Tic-tac-toe (inglés americano), tres en raya (inglés británico) o Xs and Os es un juego de papel y lápiz para dos jugadores, X y O, que se turnan para marcar los espacios en una cuadrícula de 3×3. . El jugador que logra colocar tres de sus marcas en una fila horizontal, vertical o diagonal es el ganador.
La biblioteca tkinter Python se utiliza para crear la GUI. Hay dos opciones disponibles para jugar el juego, junto con el sistema o con otro jugador .
Se utiliza una pequeña estrategia ganadora para jugar con el sistema. El sistema intentará encontrar el mejor lugar para poner su cero o cruz por el cual el sistema ganará o intentará detener a los jugadores para ganar.
Acercarse:
- Cree una página de destino que contenga botones de selección: Un jugador o multijugador.
- Cree un tablero de juego que contenga nueve fichas para jugar junto con otros detalles (es decir, jugar con un sistema u otro jugador, cuyo turno, etc.).
- Permita que el jugador presione el mosaico y verifique el estado del juego (es decir, empate, cualquiera de los jugadores ganó el partido o el juego aún se está ejecutando).
- Muestra el mensaje, quién ganó el partido.
Descripción de otras funciones:
- gameboard_pc() y gameboard_pl() crearán otra geometría para jugar. Agregará 9 botones en el tablero de 3×3 del juego (Tres filas de botones que contienen tres botones cada una).
- Las funciones get_text_pc() y get_text() colocarán el texto en los botones a medida que se presionen.
- La función pc() decidirá el próximo movimiento del sistema.
- La función winner() verificará si el jugador ganó el partido o no.
- La función isfree() verificará si el jugador puede poner una moneda o no.
- La función isfull() verificará que el tablero esté lleno o no.
Ejemplo 1: GUI de Tic Tac Toe
Python
#importing Packages from tkinter from tkinter import * from tkinter import messagebox Player1 = 'X' stop_game = False def clicked(r,c): #player global Player1 # global stop_game if Player1 == "X" and states[r] == 0 and stop_game == False: b[r].configure(text = "X") states[r] = 'X' Player1='O' if Player1 == 'O' and states[r] == 0 and stop_game == False: b[r].configure(text = 'O') states[r] = "O" Player1 = "X" check_if_win() # check_if_tie() # if check_if_win() == False: # tie = messagebox.showinfo("tie","its tie") # return tie def check_if_win(): global stop_game # count = 0 for i in range(3): if states[i][0] == states[i][1] == states[i][2] !=0: stop_game = True winner = messagebox.showinfo("Winner", states[i][0] + " Won") # disableAllButton() break # for j in range(3): elif states [0][i] == states[1][i] == states[2][i] != 0: stop_game = True winner = messagebox.showinfo("Winner", states[0][i]+ " Won!") break elif states[0][0] == states[1][1] == states[2][2] !=0: stop_game = True winner = messagebox.showinfo("Winner", states[0][0]+ " Won!") break elif states[0][2] == states[1][1] == states[2][0] !=0: stop_game = True winner = messagebox.showinfo("Winner" , states[0][2]+ " Won!") break elif states[0][0] and states[0][1] and states[0][2] and states[1][0] and states[1][1] and states[1][2] and states[2][0] and states[2][1] and states[2][2] != 0: stop_game = True winner = messagebox.showinfo("tie", "Tie") # Design window #Creating the Canvas root = Tk() # Title of the window root.title("GeeksForGeeks-:Tic Tac Toe") root.resizable(0,0) #Button b = [ [0,0,0], [0,0,0], [0,0,0]] #text for buttons states = [ [0,0,0], [0,0,0], [0,0,0]] for i in range(3): for j in range(3): b[i][j] = Button( height = 4, width = 8, font = ("Helvetica","20"), command = lambda r = i, c = j : clicked(r,c)) b[i][j].grid(row = i, column = j) mainloop()
Producción:
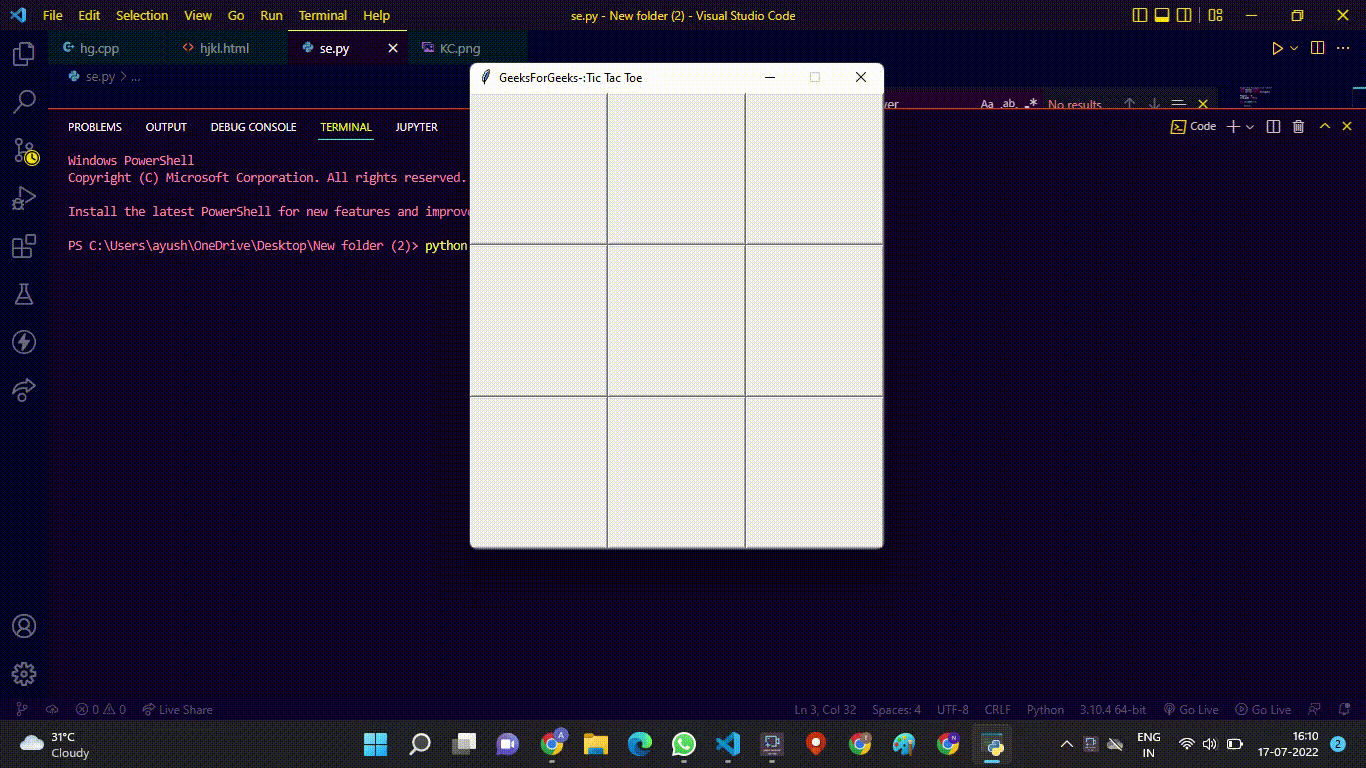
Ejemplo 2) GUI de Tic Tac Toe
Python3
# Tic Tac Toe game with GUI # using tkinter # importing all necessary libraries import random import tkinter from tkinter import * from functools import partial from tkinter import messagebox from copy import deepcopy # sign variable to decide the turn of which player sign = 0 # Creates an empty board global board board = [[" " for x in range(3)] for y in range(3)] # Check l(O/X) won the match or not # according to the rules of the game def winner(b, l): return ((b[0][0] == l and b[0][1] == l and b[0][2] == l) or (b[1][0] == l and b[1][1] == l and b[1][2] == l) or (b[2][0] == l and b[2][1] == l and b[2][2] == l) or (b[0][0] == l and b[1][0] == l and b[2][0] == l) or (b[0][1] == l and b[1][1] == l and b[2][1] == l) or (b[0][2] == l and b[1][2] == l and b[2][2] == l) or (b[0][0] == l and b[1][1] == l and b[2][2] == l) or (b[0][2] == l and b[1][1] == l and b[2][0] == l)) # Configure text on button while playing with another player def get_text(i, j, gb, l1, l2): global sign if board[i][j] == ' ': if sign % 2 == 0: l1.config(state=DISABLED) l2.config(state=ACTIVE) board[i][j] = "X" else: l2.config(state=DISABLED) l1.config(state=ACTIVE) board[i][j] = "O" sign += 1 button[i][j].config(text=board[i][j]) if winner(board, "X"): gb.destroy() box = messagebox.showinfo("Winner", "Player 1 won the match") elif winner(board, "O"): gb.destroy() box = messagebox.showinfo("Winner", "Player 2 won the match") elif(isfull()): gb.destroy() box = messagebox.showinfo("Tie Game", "Tie Game") # Check if the player can push the button or not def isfree(i, j): return board[i][j] == " " # Check the board is full or not def isfull(): flag = True for i in board: if(i.count(' ') > 0): flag = False return flag # Create the GUI of game board for play along with another player def gameboard_pl(game_board, l1, l2): global button button = [] for i in range(3): m = 3+i button.append(i) button[i] = [] for j in range(3): n = j button[i].append(j) get_t = partial(get_text, i, j, game_board, l1, l2) button[i][j] = Button( game_board, bd=5, command=get_t, height=4, width=8) button[i][j].grid(row=m, column=n) game_board.mainloop() # Decide the next move of system def pc(): possiblemove = [] for i in range(len(board)): for j in range(len(board[i])): if board[i][j] == ' ': possiblemove.append([i, j]) move = [] if possiblemove == []: return else: for let in ['O', 'X']: for i in possiblemove: boardcopy = deepcopy(board) boardcopy[i[0]][i[1]] = let if winner(boardcopy, let): return i corner = [] for i in possiblemove: if i in [[0, 0], [0, 2], [2, 0], [2, 2]]: corner.append(i) if len(corner) > 0: move = random.randint(0, len(corner)-1) return corner[move] edge = [] for i in possiblemove: if i in [[0, 1], [1, 0], [1, 2], [2, 1]]: edge.append(i) if len(edge) > 0: move = random.randint(0, len(edge)-1) return edge[move] # Configure text on button while playing with system def get_text_pc(i, j, gb, l1, l2): global sign if board[i][j] == ' ': if sign % 2 == 0: l1.config(state=DISABLED) l2.config(state=ACTIVE) board[i][j] = "X" else: button[i][j].config(state=ACTIVE) l2.config(state=DISABLED) l1.config(state=ACTIVE) board[i][j] = "O" sign += 1 button[i][j].config(text=board[i][j]) x = True if winner(board, "X"): gb.destroy() x = False box = messagebox.showinfo("Winner", "Player won the match") elif winner(board, "O"): gb.destroy() x = False box = messagebox.showinfo("Winner", "Computer won the match") elif(isfull()): gb.destroy() x = False box = messagebox.showinfo("Tie Game", "Tie Game") if(x): if sign % 2 != 0: move = pc() button[move[0]][move[1]].config(state=DISABLED) get_text_pc(move[0], move[1], gb, l1, l2) # Create the GUI of game board for play along with system def gameboard_pc(game_board, l1, l2): global button button = [] for i in range(3): m = 3+i button.append(i) button[i] = [] for j in range(3): n = j button[i].append(j) get_t = partial(get_text_pc, i, j, game_board, l1, l2) button[i][j] = Button( game_board, bd=5, command=get_t, height=4, width=8) button[i][j].grid(row=m, column=n) game_board.mainloop() # Initialize the game board to play with system def withpc(game_board): game_board.destroy() game_board = Tk() game_board.title("Tic Tac Toe") l1 = Button(game_board, text="Player : X", width=10) l1.grid(row=1, column=1) l2 = Button(game_board, text = "Computer : O", width = 10, state = DISABLED) l2.grid(row = 2, column = 1) gameboard_pc(game_board, l1, l2) # Initialize the game board to play with another player def withplayer(game_board): game_board.destroy() game_board = Tk() game_board.title("Tic Tac Toe") l1 = Button(game_board, text = "Player 1 : X", width = 10) l1.grid(row = 1, column = 1) l2 = Button(game_board, text = "Player 2 : O", width = 10, state = DISABLED) l2.grid(row = 2, column = 1) gameboard_pl(game_board, l1, l2) # main function def play(): menu = Tk() menu.geometry("250x250") menu.title("Tic Tac Toe") wpc = partial(withpc, menu) wpl = partial(withplayer, menu) head = Button(menu, text = "---Welcome to tic-tac-toe---", activeforeground = 'red', activebackground = "yellow", bg = "red", fg = "yellow", width = 500, font = 'summer', bd = 5) B1 = Button(menu, text = "Single Player", command = wpc, activeforeground = 'red', activebackground = "yellow", bg = "red", fg = "yellow", width = 500, font = 'summer', bd = 5) B2 = Button(menu, text = "Multi Player", command = wpl, activeforeground = 'red', activebackground = "yellow", bg = "red", fg = "yellow", width = 500, font = 'summer', bd = 5) B3 = Button(menu, text = "Exit", command = menu.quit, activeforeground = 'red', activebackground = "yellow", bg = "red", fg = "yellow", width = 500, font = 'summer', bd = 5) head.pack(side = 'top') B1.pack(side = 'top') B2.pack(side = 'top') B3.pack(side = 'top') menu.mainloop() # Call main function if __name__ == '__main__': play()
Producción: