De forma predeterminada, las páginas web están disponibles en inglés. Se puede proporcionar en los idiomas que elija el usuario mediante el concepto de «Internacionalización» en tecnología Java. La internacionalización (i18n) ayuda a los usuarios a ver las páginas según su nacionalidad o ubicación visitada. Un servlet puede recuperar la configuración regional del solicitante según su nacionalidad y devolverá el objeto Locale.
java.util.Locale request.getLocale()
Hay diferentes métodos disponibles para detectar la configuración regional.
número de serie |
Método |
Descripción |
---|---|---|
1 | obtenerPaís() | Ayuda a devolver el código de «país/región» en MAYÚSCULAS para la configuración regional en formato de 2 letras ISO 3166. |
2 | getDisplayCountry() | Ayuda a devolver un «NOMBRE» para el país de la configuración regional y es apropiado para mostrar al usuario. |
3 | obtenerIdioma() | Ayuda a devolver el «CÓDIGO DE IDIOMA» en minúsculas para la configuración regional en formato ISO 639. |
4 | getDisplayLanguage() | Ayuda a devolver un «NOMBRE PARA EL IDIOMA DE LA LOCALIDAD» y es apropiado para mostrarlo al usuario. |
5. | obtenerISO3País() | Es útil devolver una abreviatura de tres letras para el país de la localidad. |
6 | obtenerISO3Idioma() | Es útil devolver una abreviatura de tres letras para el idioma de la configuración regional. |
Ejemplos
Veamos diferentes ejemplos para mostrar un idioma y país asociado para una solicitud
Ejemplo 1:
Java
import java.io.IOException; import java.io.PrintWriter; import java.util.Locale; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; // Servlet implementation class SearchServlet @WebServlet("/getCurrentLocationInformation") public class GetLocationServlet extends HttpServlet { private static final long serialVersionUID = 1L; public GetLocationServlet() { super(); } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Get the Locale information // by using java.util.Locale Locale locale = request.getLocale(); // language String localeLanguage = locale.getLanguage(); // country String localeCountry = locale.getCountry(); // Print the response content type // below by using PrintWriter object response.setContentType("text/html"); PrintWriter printWriter = response.getWriter(); String title = "Locale detection"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; printWriter.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#145A32\">\n" + "<h1 align = \"Left\"> " + "<font color=\"white\">" + "Language : " + localeLanguage + "</font>" + "</h1>\n" + "<h2 align = \"Left\">" + "<font color=\"white\">" + "Country : " + localeCountry + "</font>" + "</h2>\n" + "</body>" + "</html>" ); } }
Al ejecutar el código anterior, podemos obtener el resultado de la siguiente manera
Producción:
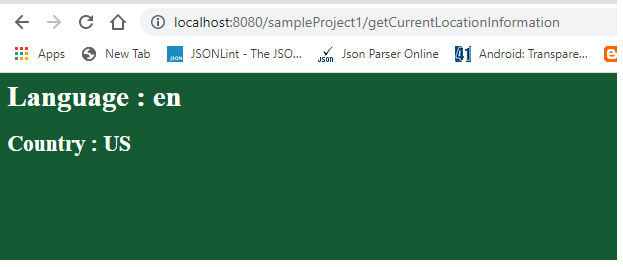
Configuración de idioma predeterminada
Si queremos configurar el idioma como el diferente, a través de la configuración de idioma se puede hacer.
// In order to set spanish language code. response.setHeader("Content-Language", "es"); // Similarly we can set different language codes in this way // http://www.mathguide.de/info/tools/languagecode.html provides various language code
Ejemplo 2:
Java
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Print the response content type below // by using PrintWriter object response.setContentType("text/html"); PrintWriter printWriter = response.getWriter(); // Set spanish language code. In this place, we need // to provide the required language setting response.setHeader("Content-Language", "es"); String title = "En Español"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; // As it is spanish, the messages // also given in spanish printWriter.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#145A32\">\n" + "<h1>" + "<font color=\"white\">" + "En Español:" + "</font>" + "</h1>\n" + "<h1>" + "<font color=\"white\">" + "Bienvenido a GeeksForGeeks!" + "</font>" + "</h1>\n" + "</body>"+ "</html>"); }
Producción:
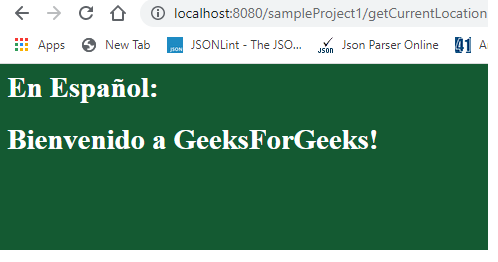
ajuste al idioma español
Ejemplo 3:
Específico para la configuración regional , mediante el uso de la clase java.text.DateFormat y el método getDateTimeInstance(), podemos formatear la fecha y la hora
Java
// import java.text.DateFormat as it is getting used protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter printWriter = response.getWriter(); // Get the client's Locale by using request.getLocale() Locale locale = request.getLocale( ); String date = DateFormat.getDateTimeInstance(DateFormat.FULL, DateFormat.SHORT, locale).format(new Date( )); String title = "Displaying Locale Specific Dates"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; printWriter.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#145A32\">\n" + "<h1 align = \"Left\">" + "<font color=\"white\">" + "Locale specific date : " + date + "</font>" + "</h1>\n" + "</body>" + "</html>" ); }
Producción:
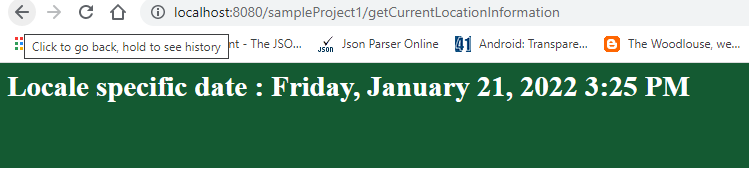
Visualización de la fecha local
Ejemplo 4:
Específico para la configuración regional , mediante el uso de la clase java.txt.NumberFormat y el método getCurrencyInstance(), podemos formatear la moneda
Java
// import java.text.NumberFormat; has to be imported protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter printWriter = response.getWriter(); // Get the current Locale information Locale currentLocale = request.getLocale( ); NumberFormat numberFormat = NumberFormat.getCurrencyInstance(currentLocale); String formattedCurrency = numberFormat.format(100000); String title = "Displaying Locale Specific Currency"; String docType = "<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n"; printWriter.println(docType + "<html>\n" + "<head><title>" + title + "</title></head>\n" + "<body bgcolor = \"#145A32\">\n" + "<h1 align = \"Left\">" + "<font color=\"white\">" + "Currency : " +formattedCurrency + "</font>" +"</h1>\n" + "</body>"+ "</html>" ); }
Producción:
Ventajas de la Internacionalización
- La captura de mercados extranjeros es más fácil.
- Representación de un resultado neutral para la configuración regional
- Al principio, si se implementa, la expansión del software en los mercados globales es más fácil.
Conclusión
Cualquier software que pretenda ser desarrollado en el mercado global, tiene que ser preparado de forma internacional solamente. En java, podemos utilizar las metodologías mencionadas anteriormente para lograr la internacionalización.
Publicación traducida automáticamente
Artículo escrito por priyarajtt y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA