MUI o Material-UI es una biblioteca de interfaz de usuario que proporciona componentes robustos y personalizables predefinidos para React para facilitar el desarrollo web. El diseño de MUI se basa en la parte superior de Material Design de Google.
En este artículo, vamos a discutir la API React MUI TableFooter . El pie de tabla se utiliza para mostrar información diferente, como el número de columnas seleccionadas o los nombres de las columnas. Las tablas se utilizan para mostrar una colección de datos de forma organizada. La API proporciona muchas funcionalidades y aprenderemos a implementarlas.
Importar API de pie de tabla:
import TableFooter from '@mui/material/TableFooter'; // or import { TableFooter } from '@mui/material';
Lista de accesorios: aquí está la lista de diferentes accesorios utilizados con este componente. Podemos acceder a ellos y modificarlos según nuestras necesidades.
- children (Node): Es un componente similar a la fila de la tabla.
- clases (Objeto): reemplaza los estilos existentes o agrega nuevos estilos al componente.
- componente (elementType): Es el componente utilizado para el Node raíz. Puede ser una string HTML o un componente.
- sx (Array<func/objeto/bool>/func/objeto): La propiedad del sistema permite definir anulaciones del sistema, así como estilos CSS adicionales
Sintaxis: cree un componente TableFooter dentro de la tabla de la siguiente manera:
<Table sx={{ minWidth: 650 }}> <TableFooter> <TableCell>Total Number of Rows is 3</TableCell> </TableFooter> </Table>
Instalar y crear la aplicación React y agregar las dependencias de MUI.
Paso 1: Cree un proyecto de reacción usando el siguiente comando.
npx create-react-app gfg_tutorial
Paso 2: Entrar en el directorio del proyecto
cd gfg_tutorial
Paso 3: instale las dependencias de MUI de la siguiente manera:
npm install @mui/material @emotion/react @emotion/styled @mui/lab
Paso 4: Ejecute el proyecto de la siguiente manera:
npm start
Ejemplo 1: En el siguiente ejemplo, tenemos una tabla con un pie de página que muestra el número de columnas.
App.js
import "./App.css"; import * as React from "react"; import Table from "@mui/material/Table"; import TableBody from "@mui/material/TableBody"; import TableCell from "@mui/material/TableCell"; import TableContainer from "@mui/material/TableContainer"; import TableHead from "@mui/material/TableHead"; import TableRow from "@mui/material/TableRow"; import Paper from "@mui/material/Paper"; import { TableFooter } from "@mui/material"; function createData(index = 0, tutorial = "", link = "") { return { index, tutorial, link }; } const rows = [ createData( 1, "Data Structures", "https://www.geeksforgeeks.org/data-structures/?ref=shm" ), createData( 2, "Algorithms", "https://www.geeksforgeeks.org/fundamentals-of-algorithms/?ref=shm" ), createData( 3, "Competitive Programming", "https://www.geeksforgeeks.org/competitive-programming-a-complete-guide/?ref=shm" ), ]; function App() { return ( <div className="App"> <div className="head" style={{ width: "fit-content", margin: "auto", }} > <h1 style={{ color: "green", }} > GeeksforGeeks </h1> <strong>React MUI TableFooter API</strong> </div> <TableContainer component={Paper}> <Table sx={{ minWidth: 650 }}> <TableHead> <TableRow> <TableCell>Sl. No.</TableCell> <TableCell>Tutorial</TableCell> <TableCell>Link</TableCell> </TableRow> </TableHead> <TableBody> {rows.map((row) => ( <TableRow key={row.name}> <TableCell component="th" scope="row"> {row.index} </TableCell> <TableCell>{row.tutorial}</TableCell> <TableCell> <a href={row.link} target="_blank"> {row.link} </a> </TableCell> </TableRow> ))} </TableBody> <TableFooter> <TableCell>Total Number of Rows is 3</TableCell> </TableFooter> </Table> </TableContainer> </div> ); } export default App;
Paso para ejecutar la aplicación: Abra la terminal y escriba el siguiente comando.
npm start
Producción:
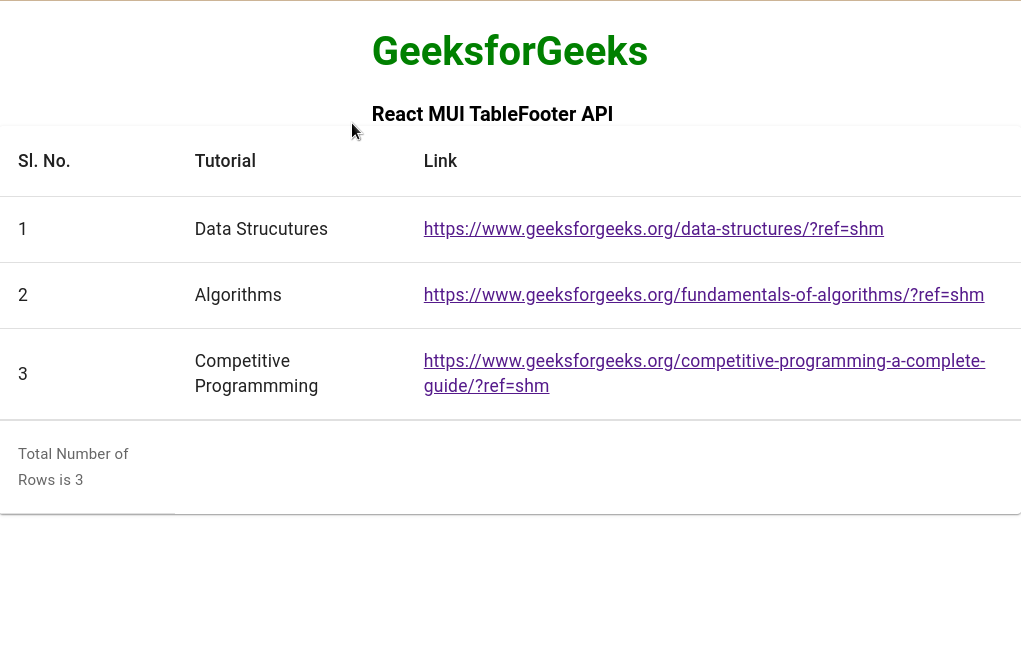
Ejemplo 2 : en este ejemplo, tenemos las filas seleccionables y el índice seleccionado se muestra en el pie de página.
App.js
import "./App.css"; import * as React from "react"; import Table from "@mui/material/Table"; import TableBody from "@mui/material/TableBody"; import TableCell from "@mui/material/TableCell"; import TableContainer from "@mui/material/TableContainer"; import TableHead from "@mui/material/TableHead"; import TableRow from "@mui/material/TableRow"; import Paper from "@mui/material/Paper"; import { TableFooter } from "@mui/material"; import { useState } from "react"; function createData(index = 0, tutorial = "", link = "") { return { index, tutorial, link }; } const rows = [ createData( 1, "Data Structures", "https://www.geeksforgeeks.org/data-structures/?ref=shm" ), createData( 2, "Algorithms", "https://www.geeksforgeeks.org/fundamentals-of-algorithms/?ref=shm" ), createData( 3, "Competitive Programming", "https://www.geeksforgeeks.org/competitive-programming-a-complete-guide/?ref=shm" ), ]; function App() { const [selectedRow, setSelectedRow] = useState(); return ( <div className="App"> <div className="head" style={{ width: "fit-content", margin: "auto", }} > <h1 style={{ color: "green", }} > GeeksforGeeks </h1> <strong>React MUI TableFooter API</strong> </div> <TableContainer component={Paper}> <Table sx={{ minWidth: 650 }}> <TableHead> <TableRow> <TableCell>Sl. No.</TableCell> <TableCell>Tutorial</TableCell> <TableCell>Link</TableCell> </TableRow> </TableHead> <TableBody> {rows.map((row, index) => ( <TableRow sx={ index == selectedRow ? { backgroundColor: "lightcoral" } : {} } onClick={() => { setSelectedRow(index); }} key={row.name} > <TableCell component="th" scope="row"> {row.index} </TableCell> <TableCell>{row.tutorial}</TableCell> <TableCell> <a href={row.link} target="_blank"> {row.link} </a> </TableCell> </TableRow> ))} </TableBody> <TableFooter> <TableCell>Total Number of Rows is 3</TableCell> <TableCell>Number of Selected Rows is {selectedRow}</TableCell> </TableFooter> </Table> </TableContainer> </div> ); } export default App;
Producción:
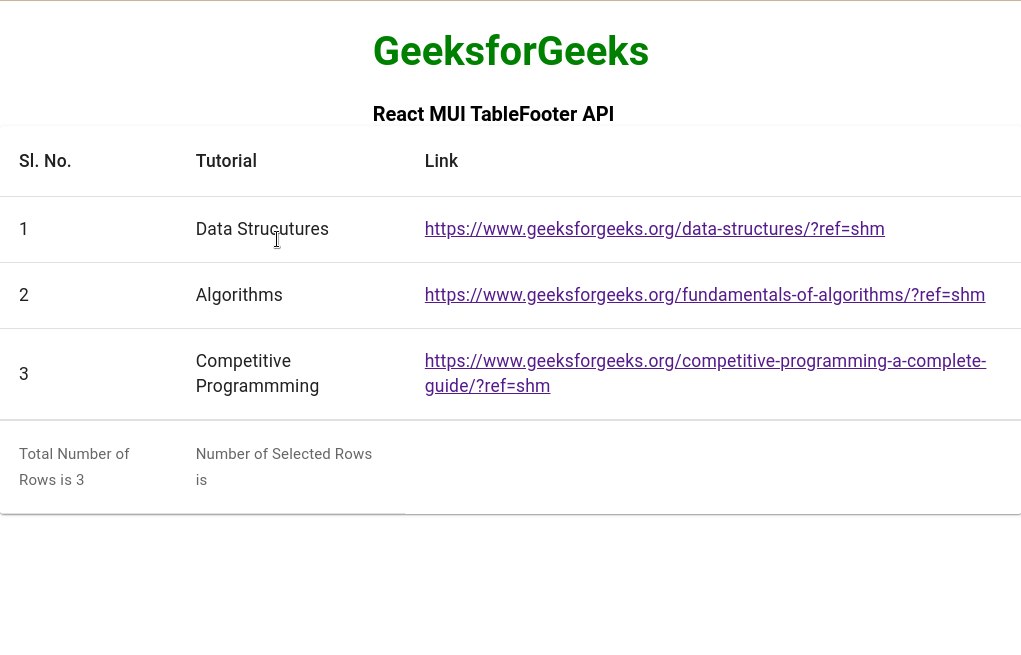
Referencia: https://mui.com/material-ui/api/table-footer/
Publicación traducida automáticamente
Artículo escrito por RajeevSarkar y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA