Un conjunto es una colección de elementos únicos. Por único, queremos decir que no hay dos elementos en un conjunto que puedan ser iguales. A diferencia de los conjuntos en C++, los elementos de un conjunto en Swift no se organizan en ningún orden en particular. Internamente, un conjunto en Swift usa una tabla hash para almacenar elementos en el conjunto. Consideremos un ejemplo, queremos verificar el número de notas únicas o diferentes obtenidas por los estudiantes en una clase. Se puede utilizar un conjunto para realizar esta tarea. Sea una lista que contenga marcas, lista = [98, 80, 86, 80, 98, 98, 67, 90, 67, 84]. En la lista, 98 ocurre tres veces, 80 y 67 ocurren dos veces mientras que 84, 86 y 90 ocurren solo una vez. Entonces, hay un total de seis marcas únicas obtenidas por los estudiantes en una clase. Podemos declarar un conjunto de enteros e insertar estos valores en el conjunto. El tamaño del conjunto determina el número de marcas únicas obtenidas por los estudiantes.
Creación de conjuntos
Podemos crear un conjunto vacío usando la siguiente sintaxis. Este método espera explícitamente un tipo de datos del conjunto. Por ejemplo, si queremos almacenar valores Int en el conjunto, podemos pasar Int entre paréntesis angulares (<Int>). Del mismo modo, podemos pasar las palabras clave Float, Character, String, Double, Bool al corchete angular, etc.
Sintaxis:
var mySet = Establecer<tipo_datos>()
Aquí, data_type es el tipo de datos almacenados por el conjunto (Int, Character, String, etc.). A continuación se muestra el programa para ilustrar cómo podemos crear un conjunto vacío.
Ejemplo:
Swift
// Swift program to illustrate how we can create // an empty set of Int, Float, Double, Character // and String type import Foundation import Glibc // Creating an empty set of Int data type var mySet1 = Set<Int>() // Inserting elements in mySet1 mySet1.insert(10) mySet1.insert(20) mySet1.insert(10) print("mySet1:", mySet1) // Creating an empty set of Float data type var mySet2 = Set<Float>() // Inserting elements in mySet2 mySet2.insert(10.123) mySet2.insert(20.123) print("mySet2:", mySet2) // Creating an empty set of Double data type var mySet3 = Set<Double>() // Inserting elements in mySet3 mySet3.insert(10.123456) mySet3.insert(20.123456) print("mySet3:", mySet3) // Creating an empty set of Character data type var mySet4 = Set<Character>() // Inserting elements in mySet4 mySet4.insert("S") mySet4.insert("C") print("mySet4:", mySet4) // Creating an empty set of String data type var mySet5 = Set<String>() // Inserting elements in mySet5 mySet5.insert("GeeksfoGeeks") mySet5.insert("Geeks") print("mySet5:", mySet5) // Creating a empty Set of Boolean type var mySet6 = Set<Bool>() // Inserting elements in mySet6 mySet6.insert(true) mySet6.insert(false) print("mySet6:", mySet6)
Producción:
mySet1: [20, 10] mySet2: [20.123, 10.123] mySet3: [20.123456, 10.123456] mySet4: ["H", "i"] mySet5: ["Geeks", "GeeksfoGeeks"] mySet6: [true, false]
Tenga en cuenta que hemos insertado 10 dos veces en mySet1 pero internamente se almacenó solo una vez en mySet1.
Inicialización de un Conjunto
Inicializar un conjunto básicamente significa que queremos almacenar algún valor en un conjunto en el momento de la declaración. Podemos inicializar un conjunto usando los siguientes métodos:
Método 1: este método de inicialización no requiere pasar explícitamente un tipo de datos al conjunto. El compilador determina implícitamente el tipo de datos en función de los valores proporcionados a un conjunto.
Sintaxis:
var mySet : Conjunto = [valor1, valor2, valor3,…….]
Aquí, mySet es el Set declarado, Set es la palabra clave utilizada para especificar que mySet es un Set, y value1,value2, value,… son los elementos que pertenecen a mySet. A continuación se muestra la implementación para ilustrar cómo podemos inicializar un conjunto.
Ejemplo:
Swift
// Swift program to illustrate how we can // initialize a set of Int, Float, Character, // String // Initializing a set of integers var mySet1: Set = [ 1, 1, 2, 3, 5 ] // Initializing a set of floats var mySet2: Set = [ 1.123, 2.123, 3.123, 4.123, 5.123 ] // Initializing a set of strings var mySet3: Set = [ "Geeks", "for", "Geeks" ] // Initializing a set of characters var mySet4: Set = [ "G", "e", "e", "k", "s" ] // Print the mySet1 print("mySet1: \(mySet1)") // Print the mySet2 print("mySet2: \(mySet2)") // Print the mySet3 print("mySet3: \(mySet3)") // Print the mySet4 print("mySet4: \(mySet4)")
Producción:
mySet1: [3, 2, 5, 1] mySet2: [2.123, 3.123, 5.123, 1.123, 4.123] mySet3: ["for", "Geeks"] mySet4: ["e", "G", "k", "s"]
Método 2: este método de inicialización requiere pasar explícitamente un tipo de datos al conjunto entre paréntesis angulares <>. Por ejemplo, si queremos almacenar elementos de tipo de datos Int en un conjunto, podemos usar <Int>. Asimismo, podemos pasar otros tipos de datos como Float, Double, Character, String, Bool, etc.
var mySet : Set<tipo_de_datos> = [valor1, valor2, valor3,…….]
Aquí, mySet es el Set declarado, data_type especifica explícitamente que mySet puede almacenar elementos de un tipo de datos particular, Set es una palabra clave que especifica que mySet es un Set y value1, value2, value,… son los elementos que pertenecen a mySet. A continuación se muestra la implementación para ilustrar cómo podemos inicializar un conjunto.
Ejemplo:
Swift
// Swift program to illustrate how to initialize a set by // explicitly specifying the data-type of the set // Initializing a set of integers // by explicitly passing the data type of the set var mySet1: Set<Int> = [ 10, 20, 20, 30, 50, 100, 20 ] // Initializing a set of floats // by explicitly passing the data type of the set var mySet2: Set<Float> = [ 1.123, 2.123, 3.123, 4.123, 5.123, 6.123, 7.123 ] // Initializing a set of doubles // by explicitly passing the data type of the set var mySet3: Set<Double> = [ 1.1234567, 2.1234567, 3.1234567, 4.1234567, 5.1234567, 6.1234567, 7.1234567 ] // Initializing a set of characters // by explicitly passing the data type of the set var mySet4: Set<Character> = ["G", "e", "e", "k", "s"] // Initializing a set of strings // by explicitly passing the data type of the set var mySet5: Set<String> = [ "GeeksforGeeks", "Java", "Python", "Swift", "Php" ] print("mySet1 elements are: ") // Print mySet1 elements for element in mySet1{ print(element) } print("\n") print("mySet2 elements are: ") // Print mySet2 elements for element in mySet2{ print(element) } print("\n") print("mySet3 elements are: ") // Print mySet3 elements for element in mySet3{ print(element) } print("\n") print("mySet4 elements are: ") // Print mySet4 elements for element in mySet4{ print(element) } print("\n") // Print mySet5 elements print("mySet5 elements are: ") // Print mySet5 elements for element in mySet5{ print(element) }
Producción:
mySet1 elements are: 20 50 100 10 30 mySet2 elements are: 5.123 6.123 3.123 2.123 1.123 4.123 7.123 mySet3 elements are: 3.1234567 1.1234567 4.1234567 6.1234567 5.1234567 2.1234567 7.1234567 mySet4 elements are: k s e G mySet5 elements are: GeeksforGeeks Java Python Swift Php
Método 3: Swift proporciona un método incorporado llamado método insert() que se usa para agregar elementos a un Conjunto. Toma O(1) o tiempo constante.
Sintaxis:
miConjunto.insertar(x)
Ejemplo:
Swift
// Swift program to illustrate how to add elements in set // Creating an empty set of Int data type var mySet = Set<String>() // Inserting elements in mySet1 mySet.insert("GeeksforGeeks") mySet.insert("GFG") mySet.insert("Geeks") print("Elements of mySet are :") for element in mySet{ // Print a Set element print(element) }
Producción:
Elements of mySet are : GeeksforGeeks Geeks GFG
Iterando sobre un conjunto
1. Usando el bucle for-in: Para iterar sobre un conjunto podemos tomar la ayuda del bucle for-in. Apunta directamente a los valores especificados en un conjunto.
Sintaxis:
para elemento en mySet {
// cuerpo
}
Aquí, mySet es el conjunto inicializado y el elemento es un elemento de mySet.
Ejemplo:
Swift
// Swift program to illustrate how we can iterate // over a set using for-in loop // Initializing a Set of integers var mySet1 : Set = [ 1, 21, 13, 4, 15, 6 ] // Iterating over mySet1 using // for loop print("mySet1 elements are :") for element in mySet1{ // Print a Set element print(element) } // Next line print("\n") // Initializing a Set of String var mySet2 : Set = [ "Bhuwanesh", "Kunal", "Aarush", "Honey", "Nawal" ] // Iterating over mySet2 using // for loop print("mySet2 elements are :") for element in mySet2{ // Print a Set element print(element) }
Producción:
mySet1 elements are : 15 21 1 4 13 6 mySet2 elements are : Kunal Honey Bhuwanesh Aarush Nawal
2. Usar el método de instancia index (:offsetBy:): Swift proporciona un método de instancia mediante el cual podemos acceder a los elementos del conjunto. Este método es equivalente a acceder a elementos del conjunto usando índices. Podemos iterar sobre los índices usando un bucle while e imprimir el valor presente en el índice especificado.
Sintaxis:
func index(mySet.startIndex, offsetBy: index)
Parámetros: mySet.startIndex es el índice inicial del conjunto y el índice es un número entero no negativo.
Valor devuelto : Devuelve un valor especificado en la posición, índice
A continuación se muestra la implementación para ilustrar el método de instancia de desplazamiento para iterar sobre un conjunto.
Ejemplo:
Swift
// Swift program to illustrate the working of // offset method to iterate over a set // Initializing a set of integers var mySet1: Set = [ 10, 3, 4, 8, 9 ] // Initializing a variable that will // act as an Index var Index = 0 print("mySet1 elements: ") // Iterating over indices // Using while loop while Index < mySet1.count{ // Printing elements using offset method print(mySet1[mySet1.index(mySet1.startIndex, offsetBy: Index)]) Index = Index + 1 } print("\n") // Initializing a set of floats var mySet2: Set = [ 1.123, 3.123, 4.123, 8.123, 9.123 ] // Initializing a variable that will // act as an Index Index = 0 print("mySet2 elements: ") // Iterating over indices // Using while loop while Index < mySet2.count{ // Printing elements using offset method print(mySet2[mySet2.index(mySet2.startIndex, offsetBy: Index)]) Index = Index + 1 }
Producción:
mySet1 elements: 10 4 9 3 8 mySet2 elements: 3.123 1.123 9.123 4.123 8.123
Comparación de conjuntos
Comparar dos conjuntos significa que queremos verificar si contienen elementos similares o no. Por ejemplo, mySet1 = [9, 1, 3, 10, 12] y mySet2 = [1, 3, 10, 12, 9] son conjuntos iguales ya que contienen los mismos elementos, mientras que mySet1 = [1, 2, 5, 4, 11] y mySet2 = [1, 2, 5, 4, 3, 11] son diferentes entre sí ya que 2 está presente en mySet2 pero no está presente en mySet1. Podemos comparar dos conjuntos usando los siguientes métodos:
1. Usando el método de contener(): podemos comparar dos conjuntos definiendo un método de comparación personalizado. Siga el enfoque a continuación para comprender cómo comparar dos conjuntos:
Acercarse:
1. Defina una función global, Compare. El tipo de retorno de este método sería bool ya que queremos saber si dos conjuntos son iguales o no. Este método acepta dos conjuntos, mySet1 y mySet2 como parámetros.
2. Iterar sobre mySet1 usando el bucle for-in. Para cada elemento en mySet1 , verifique si está presente en mySet2 usando el método contains(). Si un elemento está presente en mySet1 pero no está presente en mySet2 , devuelve falso.
3. Ahora itere sobre mySet2 usando el bucle for-in. Para cada elemento en mySet2 , verifique si está presente en mySet1 usando el método contains(). Si un elemento está presente en mySet2 pero no está presente en mySet1 , devuelve falso.
4. Eventualmente, devuelva verdadero desde la función Comparar .
A continuación se muestra la implementación para ilustrar el enfoque anterior:
Ejemplo:
Swift
// Swift program to illustrate how we can compare // two set using a custom function // Defining a custom function which accepts two // sets as a parameters func Compare(mySet1: Set<Int>, mySet2: Set<Int>) -> Bool { // Iterate over each element of mySet1 for element in mySet1 { // If there is an element which is present // in mySet1 but not present in mySet2 // return false from the Compare function if (!mySet2.contains(element)) { return false } } for element in mySet2 { // If there is an element which is present // in mySet2 but not present in mySet1 // return false if (!mySet1.contains(element)) { return false } } // Since all elements are common // return true from the Compare function return true } // Initializing a Set of Int type var mySet1 : Set = [ 1, 3, 4, 7, 10, 12 ] // Initializing another Set of Int type var mySet2 : Set = [ 10, 7, 4, 3, 1, 2] // Initializing a Set of Int type var mySet3 : Set = [ 1, 3, 4, 7, 10, 2 ] // Calling compare method to compare mySet1 and mySet2 print("Are myset1 and myset2 equal ? \(Compare(mySet1: mySet1, mySet2: mySet2))" ) // Calling compare method to compare mySet1 and mySet3 print("Are myset1 and myset3 equal ? \(Compare(mySet1: mySet1, mySet2: mySet3))" ) // Calling compare method to compare mySet2 and mySet3 print("Are myset2 and myset3 equal ? \(Compare(mySet1: mySet2, mySet2: mySet3))" )
Producción:
Are myset1 and myset2 equal ? false Are myset1 and myset3 equal ? false Are myset2 and myset3 equal ? true
2. Usar el operador de comparación ( == ): Swift proporciona el operador de comparación “==” para comparar dos conjuntos directamente. Internamente, comprueba si los conjuntos contienen los mismos elementos. Si ambos conjuntos tienen elementos similares, devuelve verdadero; de lo contrario, devuelve falso. Tenga en cuenta que los conjuntos de tipo mySet1 = [5, 1, 7, 8] y mySet2 = [1, 8, 7, 5] se consideran iguales ya que no hay un orden fijo en el que los elementos del conjunto se almacenan en un conjunto. y sólo nos preocupa si contienen elementos similares.
Sintaxis:
miConjunto1 == miConjunto2
Aquí, mySet1 y mySet2 son los conjuntos que queremos comparar
Tipo de retorno:
- Verdadero: si ambos conjuntos contienen los mismos elementos
- Falso: si hay un elemento que está presente en un conjunto y no está presente en otro conjunto o viceversa.
A continuación se muestra el programa para ilustrar cómo podemos utilizar un operador de comparación para comparar dos conjuntos en Swift.
Ejemplo:
Swift
// Swift program to illustrate how we can compare // two sets using comparison operator // Initializing a set var mySet1: Set = [ 20.123, 50.123, 30.123, 10.123, 40.123 ] // Initializing another set var mySet2: Set = [ 10.123, 20.123, 30.123, 40.123, 50.123 ] // Initializing a set var mySet3: Set = [ 10.123, 20.123, 30.123, 40.123, 50.123, 60.123 ] // Check whether mySet1 and mySet2 are equal print("Are myset1 and myset2 equal ? \(mySet1 == mySet2)") // Check whether mySet1 and mySet3 are equal print("Are myset1 and myset3 equal ? \(mySet1 == mySet3)") // Check whether mySet2 and mySet3 are equal print("Are myset2 and myset2 equal ? \(mySet2 == mySet3)")
Producción:
Are myset1 and myset2 equal ? true Are myset1 and myset3 equal ? false Are myset2 and myset2 equal ? false
Establecer operaciones
Podemos realizar una serie de operaciones básicas de conjuntos que son unión, intersección, resta. diferencia, etc utilizando los métodos incorporados proporcionados en Swift.
1. Unión: La Unión de dos conjuntos es un conjunto formado por la combinación de los elementos de dos conjuntos. Por ejemplo, considere dos conjuntos, mySet1 = {2, 10, 1, 4} y mySet2 = {10, 3, 12}. Entonces el conjunto unión sería myUnion = {2, 10, 1, 4, 3, 12}. En Swift, podemos lograr una unión de dos conjuntos con la ayuda del método union() incorporado que se usa con los conjuntos. Este método requiere dos conjuntos y crea un nuevo conjunto que contiene la unión de los conjuntos especificados.
Sintaxis:
miConjunto1.union(miConjunto2)
Aquí, mySet1 y mySet2 son conjuntos inicializados
Tipo de retorno: Devuelve un conjunto que tiene elementos que pertenecen a un conjunto, a otro conjunto o a ambos.
Esta operación toma un tiempo O(n), donde n es la longitud de la secuencia más larga.
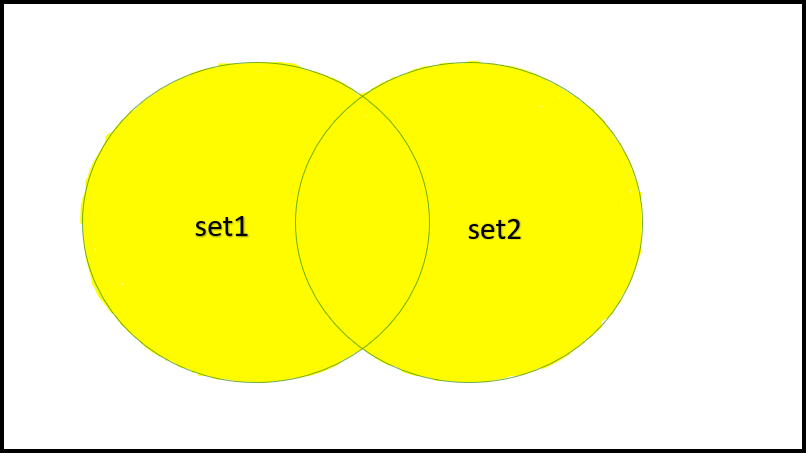
Ejemplo:
Swift
// Swift program to illustrate the working of Union operation // Initializing a set containing multiples of 3 var mySet1: Set = [ 3, 6, 9, 12, 15, 18, 21, 24, 27, 30 ] // Initializing a set containing multiple of 5 var mySet2: Set = [ 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 ] // Using inbuilt union method to produce the union // of sets containing multiples of 3 and 5 var unionOfSets = mySet1.union(mySet2).sorted() print("Union set: \(unionOfSets)")
Producción:
Union set: [3, 5, 6, 9, 10, 12, 15, 18, 20, 21, 24, 25, 27, 30, 35, 40, 45, 50]
2. Intersección: La intersección de dos conjuntos es un conjunto que tiene solo los elementos comunes a ambos conjuntos. Por ejemplo, considere dos conjuntos, mySet1 = {2, 10, 1, 4} y mySet2 = {10, 3, 12}. Entonces, el conjunto de intersección sería myIntersection = {10}. En Swift, podemos lograr una intersección de dos conjuntos con la ayuda del método de intersection() incorporado que se usa con los conjuntos. Este método requiere dos conjuntos y crea un nuevo conjunto que contiene la intersección de los conjuntos especificados.
Sintaxis:
miConjunto1.intersección(miConjunto2)
Aquí, mySet1 y mySet2 son conjuntos inicializados
Tipo de retorno: Devuelve un conjunto que tiene elementos que son comunes a ambos conjuntos
Esta operación toma un tiempo O(n), donde n es la longitud de la secuencia más larga.
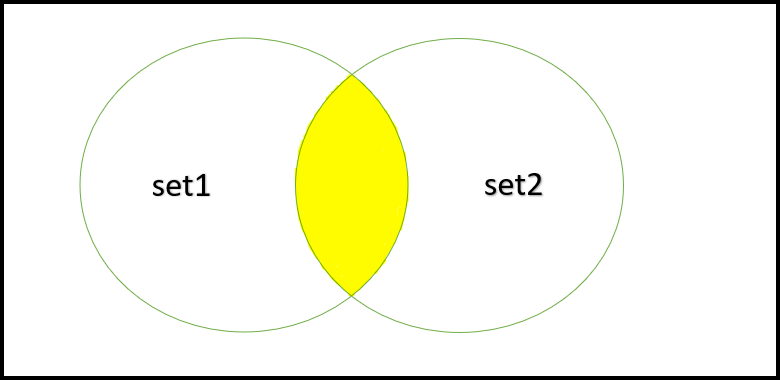
Ejemplo:
Swift
// Swift program to illustrate the working of Intersection set operation // Initializing a set containing multiples of 3 var mySet1: Set = [ 3, 6, 9, 12, 15, 18, 21, 24, 27, 30 ] // Initializing a set containing multiple of 5 var mySet2: Set = [ 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 ] // Using inbuilt intersection method to produce the // union of sets containing multiples of 3 and 5 var intersectionOfSets = mySet1.intersection(mySet2).sorted() print("Intersection set: \(intersectionOfSets)")
Producción:
Intersection set: [15, 30]
3. Resta: La resta de dos conjuntos de la forma “conjunto1 – conjunto2” es un conjunto que tiene solo los elementos que están presentes en el conjunto1 pero no están presentes en el conjunto2. Por ejemplo, considere dos conjuntos, mySet1 = {2, 10, 1, 4} y mySet2 = {10, 3, 12}. Entonces el conjunto de resta sería mySubtraction = {2, 1, 4}. En Swift, podemos lograr una resta de dos conjuntos con la ayuda del método de subtracting() incorporado que se usa con los conjuntos. Este método requiere dos conjuntos y crea un nuevo conjunto que contiene la resta de conjuntos específicos.
Sintaxis:
miConjunto1.restando(miConjunto2)
Aquí, mySet1 y mySet2 son conjuntos inicializados
Tipo de retorno: Devuelve un conjunto que tiene elementos que están presentes en un conjunto (mySet1) pero no están presentes en otro conjunto (mySet2).
Esta operación toma un tiempo O(n), donde n es la longitud de la secuencia más larga.
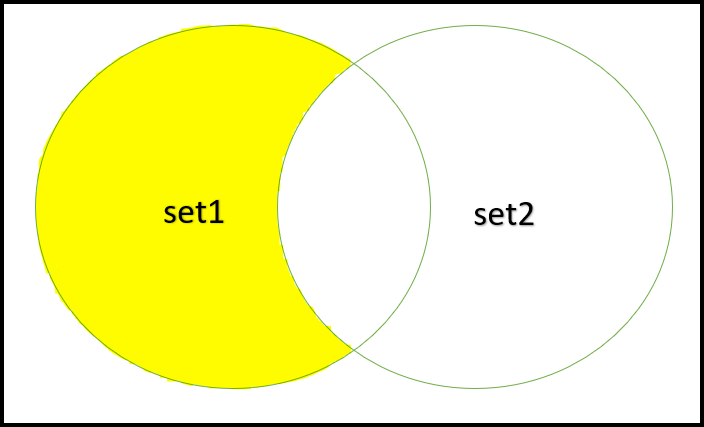
Ejemplo:
Swift
// Swift program to illustrate the working of // subtraction set operation // Initializing a set containing multiples of 3 var mySet1: Set = [ 3, 6, 9, 12, 15, 18, 21, 24, 27, 30 ] // Initializing a set containing multiple of 5 var mySet2: Set = [ 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 ] // Using inbuilt subtracting method to produce the // subtraction of sets containing multiples of 3 and 5 var subtractionOfSets = mySet1.subtracting(mySet2).sorted() print("Subtraction set: \(subtractionOfSets)")
Producción:
Subtraction set: [3, 6, 9, 12, 18, 21, 24, 27]
4. Diferencia simétrica: La diferencia simétrica de dos conjuntos A y B es un conjunto que tiene todos los elementos de ambos conjuntos excepto los elementos comunes. Por ejemplo, considere dos conjuntos, mySet1 = {2, 10, 1, 4} y mySet2 = {10, 3, 12}. Entonces, la diferencia simétrica sería mySymmetricDifference = {2, 1, 4}. En Swift, podemos lograr una diferencia simétrica de dos conjuntos con la ayuda del método symmetricDifference() incorporado que se usa con los conjuntos. Este método requiere dos conjuntos y crea un nuevo conjunto que contiene la diferencia simétrica de los conjuntos especificados.
Sintaxis:
miConjunto1.DiferenciaSimétrica(miConjunto2)
Aquí, mySet1 y mySet2 son conjuntos inicializados
Tipo de retorno: Devuelve un conjunto que tiene todos los elementos de ambos conjuntos excepto los elementos comunes.
Esta operación toma un tiempo O(n), donde n es la longitud de la secuencia más larga.
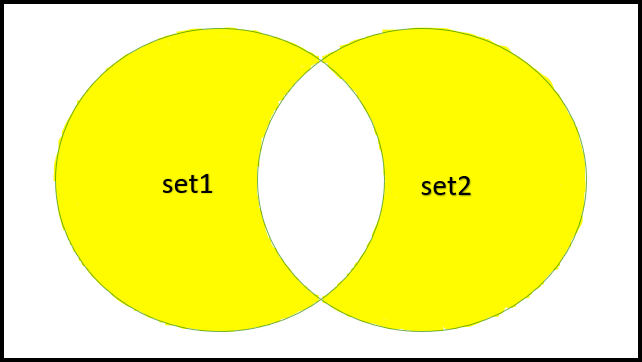
Ejemplo:
Swift
// Swift program to illustrate the working of // Symmetric Difference set operation // Initializing a set containing multiples of 3 var mySet1: Set = [ 3, 6, 9, 12, 15, 18, 21, 24, 27, 30 ] // Initializing a set containing multiple of 5 var mySet2: Set = [ 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 ] // Using inbuilt symmetric difference method to // produce the symmetric difference of sets // containing multiples of 3 and 5 var symmetricDifferenceOfSets = mySet1.symmetricDifference(mySet2).sorted() print("Symmetric difference set: \(symmetricDifferenceOfSets)")
Producción:
Symmetric difference set: [3, 5, 6, 9, 10, 12, 18, 20, 21, 24, 25, 27, 35, 40, 45, 50]
5. Subconjunto: Un conjunto es un subconjunto de otro conjunto si todos los elementos del primer conjunto son elementos del segundo conjunto. Por ejemplo, mySet1 = {1, 2, 4, 3} es un subconjunto de mySet2 = {5, 3 , 1, 2, 4}. En Swift, podemos verificar si un conjunto es un subconjunto de otro conjunto con la ayuda del método isSubset() incorporado que se usa con los conjuntos.
Sintaxis:
mySet1.isSubset(de: mySet2)
Aquí, mySet1 y mySet2 son conjuntos inicializados
Tipo de retorno:
- verdadero: si un conjunto es un subconjunto de otro conjunto
- falso: si un conjunto no es un subconjunto de otro conjunto
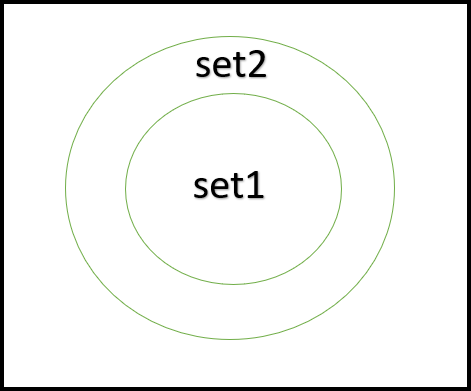
A continuación se muestra el programa para ilustrar estas operaciones de conjuntos:
Ejemplo:
Swift
// Swift program to illustrate the working of Subset // Initializing a set containing multiples of 3 var mySet1: Set = [ 3, 6, 9, 12, 15, 18, 21, 24, 27, 30 ] // Initializing a set containing multiple of 5 var mySet2: Set = [ 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 ] // Check mySet1 is a subset mySet2 or not print("Is mySet1 a subset of mySet2 ?: \(mySet1.isSubset(of: mySet2))")
Producción:
Is mySet1 a subset of mySet2 ?: false
Modificación de conjuntos
Para modificar un conjunto, Swift proporciona una serie de métodos asociados con un conjunto y son:
1. remove(x): Esta función se utiliza para eliminar un elemento ‘ x ‘ de un Conjunto. Si el elemento aún no está presente en el Conjunto, incluso entonces el programa se compila con éxito. Esta es una característica única en Swift y es por eso que un conjunto en Swift a veces es más ventajoso que un conjunto en otros lenguajes como C++.
Sintaxis:
miConjunto.remove(x)
2. removeFirst(): este método se utiliza para eliminar el primer elemento de un conjunto. Dado que los elementos del conjunto no están dispuestos en ningún orden en particular en un conjunto en Swift, es por eso que podemos predecir de antemano qué elemento se eliminará con este método. La complejidad temporal de este método es constante amortizada, O(1).
Sintaxis:
miConjunto.removeFirst(x)
3. removeAll(): esta función se usa para eliminar todos los elementos de un conjunto. Toma O(n) tiempo donde n es el tamaño del conjunto.
Sintaxis:
miConjunto.removeAll()
4. contiene (x): esta función se utiliza para verificar si el elemento x está presente en el conjunto o no. Toma O(1) tiempo por operación. Devolverá verdadero si x está presente en el conjunto, de lo contrario, devolverá falso si x no está presente en el conjunto.
Sintaxis:
miConjunto.contiene(x)
5. contar: se utiliza para encontrar el número de elementos presentes en un conjunto. Toma O(1) tiempo por operación. Por ejemplo, se nos da un conjunto, mySet = {1, 4, 5, 7, 5}. El recuento de mySet sería igual a 4. Siempre devolverá un número entero no negativo.
Sintaxis:
mySet.count
6. isEmpty: devuelve verdadero si un conjunto está vacío; de lo contrario, devuelve falso. Toma O(1) tiempo por operación. Devolverá verdadero si mySet está vacío; de lo contrario, devolverá false si mySet no está vacío.
Sintaxis:
miConjunto.estáVacío
7. sorted(): esta función organiza temporalmente los elementos del conjunto en orden ordenado. Por temporalmente, queremos decir que después de esta operación, el conjunto se ordena de nuevo en orden aleatorio. Toma un tiempo O(n log n), donde n es la longitud del conjunto.
Sintaxis:
miConjunto.ordenado()
8. firstIndex(x): este método se utiliza para obtener el valor hash del índice del elemento x. Toma O (1) tiempo por operación.
Sintaxis:
primerÍndice(x)
9. randomElement()!: Devuelve un elemento aleatorio del conjunto. Toma el tiempo O(1) solo si la colección se ajusta a RandomAccessCollection; de lo contrario, toma el tiempo O(n), donde n es la longitud de la colección.
Sintaxis:
miConjunto.ElementoAleatorio()!