Type Conversion o casting en Rust es una forma de convertir un tipo a otro. Como su nombre indica, la conversión de tipos es el proceso de convertir variables de un tipo de datos a otro. Entonces, el compilador trata la variable como un nuevo tipo de datos. Rust no nos permite convertir implícitamente el tipo de datos entre tipos primitivos.
Los tipos primitivos son i8, i16, i32, i64, u8, u16, u32, u64, f32, f64, char y bool. Aquí i8, i16, i32, i64 son tipos enteros con signo y u8, u16, u32, u64 son tipos enteros sin signo. f32 y f64 son tipos de punto flotante. char es un tipo de carácter único y bool es un tipo booleano.
El término implícitamente significa que el compilador no requiere que escribamos el código para convertir el tipo de datos. El compilador hace esto automáticamente. Pero rust no nos permite convertir implícitamente el tipo de datos entre tipos primitivos. Tenemos que convertir explícitamente el tipo de datos usando la palabra clave as .
Tipo de fundición:
- Conversión de tipos implícita
- Casting de tipo explícito
1. Casting de tipo implícito:
El encasillamiento implícito es el proceso de convertir un tipo de datos en otro. El compilador hace esto automáticamente.
Rust
// Implicit type casting results in an error fn main() { // main function // declaring the variable with float datatype let x = 1000.456456154651; // converting the float datatype to integer datatype // results in an error let y: i32 = x; println!("The value of y is: {}", y); }
Producción:
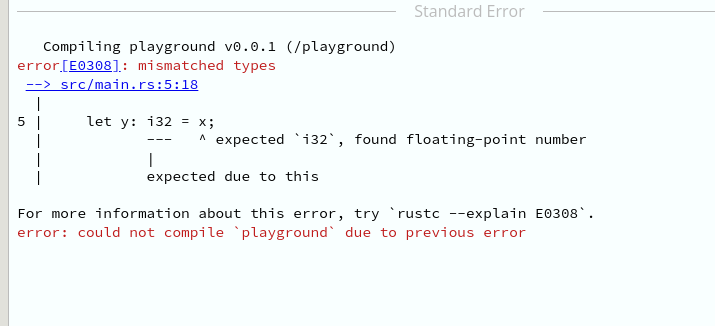
Rust no permite la conversión de tipos implícitos
El compilador de Rust no puede simplemente convertir el tipo de datos flotante al tipo de datos entero, tenemos que convertir explícitamente el tipo de datos. Por lo tanto, si intentamos ejecutar el código anterior, obtendremos un error.
2. Casting de tipo explícito:
El encasillamiento explícito se puede hacer usando la palabra clave as . La palabra clave as se utiliza para convertir de forma segura el tipo de datos. El compilador comprueba si el tipo de datos es correcto o no. Si el tipo de datos es correcto, el compilador convierte el tipo de datos. Si el tipo de datos es incorrecto, el compilador da un error. Para realizar una conversión segura, el encasillamiento debe realizarse desde un tipo de datos bajo a un tipo de datos alto.
Ejemplo 1:
Rust
// Rust program to convert the datatype from i32 to u32 // function to print the type of a variable fn print_type_of<T>(_: &T) { println!("{}", std::any::type_name::<T>()) } fn main() { // main function // declare x as i32 let x: i32 = 100000; // explicitly convert the datatype from i32 to u32 let y: u32 = x as u32; // print the type of x println!("Before Type Conversion of x "); print_type_of(&x); // print the type of y println!("After Type Conversion of x "); print_type_of(&y); }
Producción:
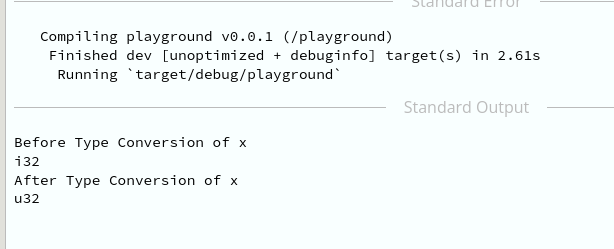
conversión de tipo de i32 a u32
Ejemplo 2:
Rust
// Rust program to convert the datatype from i32 to u32 // function to print the type of a variable fn print_type_of<T>(_: &T) { println!("{}", std::any::type_name::<T>()) } // type casting fn main() { // declare x as i32 let decimal_value = 65.4321_f32; // converting to integer and truncating let integer_value = decimal_value as u8; // converting integer to character using ascii table let character_value = integer_value as char; // printing the values and their datatype println!("The value of decimal_value is: {} and its datatype is ", decimal_value); print_type_of(&decimal_value); println!("The value of integer_value is: {} and its datatype is ", integer_value); print_type_of(&integer_value); println!("The value of character_value is: {} and its datatype is", character_value); print_type_of(&character_value); // printing the type of the variables println!("Casting from {} -> {} -> {}", decimal_value, integer_value, character_value); }
Producción:
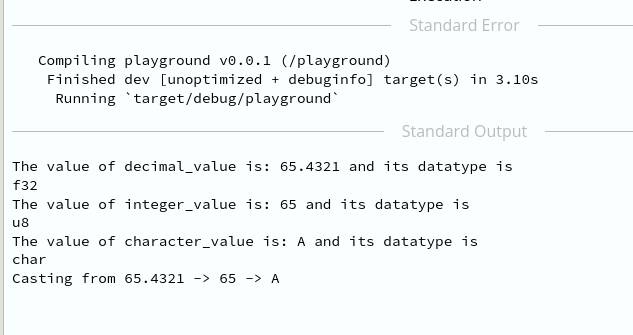
Tipo de conversión
Publicación traducida automáticamente
Artículo escrito por amnindersingh1414 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA