React Suite es una biblioteca front-end popular con un conjunto de componentes React que están diseñados para la plataforma intermedia y los productos back-end. Apile los componentes de diseño rápidamente a través de Flexbox, admita el apilamiento vertical y horizontal y admita espaciado y ajuste personalizados.
Stack Interactive se utiliza para alinear elementos, justificar contenido y establecer la dirección de la pila.
Sintaxis:
<Stack direction={direction} alignItems={alignment} justifyContent={justifyContent}> <Button >....</Button> </Stack>
Creación de la aplicación React e instalación del módulo:
Paso 1: Cree una aplicación React usando el siguiente comando:
npx create-react-app foldername
Paso 2: después de crear la carpeta de su proyecto, es decir, el nombre de la carpeta, acceda a ella con el siguiente comando:
cd foldername
Paso 3: Después de crear la aplicación ReactJS, instale el módulo requerido usando el siguiente comando:
npm install rsuite
Estructura del proyecto: Tendrá el siguiente aspecto:
Ejemplo 1: ahora escriba el siguiente código en el archivo App.js. Aquí, la aplicación es nuestro componente predeterminado donde hemos escrito nuestro código. En este ejemplo, aprenderemos sobre la dirección y la justificación del contenido en Stack Interactive.
Javascript
import React,{useState} from 'react'; import 'rsuite/dist/rsuite.min.css'; import Stack from 'rsuite/Stack'; import Button from '@mui/material/Button'; import { Radio, RadioGroup } from 'rsuite'; export default function App(){ const [justifyContent, setJustifyContent] = React.useState('flex-start'); const [alignItems, setAlignItems] = React.useState('flex-start'); const [direction, setDirection] = React.useState('row'); return ( <div> <h1 style={{color:'green'}}>GeeksforGeeks</h1> <h3>React Suite Stack Interactive</h3> <Stack spacing={6} direction={direction} alignItems={alignItems} justifyContent={justifyContent} > <Button style={{color:'white',background:'green'}}>Item 1</Button> <Button style={{color:'white',background:'red'}}>Item 2</Button> <Button style={{color:'black',background:'yellow'}}>Item 3</Button> <Button style={{color:'white',background:'violet'}} >Item 4</Button> </Stack> <hr /> <Stack> <label>direction:</label> <RadioGroup inline value={direction} onChange={setDirection}> <Radio value="row">row</Radio> <Radio value="row-reverse">row-reverse</Radio> <Radio value="column">column</Radio> <Radio value="column-reverse">column-reverse</Radio> </RadioGroup> </Stack> <Stack> <label>justifyContent:</label> <RadioGroup inline value={justifyContent} onChange={setJustifyContent}> <Radio value="flex-start">flex-start</Radio> <Radio value="center">center</Radio> <Radio value="flex-end">flex-end</Radio> <Radio value="space-between">space-between</Radio> <Radio value="space-around">space-around</Radio> </RadioGroup> </Stack> </div> ) }
Paso para ejecutar la aplicación: ejecute la aplicación utilizando el siguiente comando desde el directorio raíz del proyecto:
npm start
Salida: Ahora abra su navegador y vaya a http://localhost:3000/, verá la siguiente salida:
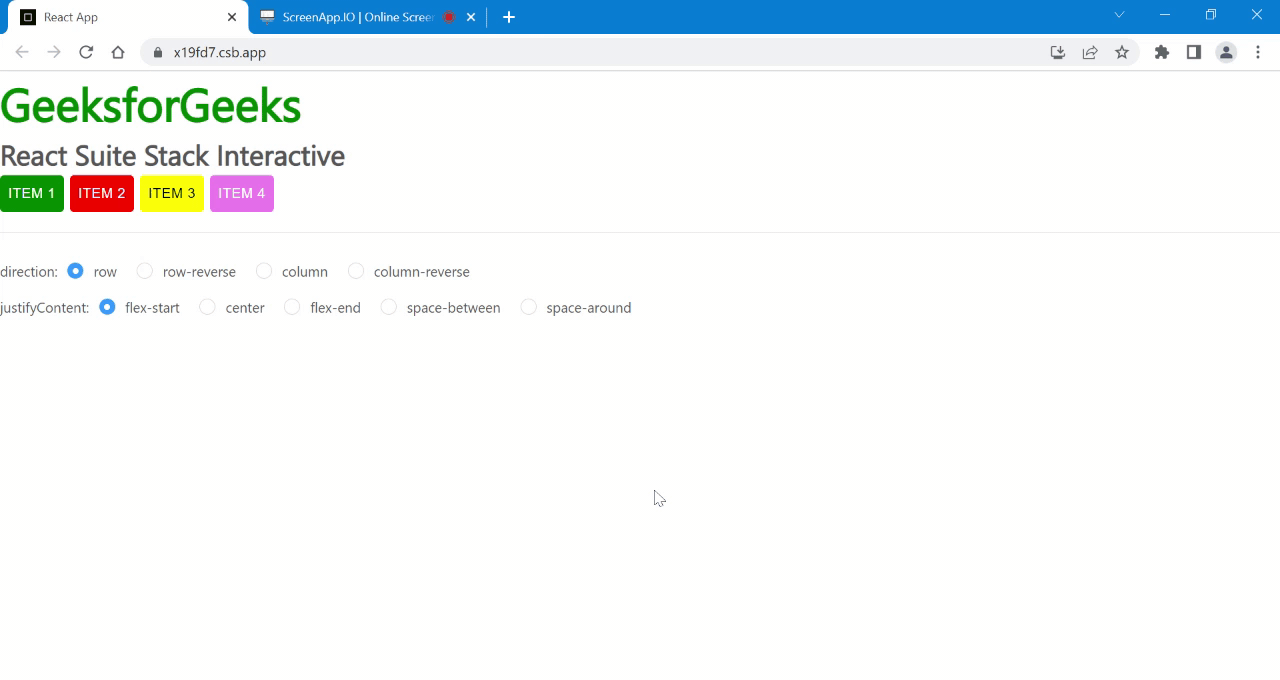
Ejemplo 2: en este ejemplo, aprenderemos sobre alignItems en Stack Interactive
Javascript
import React,{useState} from 'react'; import 'rsuite/dist/rsuite.min.css'; import Stack from 'rsuite/Stack'; import Button from '@mui/material/Button'; import { Radio, RadioGroup } from 'rsuite'; export default function App(){ const [justifyContent, setJustifyContent] = React.useState('flex-start'); const [alignItems, setAlignItems] = React.useState('flex-start'); const [direction, setDirection] = React.useState('column'); return ( <div> <h1 style={{color:'green'}}>GeeksforGeeks</h1> <h3>React Suite Stack Interactive</h3> <Stack spacing={6} direction={direction} alignItems={alignItems} justifyContent={justifyContent} > <Button style={{color:'white',background:'green'}}>Item 1</Button> <Button style={{color:'white',background:'red'}}>Item 2</Button> <Button style={{color:'black',background:'yellow'}}>Item 3</Button> <Button style={{color:'white',background:'violet'}} >Item 4</Button> </Stack> <hr /> <Stack> <label>alignItems:</label> <RadioGroup inline value={alignItems} onChange={setAlignItems}> <Radio value="flex-start">flex-start</Radio> <Radio value="center">center</Radio> <Radio value="flex-end">flex-end</Radio> <Radio value="stretch">stretch</Radio> <Radio value="baseline">baseline</Radio> </RadioGroup> </Stack> </div> ) }
Producción:
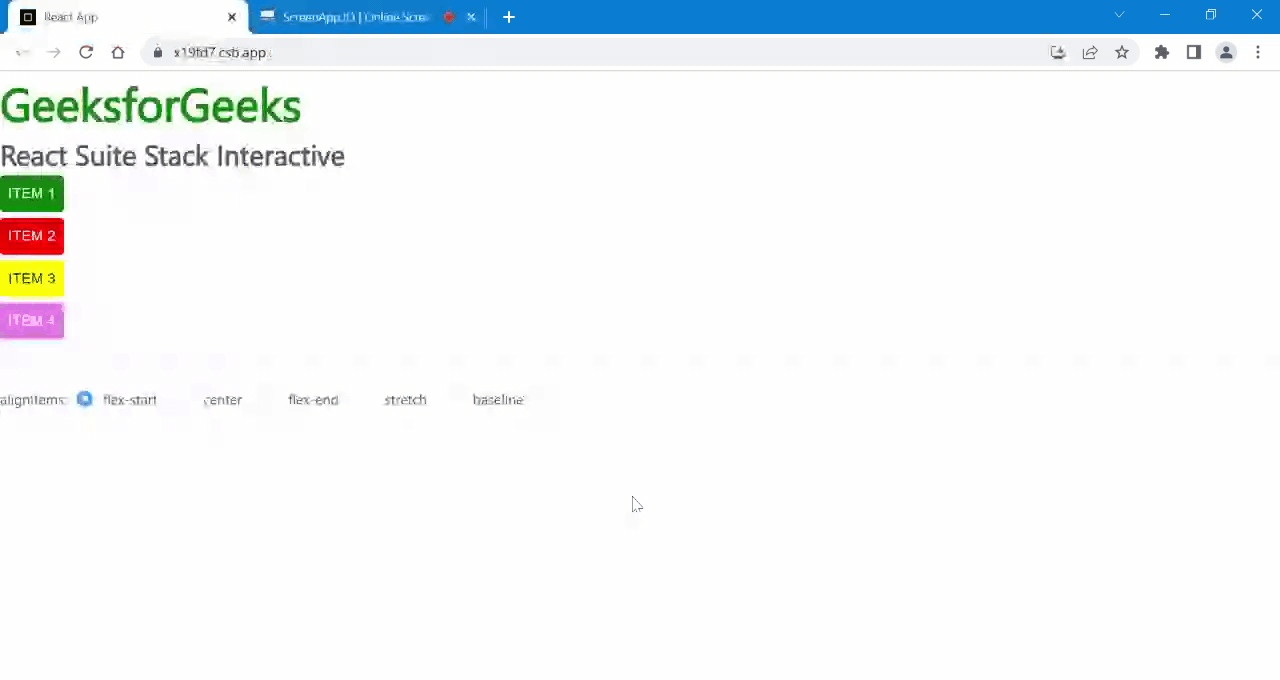
Referencia: https://rsuitejs.com/components/stack/#interactive
Publicación traducida automáticamente
Artículo escrito por akshitsaxenaa09 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA