplate(JdbcTemplate template) { this.template = template; } // We can search the data from MySQL by means of // studentname public Student getStudentsByName(String studentName) throws SQLException { String sql = "select * from studentsdetails where name=?"; return template.queryForObject( sql, new Object[] { studentName }, new BeanPropertyRowMapper<Student>( Student.class)); } // We can search the data from MySQL by means of caste public Student getStudentsByCaste(String caste) throws SQLException { String sql = "select * from studentsdetails where caste=?"; return template.queryForObject( sql, new Object[] { caste }, new BeanPropertyRowMapper<Student>( Student.class)); } // We can search the data from MySQL by means of id public Student getStudentsById(int id) throws SQLException { String sql = "select * from studentsdetails where id =?"; return template.queryForObject( sql, new Object[] { id }, new BeanPropertyRowMapper<Student>( Student.class)); } // We can search the data from MySQL by means of // neetmarks public Student getStudentsByNeetMarks(int neetMarks) throws SQLException { String sql = "select * from studentsdetails where neetMarks=?"; return template.queryForObject( sql, new Object[] { neetMarks }, new BeanPropertyRowMapper<Student>( Student.class)); } // As much of business logic can be written here }
Veamos ahora la clase de controlador.
StudentController.java
Java
// Java Program to Illustrate StudentController Class // Importing required classes import com.students.beans.Student; import com.students.dao.StudentDao; import java.sql.SQLException; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.ModelAttribute; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.SessionAttributes; import org.springframework.web.servlet.ModelAndView; // Class @Controller @SessionAttributes("student") public class StudentController { // @Autowired // It will inject dao from xml file StudentDao dao; @Autowired public StudentController(StudentDao dao) { // this keyword refers to current instance itself this.dao = dao; } @ModelAttribute("student") public Student getStudent() { return new Student(); } // For searchform @RequestMapping("/studentsearchform") public String searchform(Model m) { m.addAttribute("command", new Student()); return "studentsearchform"; } // It provides check students and determines // for medical seat availability based on NEET marks // in model object // It is up to one to change the logic here // for the availability of medical seat // Just to show as an example, below calculations // aretaken Exception need to be handled carefully as // interacting with database @RequestMapping(value = "/checkByNeetMarks", method = RequestMethod.POST) public ModelAndView checkByNeetMarks(@ModelAttribute("student") Student student) { ModelAndView mav = null; Student student1; // Try block to check for exceptions try { student1 = dao.getStudentsByName(student.getName()); mav = new ModelAndView("welcome"); if (null != student1) { System.out.println( student1.getId() + "..." + student1.getName() + ".." + student1.getCaste() + "..neet marks.." + student1.getNeetMarks()); boolean isAvailable = false; if (student1.getCaste().equalsIgnoreCase( "General") && student1.getNeetMarks() >= 600) { isAvailable = true; } if (student1.getCaste().equalsIgnoreCase( "OBC") && student1.getNeetMarks() >= 500) { isAvailable = true; } if (student1.getCaste().equalsIgnoreCase( "SC") && student1.getNeetMarks() >= 400) { isAvailable = true; } mav.addObject("firstname", student1.getName()); if (isAvailable) { mav.addObject( "availability", "Eligible to get Medical Seat"); } else { mav.addObject( "availability", "Not eligible to get Medical Seat"); } mav.addObject("caste", student1.getCaste()); mav.addObject("neetmarks", student1.getNeetMarks()); } else { mav.addObject("firstname", student.getName()); mav.addObject( "availability", "Not present in the database"); // mav.addObject("location", // student.getLocation()); } } // Catch block to handle SQL Exceptions catch (SQLException e) { // Displaying exception along with line number // using printStackTrace() method e.printStackTrace(); } return mav; } }
El conjunto anterior se puede preparar como un archivo war y se puede implementar en la carpeta tomcat webapps.
Al comienzo de Tomcat , la aplicación anterior se puede invocar usando
http://localhost:8080/SpringMVCMedicalSeatAvailabilityBasedOnNEETMarks/
Como esto se toma como un proyecto de muestra, no se hace mucho embellecimiento
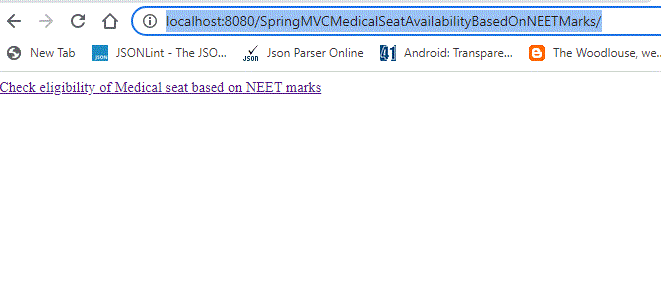
Podemos hacer clic en este enlace y avanzar
Al hacer clic en el enlace, obtendremos lo siguiente
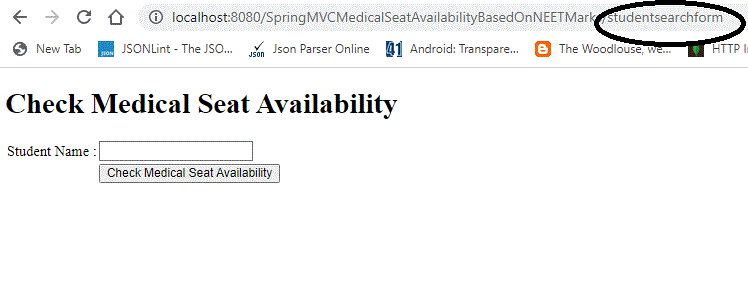
Compruebe el cambio de URL. Todo debe estar alineado con el controlador.
Caso de uso :
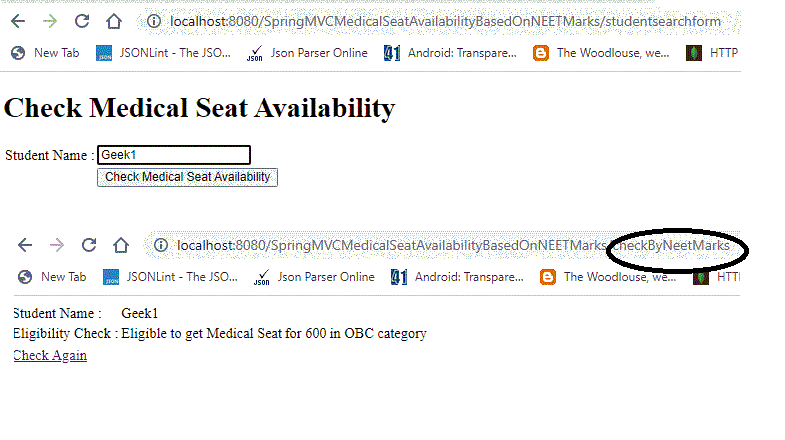
Según la lógica escrita, estamos obteniendo resultados, aquí
- “Geek1 ” es el nombre que se le da a la búsqueda. Se comparará con la tabla «studentsdetails»
- El círculo indica el nombre de la asignación de solicitud.
Es una aplicación de muestra y se dan los pasos necesarios en esta que interactúa con la base de datos MySQL. Usando Spring MVC y MySQL, podemos llevar a cabo fácilmente la lógica comercial.
Publicación traducida automáticamente
Artículo escrito por priyarajtt y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA