La herencia es un pilar importante de OOP (Programación Orientada a Objetos). Es el mecanismo en java por el cual una clase puede heredar las características (campos y métodos) de otra clase. En este artículo, crearemos una aplicación para calcular el interés de FD, RD en función de ciertas condiciones mediante la herencia. Cree tres clases en un solo paquete. Cree su función miembro. Cree un programa dirigido por menús.
Pasos
- Cree el archivo principal de Java para ingresar las opciones en la Calculadora de intereses, como cuenta de ahorro, cuenta de depósito fijo, depósitos recurrentes.
- Crear Otra Clase para calcular el Interés del depósito a plazo fijo. Calcule el interés de FD por fórmula = FDAmount * FDinterestRate.
- Además, verifique si el interés es aplicable para un ciudadano normal de la tercera edad. Crear Otra Clase para calcular el depósito de Intereses de Ahorro. Calcule el interés de la cuenta de ahorro por Fórmula = Cantidad * Tasa de interés de la cuenta de ahorro . Además, verifique si es una Cuenta Normal o una Cuenta NRI.
- Crear Otra Clase para calcular el Interés de los Depósitos Recurrentes. Calcule el interés de RD por fórmula = RDAmount * RDinterestRate.
- Además, Verifique los Meses y la edad del titular para Calcular el Interés.
Diagrama de flujo
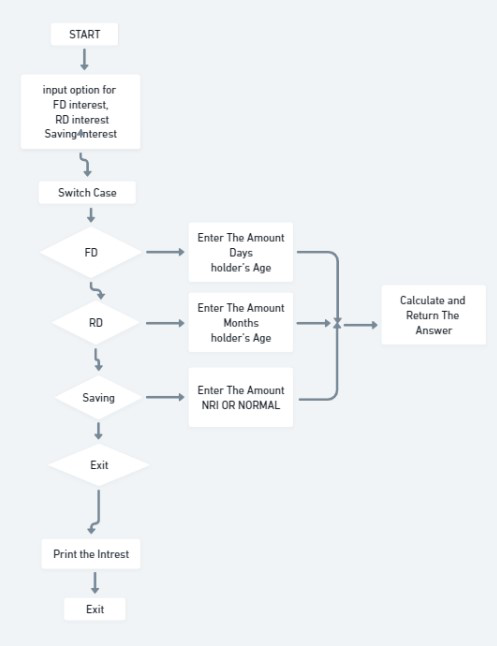
Código
Cuenta.Java
Java
package College; public abstract class Account { double interestRate; double amount; abstract double calculateInterest(double amount) throws InvalidMonthsException, InvalidAgeException, InvalidAmountException, InvalidDaysException; }
Calculadora de intereses.java
Java
package College; import java.util.Scanner; public class InterestCalculator { public static void main(String[] args) { // TODO code application logic here Scanner sc = new Scanner(System.in); System.out.println( "SELECT THE OPTIONS " + "\n1." + " Interest Calculator-Saving Account " + " \n2." + " Interest Calculator - Fixed Deposit " + "\n3." + " InterestCalculator-Recurring Deposits" + "\n4 " + " Exit"); int choice = sc.nextInt(); switch (choice) { case 1: SBaccount sb = new SBaccount(); try { System.out.println( "Enter the Average SB amount "); double amount = sc.nextDouble(); System.out.println( "Interest gained is : $ " + sb.calculateInterest(amount)); } catch (InvalidAmountException e) { System.out.println( "Exception : Invalid amount"); } break; case 2: try { FDaccount fd = new FDaccount(); System.out.println("Enter the FD Amount"); double fAmount = sc.nextDouble(); System.out.println( "Interest gained is: $ " + fd.calculateInterest(fAmount)); } catch (InvalidAgeException e) { System.out.println("Invalid Age Entered"); } catch (InvalidAmountException e) { System.out.println( "Invalid Amount Entered"); } catch (InvalidDaysException e) { System.out.println("Invalid Days Entered"); } break; case 3: try { RDaccount rd = new RDaccount(); System.out.println("Enter the RD amount"); double Ramount = sc.nextDouble(); System.out.println( "Interest gained is: $ " + rd.calculateInterest(Ramount)); } catch (InvalidAgeException e) { System.out.println("Invalid Age Entered"); } catch (InvalidAmountException e) { System.out.println( "Invalid Amount Entered"); } catch (InvalidMonthsException e) { System.out.println("Invalid Days Entered"); } break; case 4: System.out.println( "DO YOU WANT TO CALCULATE AGAIN ????" + " " + "RUN AGAIN THE PROGRAM"); default: System.out.println("Wrong choice"); } } }
FDACuenta.java
Java
package College; import java.util.Scanner; public class FDaccount extends Account { double FDinterestRate; double FDAmount; int noOfDays; int ageOfACHolder; double General, SCitizen; Scanner FDScanner = new Scanner(System.in); @Override double calculateInterest(double amount) throws InvalidAgeException, InvalidAmountException, InvalidDaysException { this.FDAmount = amount; System.out.println("Enter FD days"); noOfDays = FDScanner.nextInt(); System.out.println("Enter FD age holder "); ageOfACHolder = FDScanner.nextInt(); if (amount < 0) { throw new InvalidAmountException(); } if (noOfDays < 0) { throw new InvalidDaysException(); } if (ageOfACHolder < 0) { throw new InvalidAgeException(); } if (amount < 10000000) { if (noOfDays >= 7 && noOfDays <= 14) { General = 0.0450; SCitizen = 0.0500; } else if (noOfDays >= 15 && noOfDays <= 29) { General = 0.0470; SCitizen = 0.0525; } else if (noOfDays >= 30 && noOfDays <= 45) { General = 0.0550; SCitizen = 0.0600; } else if (noOfDays >= 45 && noOfDays <= 60) { General = 0.0700; SCitizen = 0.0750; } else if (noOfDays >= 61 && noOfDays <= 184) { General = 0.0750; SCitizen = 0.0800; } else if (noOfDays >= 185 && noOfDays <= 365) { General = 0.0800; SCitizen = 0.0850; } FDinterestRate = (ageOfACHolder < 50) ? General : SCitizen; } else { if (noOfDays >= 7 && noOfDays <= 14) { interestRate = 0.065; } else if (noOfDays >= 15 && noOfDays <= 29) { interestRate = 0.0675; } else if (noOfDays >= 30 && noOfDays <= 45) { interestRate = 0.00675; } else if (noOfDays >= 45 && noOfDays <= 60) { interestRate = 0.080; } else if (noOfDays >= 61 && noOfDays <= 184) { interestRate = 0.0850; } else if (noOfDays >= 185 && noOfDays <= 365) { interestRate = 0.10; } } return FDAmount * FDinterestRate; } }
RDaccount.java
Java
package College; import java.util.Scanner; public class RDaccount extends Account { double RDInterestRate; double RDamount; int noOfMonths; double monthlyAmount; double General, SCitizen; Scanner RDScanner = new Scanner(System.in); @Override double calculateInterest(double Ramount) throws InvalidMonthsException, InvalidAmountException, InvalidAgeException { this.RDamount = Ramount; System.out.println("Enter RD months"); noOfMonths = RDScanner.nextInt(); System.out.println("Enter RD holder age"); int age = RDScanner.nextInt(); if (RDamount < 0) { throw new InvalidAmountException(); } if (noOfMonths < 0) { throw new InvalidMonthsException(); } if (age < 0) { throw new InvalidAgeException(); } if (noOfMonths >= 0 && noOfMonths <= 6) { General = .0750; SCitizen = 0.080; } else if (noOfMonths >= 7 && noOfMonths <= 9) { General = .0775; SCitizen = 0.0825; } else if (noOfMonths >= 10 && noOfMonths <= 12) { General = .0800; SCitizen = 0.0850; } else if (noOfMonths >= 13 && noOfMonths <= 15) { General = .0825; SCitizen = 0.0875; } else if (noOfMonths >= 16 && noOfMonths <= 18) { General = .0850; SCitizen = 0.0900; } else if (noOfMonths >= 22) { General = .0875; SCitizen = 0.0925; } RDInterestRate = (age < 50) ? General : SCitizen; return RDamount * RDInterestRate; } }
SBaccount.Java
Java
package College; import java.util.Scanner; class SBaccount extends Account { double SBamount, SbInterestRate, interest; Scanner SBScanner = new Scanner(System.in); @Override double calculateInterest(double amount) throws InvalidAmountException { this.SBamount = amount; if (SBamount < 0) { throw new InvalidAmountException(); } System.out.println( "Select account type \n1. NRI \n2. Normal "); int accountChoice = SBScanner.nextInt(); switch (accountChoice) { case 1: SbInterestRate = .06; break; case 2: SbInterestRate = .04; break; default: System.out.println( "Please choose right account again"); } return amount * SbInterestRate; } }
Producción
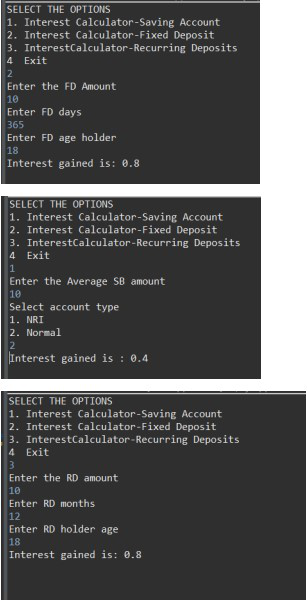
Publicación traducida automáticamente
Artículo escrito por dragonuncaged y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA