Material-UI es una biblioteca de interfaz de usuario que proporciona componentes robustos y personalizables predefinidos para React para facilitar el desarrollo web. El diseño de MUI se basa en la parte superior de Material Design de Google.
En este tutorial, vamos a discutir la API TableSortLabel de React MUI . La función de clasificación se puede implementar en las tablas mediante TableSortLabel. La API proporciona muchas funcionalidades y vamos a aprender a implementarlas.
Importar API TableSortLabel
import TableSortLabel from '@mui/material/TableSortLabel'; // or import { TableSortLabel } from '@mui/material';
Lista de accesorios : aquí está la lista de diferentes accesorios utilizados con este componente. Podemos acceder a ellos y modificarlos según nuestras necesidades.
- children : Es un componente similar a la fila de la tabla.
- clases : esto anula los estilos existentes o agrega nuevos estilos al componente.
- sx : El accesorio del sistema permite definir anulaciones del sistema, así como estilos CSS adicionales.
- active : si se establece en verdadero, la etiqueta tendrá un estilo activo.
- dirección (asc/desc) : toma la dirección de clasificación.
- hideSortIcon : si es verdadero, oculta el icono de clasificación.
- IconComponent : Es el icono de la TableSortLable.
Sintaxis : cree el componente TableSortLabel de la siguiente manera:
<TableSortLabel active={orderBy === headCell.id} direction={orderBy === headCell.id ? order : 'asc'} onClick={createSortHandler(headCell.id)} > {headCell.label} {orderBy === headCell.id ? ( <Box component="span" sx={visuallyHidden}> {order === 'desc' ? 'sorted descending' : 'sorted ascending'} </Box> ) : null} </TableSortLabel>
Instalar y crear la aplicación React y agregar las dependencias de MUI.
Paso 1: Cree un proyecto de reacción usando el siguiente comando.
npx create-react-app gfg_tutorial
Paso 2 : Entrar en el directorio del proyecto
cd gfg_tutorial
Paso 3 : instale las dependencias de MUI de la siguiente manera:
npm install @mui/material @emotion/react @emotion/styled @mui/lab
Paso 4 : Ejecute el proyecto de la siguiente manera:
npm start
Ejemplo 1 : en el siguiente ejemplo, tenemos una tabla con TableSortLabel.
App.js
import * as React from 'react' import PropTypes from 'prop-types' import { Box, Table, TableRow, TableCell, TableHead, TableContainer, TableBody, TableSortLabel, Paper, Toolbar, } from '@mui/material' import { visuallyHidden } from '@mui/utils' function App() { return ( <div className="App"> <div className="head" style={{ width: 'fit-content', margin: 'auto', }} > <h1 style={{ color: 'green', }} > GeeksforGeeks </h1> <strong>React MUI TableSortLabel API</strong> </div> <TableSorter /> </div> ) } function createData(name, likes) { return { name, likes, } } const rows = [ createData('C++', 305), createData('Java', 452), createData('Python', 262), createData('React', 159), createData('Rust', 356), ] function descendingComparator(a, b, orderBy) { if (b[orderBy] < a[orderBy]) { return -1 } if (b[orderBy] > a[orderBy]) { return 1 } return 0 } function getComparator(order, orderBy) { return order === 'desc' ? (a, b) => descendingComparator(a, b, orderBy) : (a, b) => -descendingComparator(a, b, orderBy) } // This method is created for cross-browser // compatibility, if you don't need to support IE11, // you can use Array.prototype.sort() directly function stableSort(array, comparator) { const stabilizedThis = array.map((el, index) => [el, index]) stabilizedThis.sort((a, b) => { const order = comparator(a[0], b[0]) if (order !== 0) { return order } return a[1] - b[1] }) return stabilizedThis.map((el) => el[0]) } const headCells = [ { id: 'name', numeric: false, disablePadding: true, label: 'Name', }, { id: 'likes', numeric: true, disablePadding: false, label: 'Likes', }, ] function EnhancedTableHead(props) { const { onSelectAllClick, order, orderBy, numSelected, rowCount, onRequestSort, } = props const createSortHandler = (property) => (event) => { onRequestSort(event, property) } return ( <TableHead> <TableRow> {headCells.map((headCell) => ( <TableCell key={headCell.id} align="center" padding={headCell.disablePadding ? 'none' : 'normal'} sortDirection={orderBy === headCell.id ? order : false} > <TableSortLabel direction={orderBy === headCell.id ? order : 'asc'} onClick={createSortHandler(headCell.id)} > {headCell.label} {orderBy === headCell.id ? ( <Box component="span" sx={visuallyHidden}> {order === 'desc' ? 'sorted descending' : 'sorted ascending'} </Box> ) : null} </TableSortLabel> </TableCell> ))} </TableRow> </TableHead> ) } EnhancedTableHead.propTypes = { numSelected: PropTypes.number.isRequired, onRequestSort: PropTypes.func.isRequired, onSelectAllClick: PropTypes.func.isRequired, order: PropTypes.oneOf(['asc', 'desc']).isRequired, orderBy: PropTypes.string.isRequired, rowCount: PropTypes.number.isRequired, } const EnhancedTableToolbar = (props) => { const { numSelected } = props return <Toolbar></Toolbar> } EnhancedTableToolbar.propTypes = { numSelected: PropTypes.number.isRequired, } function TableSorter() { const [order, setOrder] = React.useState('asc') const [orderBy, setOrderBy] = React.useState('calories') const [selected, setSelected] = React.useState([]) const [page, setPage] = React.useState(0) const [rowsPerPage, setRowsPerPage] = React.useState(5) const handleRequestSort = (event, property) => { const isAsc = orderBy === property && order === 'asc' setOrder(isAsc ? 'desc' : 'asc') setOrderBy(property) } const handleSelectAllClick = (event) => { if (event.target.checked) { const newSelecteds = rows.map((n) => n.name) setSelected(newSelecteds) return } setSelected([]) } const handleClick = (event, name) => { const selectedIndex = selected.indexOf(name) let newSelected = [] if (selectedIndex === -1) { newSelected = newSelected.concat(selected, name) } else if (selectedIndex === 0) { newSelected = newSelected.concat(selected.slice(1)) } else if (selectedIndex === selected.length - 1) { newSelected = newSelected.concat(selected.slice(0, -1)) } else if (selectedIndex > 0) { newSelected = newSelected.concat( selected.slice(0, selectedIndex), selected.slice(selectedIndex + 1), ) } setSelected(newSelected) } const isSelected = (name) => selected.indexOf(name) !== -1 return ( <Box sx={{ width: '100%' }}> <Paper sx={{ mb: 2, px: 2 }}> <EnhancedTableToolbar numSelected={selected.length} /> <TableContainer> <Table aria-labelledby="tableTitle"> <EnhancedTableHead numSelected={selected.length} order={order} orderBy={orderBy} onSelectAllClick={handleSelectAllClick} onRequestSort={handleRequestSort} rowCount={rows.length} /> <TableBody> {/* if you don't need to support IE11, you can replace the `stableSort` call with: rows.slice().sort(getComparator(order, orderBy)) */} {stableSort(rows, getComparator(order, orderBy)) .slice(page * rowsPerPage, page * rowsPerPage + rowsPerPage) .map((row, index) => { const isItemSelected = isSelected(row.name) const labelId = `enhanced-table-checkbox-${index}` return ( <TableRow hover onClick={(event) => handleClick(event, row.name)} role="checkbox" aria-checked={isItemSelected} tabIndex={-1} key={row.name} selected={isItemSelected} > <TableCell component="th" id={labelId} scope="row" padding="none" align="center" > {row.name} </TableCell> <TableCell align="center"> {row.likes}</TableCell> </TableRow> ) })} </TableBody> </Table> </TableContainer> </Paper> </Box> ) } export default App
Producción:
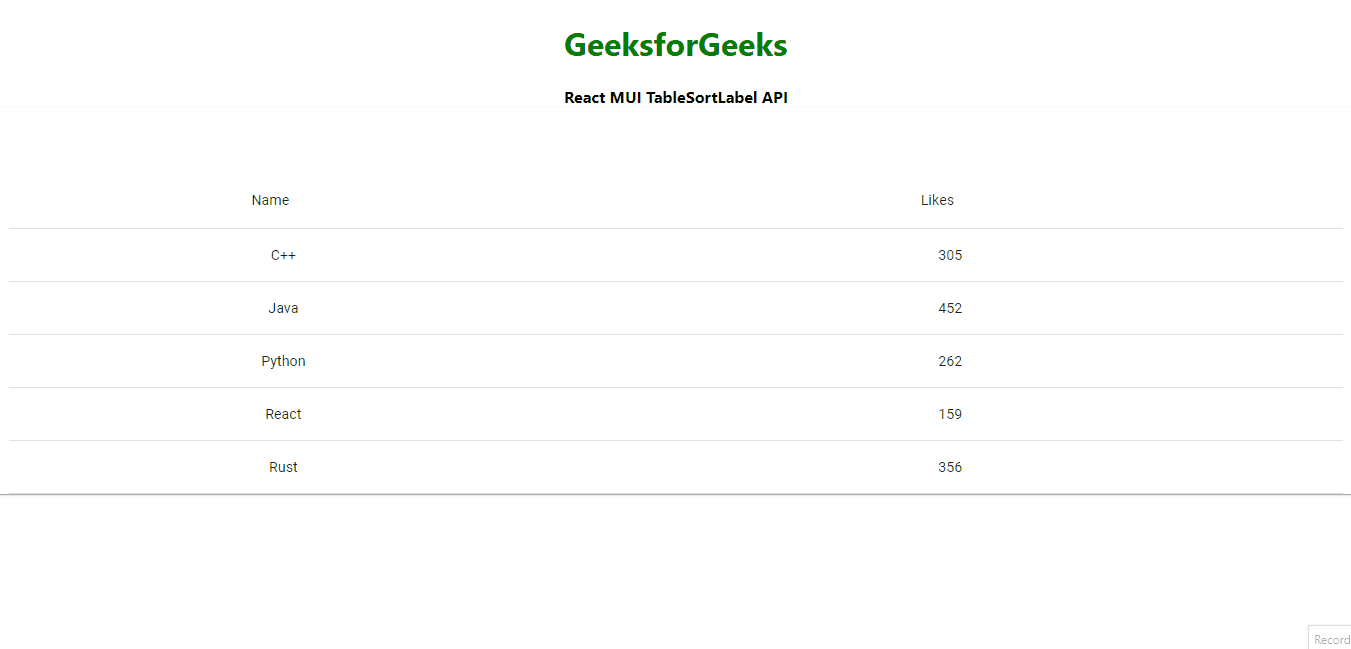
Ejemplo 2 : En el siguiente ejemplo, hemos configurado el activo que mostrará el icono al final.
App.js
import * as React from 'react' import PropTypes from 'prop-types' import { Box, Table, TableRow, TableCell, TableHead, TableContainer, TableBody, TableSortLabel, Paper, Toolbar, } from '@mui/material' import { visuallyHidden } from '@mui/utils' function App() { return ( <div className="App"> <div className="head" style={{ width: 'fit-content', margin: 'auto', }} > <h1 style={{ color: 'green', }} > GeeksforGeeks </h1> <strong> React MUI TableSortLabel API</strong> </div> <TableSorter /> </div> ) } function createData(name, likes) { return { name, likes, } } const rows = [ createData('C++', 305), createData('Java', 452), createData('Python', 262), createData('React', 159), createData('Rust', 356), ] function descendingComparator(a, b, orderBy) { if (b[orderBy] < a[orderBy]) { return -1 } if (b[orderBy] > a[orderBy]) { return 1 } return 0 } function getComparator(order, orderBy) { return order === 'desc' ? (a, b) => descendingComparator(a, b, orderBy) : (a, b) => -descendingComparator(a, b, orderBy) } // This method is created for cross-browser // compatibility, if you don't need to support IE11, // you can use Array.prototype.sort() directly function stableSort(array, comparator) { const stabilizedThis = array.map((el, index) => [el, index]) stabilizedThis.sort((a, b) => { const order = comparator(a[0], b[0]) if (order !== 0) { return order } return a[1] - b[1] }) return stabilizedThis.map((el) => el[0]) } const headCells = [ { id: 'name', numeric: false, disablePadding: true, label: 'Name', }, { id: 'likes', numeric: true, disablePadding: false, label: 'Likes', }, ] function EnhancedTableHead(props) { const { onSelectAllClick, order, orderBy, numSelected, rowCount, onRequestSort, } = props const createSortHandler = (property) => (event) => { onRequestSort(event, property) } return ( <TableHead> <TableRow> {headCells.map((headCell) => ( <TableCell key={headCell.id} align="center" padding={headCell.disablePadding ? 'none' : 'normal'} sortDirection={orderBy === headCell.id ? order : false} > <TableSortLabel active={orderBy === headCell.id} direction={orderBy === headCell.id ? order : 'asc'} onClick={createSortHandler(headCell.id)} > {headCell.label} {orderBy === headCell.id ? ( <Box component="span" sx={visuallyHidden}> {order === 'desc' ? 'sorted descending' : 'sorted ascending'} </Box> ) : null} </TableSortLabel> </TableCell> ))} </TableRow> </TableHead> ) } EnhancedTableHead.propTypes = { numSelected: PropTypes.number.isRequired, onRequestSort: PropTypes.func.isRequired, onSelectAllClick: PropTypes.func.isRequired, order: PropTypes.oneOf(['asc', 'desc']).isRequired, orderBy: PropTypes.string.isRequired, rowCount: PropTypes.number.isRequired, } const EnhancedTableToolbar = (props) => { const { numSelected } = props return <Toolbar></Toolbar> } EnhancedTableToolbar.propTypes = { numSelected: PropTypes.number.isRequired, } function TableSorter() { const [order, setOrder] = React.useState('asc') const [orderBy, setOrderBy] = React.useState('calories') const [selected, setSelected] = React.useState([]) const [page, setPage] = React.useState(0) const [rowsPerPage, setRowsPerPage] = React.useState(5) const handleRequestSort = (event, property) => { const isAsc = orderBy === property && order === 'asc' setOrder(isAsc ? 'desc' : 'asc') setOrderBy(property) } const handleSelectAllClick = (event) => { if (event.target.checked) { const newSelecteds = rows.map((n) => n.name) setSelected(newSelecteds) return } setSelected([]) } const handleClick = (event, name) => { const selectedIndex = selected.indexOf(name) let newSelected = [] if (selectedIndex === -1) { newSelected = newSelected.concat(selected, name) } else if (selectedIndex === 0) { newSelected = newSelected.concat(selected.slice(1)) } else if (selectedIndex === selected.length - 1) { newSelected = newSelected.concat(selected.slice(0, -1)) } else if (selectedIndex > 0) { newSelected = newSelected.concat( selected.slice(0, selectedIndex), selected.slice(selectedIndex + 1), ) } setSelected(newSelected) } const isSelected = (name) => selected.indexOf(name) !== -1 return ( <Box sx={{ width: '100%' }}> <Paper sx={{ mb: 2, px: 2 }}> <EnhancedTableToolbar numSelected={selected.length} /> <TableContainer> <Table aria-labelledby="tableTitle"> <EnhancedTableHead numSelected={selected.length} order={order} orderBy={orderBy} onSelectAllClick={handleSelectAllClick} onRequestSort={handleRequestSort} rowCount={rows.length} /> <TableBody> {/* if you don't need to support IE11, you can replace the `stableSort` call with: rows.slice().sort(getComparator(order, orderBy)) */} {stableSort(rows, getComparator(order, orderBy)) .slice(page * rowsPerPage, page * rowsPerPage + rowsPerPage) .map((row, index) => { const isItemSelected = isSelected(row.name) const labelId = `enhanced-table-checkbox-${index}` return ( <TableRow hover onClick={(event) => handleClick(event, row.name)} role="checkbox" aria-checked={isItemSelected} tabIndex={-1} key={row.name} selected={isItemSelected} > <TableCell component="th" id={labelId} scope="row" padding="none" align="center" > {row.name} </TableCell> <TableCell align="center"> {row.likes}</TableCell> </TableRow> ) })} </TableBody> </Table> </TableContainer> </Paper> </Box> ) } export default App
Producción:
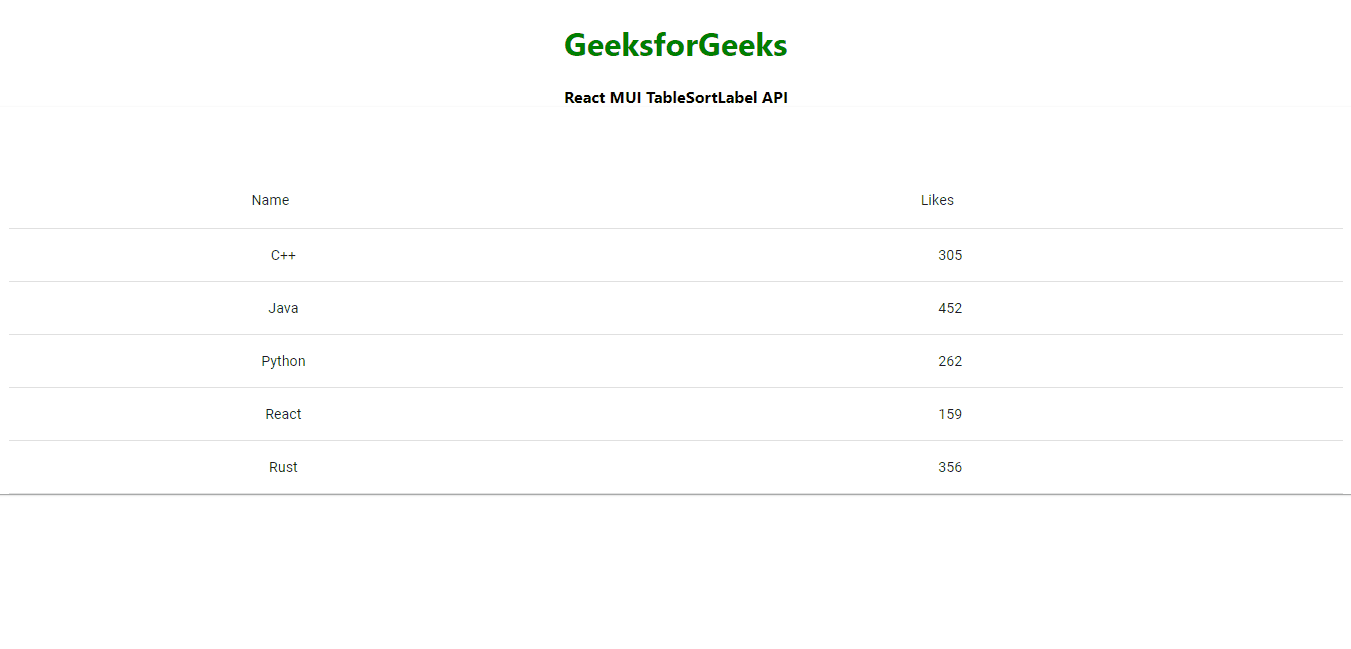
Referencia: https://mui.com/material-ui/api/table-sort-label/
Publicación traducida automáticamente
Artículo escrito por manavsarkar07 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA