En este artículo, vamos a discutir cómo crear un script de python para monitorear los cambios en el sitio web. Puede codificar un programa para monitorear un sitio web y le notificará si hay algún cambio. Este programa tiene muchos escenarios útiles, por ejemplo, si el sitio web de su escuela ha actualizado algo que llegará a saber al respecto.
Acercarse:
Seguiremos los siguientes pasos para escribir este programa:
- Lea la URL que desea monitorear.
- Hash todo el sitio web.
- Espere una cantidad específica de segundos.
- Si hay algún cambio en comparación con el hash anterior, avíseme; de lo contrario, espere y vuelva a tomar el hash.
Bibliotecas requeridas:
Las bibliotecas que usaremos son:
- tiempo: Para esperar una cantidad de tiempo específica.
- hashlib: para codificar el contenido de todo el sitio web.
- urllib: Para realizar la solicitud de obtención y cargar el contenido del sitio web.
Implementación:
Python3
# Importing libraries import time import hashlib from urllib.request import urlopen, Request # setting the URL you want to monitor url = Request('https://leetcode.com/', headers={'User-Agent': 'Mozilla/5.0'}) # to perform a GET request and load the # content of the website and store it in a var response = urlopen(url).read() # to create the initial hash currentHash = hashlib.sha224(response).hexdigest() print("running") time.sleep(10) while True: try: # perform the get request and store it in a var response = urlopen(url).read() # create a hash currentHash = hashlib.sha224(response).hexdigest() # wait for 30 seconds time.sleep(30) # perform the get request response = urlopen(url).read() # create a new hash newHash = hashlib.sha224(response).hexdigest() # check if new hash is same as the previous hash if newHash == currentHash: continue # if something changed in the hashes else: # notify print("something changed") # again read the website response = urlopen(url).read() # create a hash currentHash = hashlib.sha224(response).hexdigest() # wait for 30 seconds time.sleep(30) continue # To handle exceptions except Exception as e: print("error")
Producción:
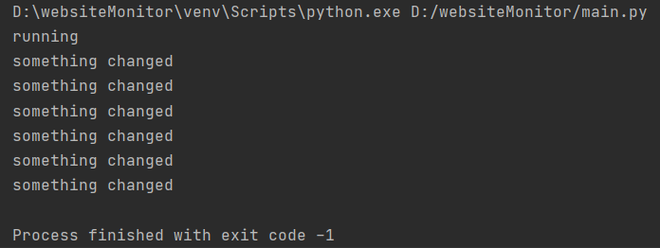
producción
Nota: time.sleep() toma segundos como parámetro. Puede realizar cambios para la notificación en lugar de imprimir el estado en el terminal, puede escribir un programa para recibir un correo electrónico.