El juego Snake es uno de los juegos de arcade más populares de todos los tiempos. En este juego, el objetivo principal del jugador es atrapar la mayor cantidad de frutas sin chocar contra la pared ni contra sí mismo. Crear un juego de serpientes puede ser un desafío mientras se aprende Python o Pygame. Es uno de los mejores proyectos para principiantes que todo programador novato debería tomar como un desafío. Aprender a construir un videojuego es un aprendizaje interesante y divertido.
Usaremos Pygame para crear este juego de serpientes. Pygame es una biblioteca de código abierto diseñada para crear videojuegos. Tiene bibliotecas de gráficos y sonidos incorporadas. También es apto para principiantes y multiplataforma.
Instalación
Para instalar Pygame, debe abrir su terminal o símbolo del sistema y escribir el siguiente comando:
pip install pygame
Después de instalar Pygame, estamos listos para crear nuestro genial juego de serpientes.
Un enfoque paso a paso para crear un juego de serpientes usando Pygame:
Paso 1: Primero estamos importando las bibliotecas necesarias.
- Después de eso, estamos definiendo el ancho y el alto de la ventana en la que se jugará el juego.
- Y definir el color en formato RGB que vamos a utilizar en nuestro juego para mostrar texto.
Python3
# importing libraries import pygame import time import random snake_speed = 15 # Window size window_x = 720 window_y = 480 # defining colors black = pygame.Color(0, 0, 0) white = pygame.Color(255, 255, 255) red = pygame.Color(255, 0, 0) green = pygame.Color(0, 255, 0) blue = pygame.Color(0, 0, 255)
Paso 2: después de importar las bibliotecas, necesitamos inicializar Pygame usando el método pygame.init() .
- Cree una ventana de juego utilizando el ancho y el alto definidos en el paso anterior.
- Aquí pygame.time.Clock() se usará más en la lógica principal del juego para cambiar la velocidad de la serpiente.
Python3
# Initialising pygame pygame.init() # Initialise game window pygame.display.set_caption('GeeksforGeeks Snakes') game_window = pygame.display.set_mode((window_x, window_y)) # FPS (frames per second) controller fps = pygame.time.Clock()
Paso 3: Inicialice la posición de la serpiente y su tamaño.
- Después de inicializar la posición de la serpiente, inicialice la posición de la fruta al azar en cualquier lugar de la altura y el ancho definidos.
- Al configurar la dirección a la DERECHA, nos aseguramos de que, cada vez que un usuario ejecute el programa/juego, la serpiente debe moverse directamente a la pantalla.
Python3
# defining snake default position snake_position = [100, 50] # defining first 4 blocks of snake # body snake_body = [ [100, 50], [90, 50], [80, 50], [70, 50] ] # fruit position fruit_position = [random.randrange(1, (window_x//10)) * 10, random.randrange(1, (window_y//10)) * 10] fruit_spawn = True # setting default snake direction # towards right direction = 'RIGHT' change_to = direction
Paso 4: crea una función para mostrar la puntuación del jugador.
- En esta función, primero estamos creando un objeto de fuente, es decir, el color de la fuente irá aquí.
- Luego estamos usando render para crear una superficie de fondo que vamos a cambiar cada vez que se actualice nuestra partitura.
- Cree un objeto rectangular para el objeto de superficie de texto (donde se actualizará el texto)
- Luego, mostramos nuestra puntuación usando blit. blit toma dos argumentos screen.blit(background,(x,y))
Python3
# initial score score = 0 # displaying Score function def show_score(choice, color, font, size): # creating font object score_font score_font = pygame.font.SysFont(font, size) # create the display surface object # score_surface score_surface = score_font.render('Score : ' + str(score), True, color) # create a rectangular object for the # text surface object score_rect = score_surface.get_rect() # displaying text game_window.blit(score_surface, score_rect)
Paso 5 : Ahora cree una función de finalización del juego que representará el puntaje después de que la serpiente sea golpeada por una pared o por sí misma.
- En la primera línea, estamos creando un objeto de fuente para mostrar las puntuaciones.
- Luego estamos creando superficies de texto para representar partituras.
- Después de eso, estamos configurando la posición del texto en el medio del área jugable.
- Muestre las puntuaciones usando blit y actualice la puntuación actualizando la superficie usando flip().
- Estamos usando sleep(2) para esperar 2 segundos antes de cerrar la ventana usando quit().
Python3
# game over function def game_over(): # creating font object my_font my_font = pygame.font.SysFont('times new roman', 50) # creating a text surface on which text # will be drawn game_over_surface = my_font.render('Your Score is : ' + str(score), True, red) # create a rectangular object for the text # surface object game_over_rect = game_over_surface.get_rect() # setting position of the text game_over_rect.midtop = (window_x/2, window_y/4) # blit will draw the text on screen game_window.blit(game_over_surface, game_over_rect) pygame.display.flip() # after 2 seconds we will quit the # program time.sleep(2) # deactivating pygame library pygame.quit() # quit the program quit()
Paso 6: Ahora crearemos nuestra función principal que hará lo siguiente:
- Validaremos las claves que serán responsables del movimiento de la serpiente, luego crearemos una condición especial para que la serpiente no pueda moverse en la dirección opuesta instantáneamente.
- Después de eso, si la serpiente y la fruta chocan, incrementaremos la puntuación en 10 y se generará nueva fruta.
- Después de eso, estamos comprobando si la serpiente es golpeada con una pared o no. Si una serpiente golpea una pared, llamaremos a la función game over.
- Si la serpiente se golpea a sí misma, se activará la función Game Over.
- Y al final, mostraremos los puntajes usando la función show_score creada anteriormente.
Python3
# Main Function while True: # handling key events for event in pygame.event.get(): if event.type == pygame.KEYDOWN: if event.key == pygame.K_UP: change_to = 'UP' if event.key == pygame.K_DOWN: change_to = 'DOWN' if event.key == pygame.K_LEFT: change_to = 'LEFT' if event.key == pygame.K_RIGHT: change_to = 'RIGHT' # If two keys pressed simultaneously # we don't want snake to move into two directions # simultaneously if change_to == 'UP' and direction != 'DOWN': direction = 'UP' if change_to == 'DOWN' and direction != 'UP': direction = 'DOWN' if change_to == 'LEFT' and direction != 'RIGHT': direction = 'LEFT' if change_to == 'RIGHT' and direction != 'LEFT': direction = 'RIGHT' # Moving the snake if direction == 'UP': snake_position[1] -= 10 if direction == 'DOWN': snake_position[1] += 10 if direction == 'LEFT': snake_position[0] -= 10 if direction == 'RIGHT': snake_position[0] += 10 # Snake body growing mechanism # if fruits and snakes collide then scores will be # incremented by 10 snake_body.insert(0, list(snake_position)) if snake_position[0] == fruit_position[0] and snake_position[1] == fruit_position[1]: score += 10 fruit_spawn = False else: snake_body.pop() if not fruit_spawn: fruit_position = [random.randrange(1, (window_x//10)) * 10, random.randrange(1, (window_y//10)) * 10] fruit_spawn = True game_window.fill(black) for pos in snake_body: pygame.draw.rect(game_window, green, pygame.Rect( pos[0], pos[1], 10, 10)) pygame.draw.rect(game_window, white, pygame.Rect( fruit_position[0], fruit_position[1], 10, 10)) # Game Over conditions if snake_position[0] < 0 or snake_position[0] > window_x-10: game_over() if snake_position[1] < 0 or snake_position[1] > window_y-10: game_over() # Touching the snake body for block in snake_body[1:]: if snake_position[0] == block[0] and snake_position[1] == block[1]: game_over() # displaying score countinuously show_score(1, white, 'times new roman', 20) # Refresh game screen pygame.display.update() # Frame Per Second /Refresh Rate fps.tick(snake_speed)
A continuación se muestra la implementación
Python3
# importing libraries import pygame import time import random snake_speed = 15 # Window size window_x = 720 window_y = 480 # defining colors black = pygame.Color(0, 0, 0) white = pygame.Color(255, 255, 255) red = pygame.Color(255, 0, 0) green = pygame.Color(0, 255, 0) blue = pygame.Color(0, 0, 255) # Initialising pygame pygame.init() # Initialise game window pygame.display.set_caption('GeeksforGeeks Snakes') game_window = pygame.display.set_mode((window_x, window_y)) # FPS (frames per second) controller fps = pygame.time.Clock() # defining snake default position snake_position = [100, 50] # defining first 4 blocks of snake body snake_body = [[100, 50], [90, 50], [80, 50], [70, 50] ] # fruit position fruit_position = [random.randrange(1, (window_x//10)) * 10, random.randrange(1, (window_y//10)) * 10] fruit_spawn = True # setting default snake direction towards # right direction = 'RIGHT' change_to = direction # initial score score = 0 # displaying Score function def show_score(choice, color, font, size): # creating font object score_font score_font = pygame.font.SysFont(font, size) # create the display surface object # score_surface score_surface = score_font.render('Score : ' + str(score), True, color) # create a rectangular object for the text # surface object score_rect = score_surface.get_rect() # displaying text game_window.blit(score_surface, score_rect) # game over function def game_over(): # creating font object my_font my_font = pygame.font.SysFont('times new roman', 50) # creating a text surface on which text # will be drawn game_over_surface = my_font.render( 'Your Score is : ' + str(score), True, red) # create a rectangular object for the text # surface object game_over_rect = game_over_surface.get_rect() # setting position of the text game_over_rect.midtop = (window_x/2, window_y/4) # blit will draw the text on screen game_window.blit(game_over_surface, game_over_rect) pygame.display.flip() # after 2 seconds we will quit the program time.sleep(2) # deactivating pygame library pygame.quit() # quit the program quit() # Main Function while True: # handling key events for event in pygame.event.get(): if event.type == pygame.KEYDOWN: if event.key == pygame.K_UP: change_to = 'UP' if event.key == pygame.K_DOWN: change_to = 'DOWN' if event.key == pygame.K_LEFT: change_to = 'LEFT' if event.key == pygame.K_RIGHT: change_to = 'RIGHT' # If two keys pressed simultaneously # we don't want snake to move into two # directions simultaneously if change_to == 'UP' and direction != 'DOWN': direction = 'UP' if change_to == 'DOWN' and direction != 'UP': direction = 'DOWN' if change_to == 'LEFT' and direction != 'RIGHT': direction = 'LEFT' if change_to == 'RIGHT' and direction != 'LEFT': direction = 'RIGHT' # Moving the snake if direction == 'UP': snake_position[1] -= 10 if direction == 'DOWN': snake_position[1] += 10 if direction == 'LEFT': snake_position[0] -= 10 if direction == 'RIGHT': snake_position[0] += 10 # Snake body growing mechanism # if fruits and snakes collide then scores # will be incremented by 10 snake_body.insert(0, list(snake_position)) if snake_position[0] == fruit_position[0] and snake_position[1] == fruit_position[1]: score += 10 fruit_spawn = False else: snake_body.pop() if not fruit_spawn: fruit_position = [random.randrange(1, (window_x//10)) * 10, random.randrange(1, (window_y//10)) * 10] fruit_spawn = True game_window.fill(black) for pos in snake_body: pygame.draw.rect(game_window, green, pygame.Rect(pos[0], pos[1], 10, 10)) pygame.draw.rect(game_window, white, pygame.Rect( fruit_position[0], fruit_position[1], 10, 10)) # Game Over conditions if snake_position[0] < 0 or snake_position[0] > window_x-10: game_over() if snake_position[1] < 0 or snake_position[1] > window_y-10: game_over() # Touching the snake body for block in snake_body[1:]: if snake_position[0] == block[0] and snake_position[1] == block[1]: game_over() # displaying score countinuously show_score(1, white, 'times new roman', 20) # Refresh game screen pygame.display.update() # Frame Per Second /Refresh Rate fps.tick(snake_speed)
Producción:
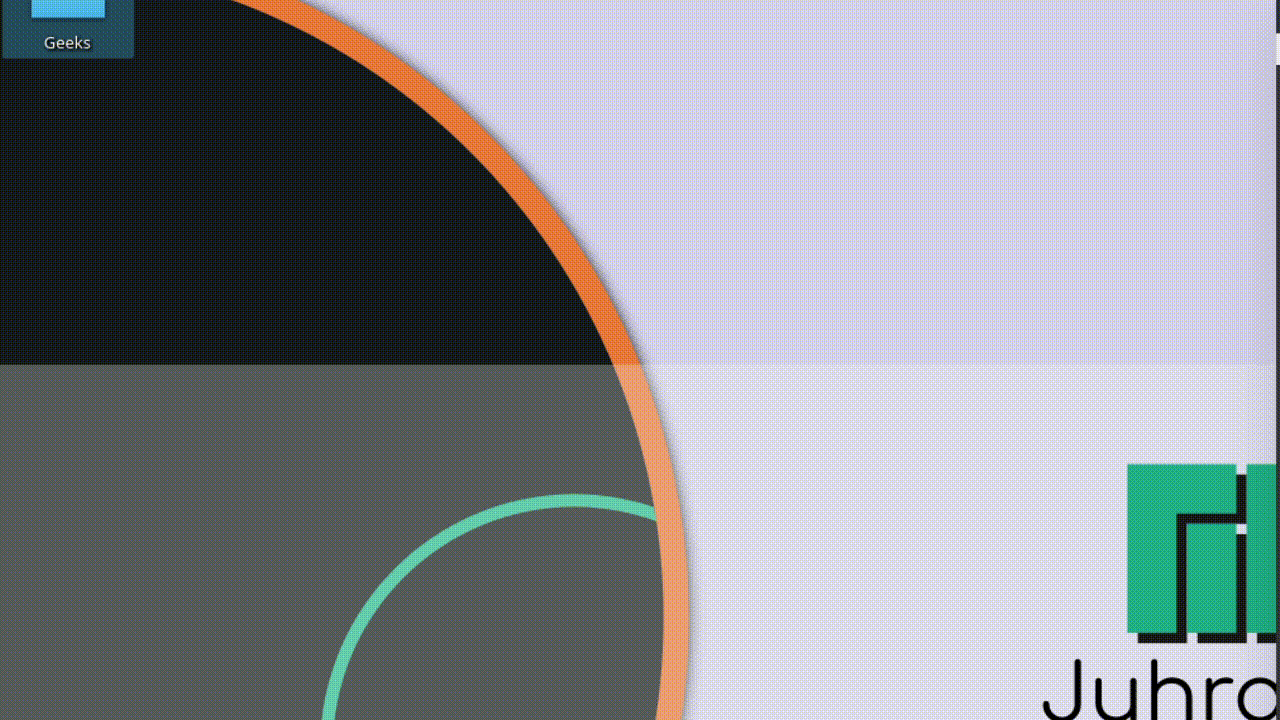
Serpiente usando Pygame
Publicación traducida automáticamente
Artículo escrito por amnindersingh1414 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA