Cada proyecto de Spring Boot tiene varios módulos y cada módulo coincide con alguna capa de aplicación (capa de servicio, capa de repositorio, capa web, modelo, etc.). En este artículo, veamos un proyecto impulsado por maven como ejemplo para mostrar módulos de proyecto.
Ejemplo
pom.xml (nivel general del proyecto)
XML
<?xml version="1.0" encoding="UTF-8" standalone="no"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <!-- Spring IO Platform is the parent of the generated application to be able to use Spring Boot and all its default configuration --> <parent> <groupId>io.spring.platform</groupId> <artifactId>platform-bom</artifactId> <version>2.0.1.RELEASE</version> </parent> <groupId>gfg.multimodule</groupId> <artifactId>gfg.multimodule</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>pom</packaging> <name>Parent - Pom Aggregator</name> <description>This pom is a maven aggregator that contains all application modules. Also, include all common dependencies needed by more than one module. Dependencies are defined without version because this project has defined Spring IO Platform as parent.</description> <properties> <java.version>1.6</java.version> </properties> <modules> <module>model</module> <module>repository</module> <module>service-api</module> <module>service-impl</module> <module>application</module> </modules> <dependencies> <!-- Spring Boot dependencies --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> </project>
Este proyecto de muestra contiene los siguientes módulos
aplicación (Aquí se mantiene el punto de partida de la aplicación Spring Boot y también contendrá páginas de representación)
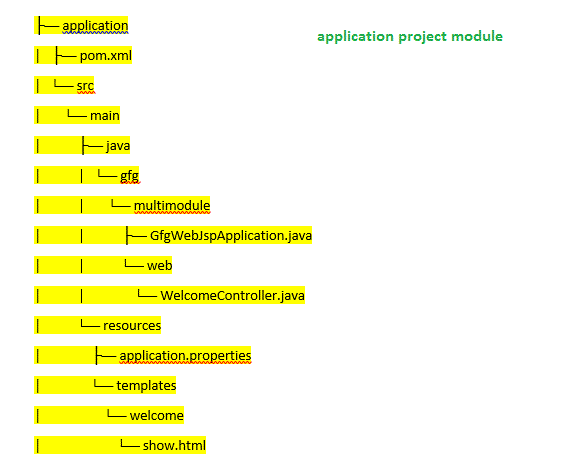
Veamos los archivos importantes clave
GfgWebJspApplication.java
Java
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class GfgWebJspApplication { public static void main(String[] args) throws Exception { SpringApplication.run(GfgWebJspApplication.class, args); } }
BienvenidoControlador.java
Java
import gfg.multimodule.domain.entity.SalaryAccount; import gfg.multimodule.service.api.EmployeeAccountService; import java.util.Map; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; @Controller public class WelcomeController { @Value("${application.message:Hello World}") private String message = "Hello World"; @Autowired protected EmployeeAccountService accountService; @RequestMapping("/") public String welcome(Map<String, Object> model) { // Trying to obtain for account 100 SalaryAccount account = accountService.findOne("100"); if (account == null) { // If there's some problem creating account, // return show view with error status model.put("message", "Error getting account!"); model.put("account", ""); return "welcome/show"; } // Return show view with 100 account info // As a sample checking for account number 100 and // if available it will get redirected to show.html String accountInfo = "Your account number is ".concat( account.getEmployeeAccountNumber()); model.put("message", this.message); model.put("account", accountInfo); return "welcome/show"; } @RequestMapping("foo") public String foo(Map<String, Object> model) { throw new RuntimeException("Foo"); } }
show.html (una vez que la cuenta esté disponible, podemos ver el resultado de show.html)
HTML
<!DOCTYPE HTML> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Spring Boot Multimodule</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <style> #orangerow { background:orange; font:17 px "Times New Roman", serif; color:#000000; } #yellowrow { background:yellow; font:17 px "Times New Roman", serif; color:#000000; } </style> </head> <body> <table border="1"> <tr id="yellowrow"> <td> Welcome Message </td> <td> Account Details </td> </tr> <tr id="orangerow"> <td> <span th:text="${message}" /> </td> <td> <span th:text="${account}" /> </td> </tr> </table> </body> </html>
Para esta carpeta de aplicación, necesitamos tener un pom.xml específico que muestre las dependencias para este módulo
aplicación -> pom.xml
XML
<?xml version="1.0" encoding="UTF-8" standalone="no"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>gfg.multimodule</groupId> <artifactId>gfg.multimodule</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>gfg.multimodule.application</artifactId> <packaging>jar</packaging> <name>Project Module - Application</name> <description>This is the main module of the project. It contains Application.java class, that contains main method, necessary to run Spring Boot applications. It contains all necessary application configuration properties. It contains all web controllers, views and web resources. It include Service Implementation module as dependency that contains Model Module, Repository Module and Service Api Module. </description> <dependencies> <!-- Project modules --> <dependency> <groupId>gfg.multimodule</groupId> <artifactId>gfg.multimodule.service.impl</artifactId> <version>${project.version}</version> </dependency> <!-- Spring Boot dependencies --> <dependency> <groupId>org.apache.tomcat.embed</groupId> <artifactId>tomcat-embed-jasper</artifactId> <scope>provided</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> </dependencies> <build> <plugins> <!-- Spring Boot plugins --> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Siguiente módulo que vamos a ver como modelo (aquí tendremos nuestras clases de POJO/entidad y sus detalles relacionados).
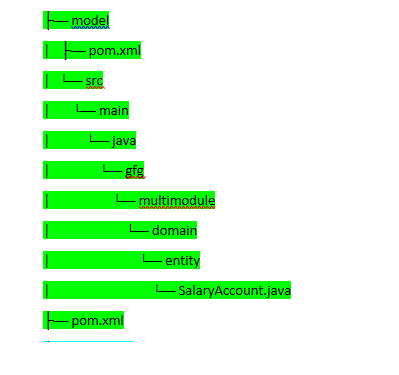
CuentaSalario.java
Java
import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class SalaryAccount { @Id @GeneratedValue(strategy = GenerationType.AUTO) // data members. Their getter and setter private Long employeeAccountId; private String employeeAccountNumber; private String type; private String creditCardNumber; public SalaryAccount() {} public SalaryAccount(Long employeeAccountId, String employeeAccountNumber) { this.employeeAccountNumber = employeeAccountNumber; this.employeeAccountId = employeeAccountId; } public Long getEmployeeAccountId() { return employeeAccountId; } public void setEmployeeAccountId(Long employeeAccountId) { this.employeeAccountId = employeeAccountId; } public String getEmployeeAccountNumber() { return employeeAccountNumber; } public void setEmployeeAccountNumber(String employeeAccountNumber) { this.employeeAccountNumber = employeeAccountNumber; } public String getType() { return type; } public void setType(String type) { this.type = type; } public String getCreditCardNumber() { return creditCardNumber; } public void setCreditCardNumber(String creditCardNumber) { this.creditCardNumber = creditCardNumber; } }
modelo -> pom.xml
XML
<?xml version="1.0" encoding="UTF-8" standalone="no"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>gfg.multimodule</groupId> <artifactId>gfg.multimodule</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>gfg.multimodule.model</artifactId> <packaging>jar</packaging> <name>Project Module - Model</name> <description>Module that contains all Entities and Visual Objects to be used in the project. It doesn't have any dependencies.</description> </project>
A continuación, veamos el módulo de repositorio . Este es el módulo donde podemos escribir nuestra interfaz de operaciones relacionadas con CRUD. Por lo general, tendrá funciones de búsqueda/inserción/actualización/eliminación
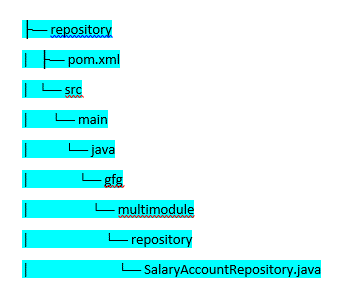
SalaryAccountRepository.java
Java
import gfg.multimodule.domain.entity.SalaryAccount; import org.springframework.data.repository.CrudRepository; import org.springframework.stereotype.Repository; @Repository // It should extends CrudRepository as we will have useful // methods related to CRUD operations public interface SalaryAccountRepository extends CrudRepository<SalaryAccount, Long> { SalaryAccount findByEmployeeAccountNumber( String employeeAccountNumber); }
pom.xml
XML
<?xml version="1.0" encoding="UTF-8" standalone="no"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>gfg.multimodule</groupId> <artifactId>gfg.multimodule</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>gfg.multimodule.repository</artifactId> <packaging>jar</packaging> <name>Project Module - Repository</name> <description>Module that contains all repositories to be used in the project. Depends of Model Module.</description> <dependencies> <!-- Project modules --> <dependency> <groupId>gfg.multimodule</groupId> <artifactId>gfg.multimodule.model</artifactId> <version>${project.version}</version> </dependency> <!-- Spring Boot dependencies --> <!-- Should specify the database that we are using --> <dependency> <groupId>org.hsqldb</groupId> <artifactId>hsqldb</artifactId> <scope>runtime</scope> </dependency> </dependencies> </project>
El siguiente es service-api. Los métodos relacionados con la lógica empresarial se presentan aquí.
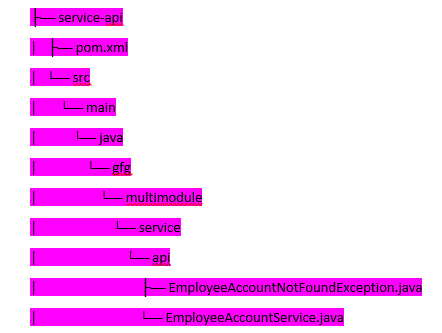
Archivos clave importantes
EmployeeAccountService.java
Java
package gfg.multimodule.service.api; import gfg.multimodule.domain.entity.SalaryAccount; import java.util.List; public interface EmployeeAccountService { SalaryAccount findOne(String number) throws EmployyeAccountNotFoundException; SalaryAccount createAccountByEmployeeAccountNumber(String number); }
EmployyeAccountNotFoundException.java
Java
public class EmployyeAccountNotFoundException extends RuntimeException { private static final long serialVersionUID = -3891534644498421234L; public EmployyeAccountNotFoundException( String accountId) { super("No such account with id: " + accountId); } }
El último es el de implementación. El servicio-impl Esto implementará los métodos de servicio (requisitos de lógica de negocios) y la implementación real se está realizando aquí.
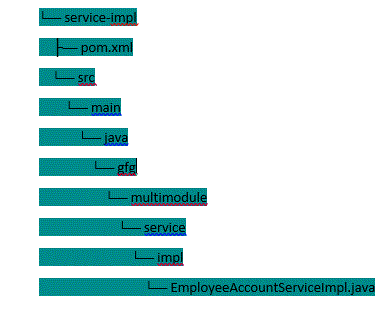
EmployeeAccountServiceImpl.java
Java
import gfg.multimodule.domain.entity.SalaryAccount; import gfg.multimodule.repository.SalaryAccountRepository; import gfg.multimodule.service.api.EmployeeAccountService; import gfg.multimodule.service.api.EmployyeAccountNotFoundException; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Service; @Service public class EmployeeAccountServiceImpl implements EmployeeAccountService { @Value("${dummy.type}") private String dummyType; @Autowired private SalaryAccountRepository accountRepository; @Override public SalaryAccount findOne(String employeeAccountNumber) throws EmployyeAccountNotFoundException { if (employeeAccountNumber.equals("0000")) { throw new EmployyeAccountNotFoundException( "0000"); } SalaryAccount salaryAccount = accountRepository.findByEmployeeAccountNumber( employeeAccountNumber); if (salaryAccount == null) { salaryAccount = createAccountByEmployeeAccountNumber( employeeAccountNumber); } return salaryAccount; } @Override public SalaryAccount createAccountByEmployeeAccountNumber( String employeeAccountNumber) { SalaryAccount account = new SalaryAccount(); account.setEmployeeAccountNumber( employeeAccountNumber); return accountRepository.save(account); } public String getDummyType() { return dummyType; } public void setDummyType(String dummyType) { this.dummyType = dummyType; } }
Ahora veamos cómo ejecutar todo el proyecto. Se debe proporcionar » mvn clean install » donde se alojó el proyecto (carpeta principal donde pom, xml) presente
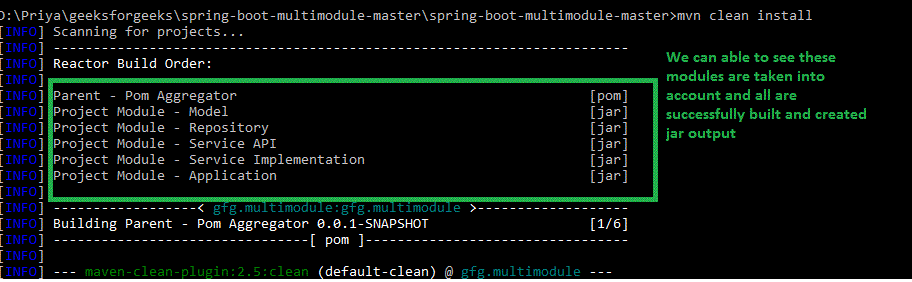
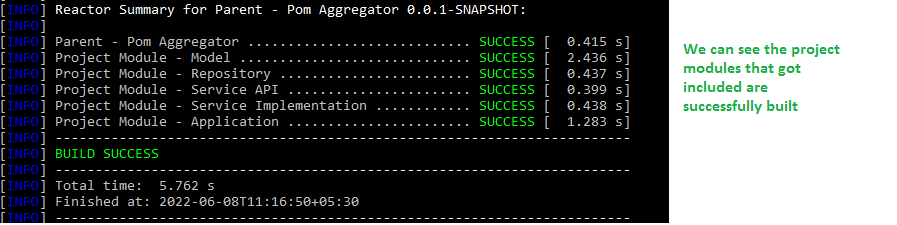
Ahora debemos avanzar a la carpeta de la aplicación y ejecutarla como mvn spring-boot:run
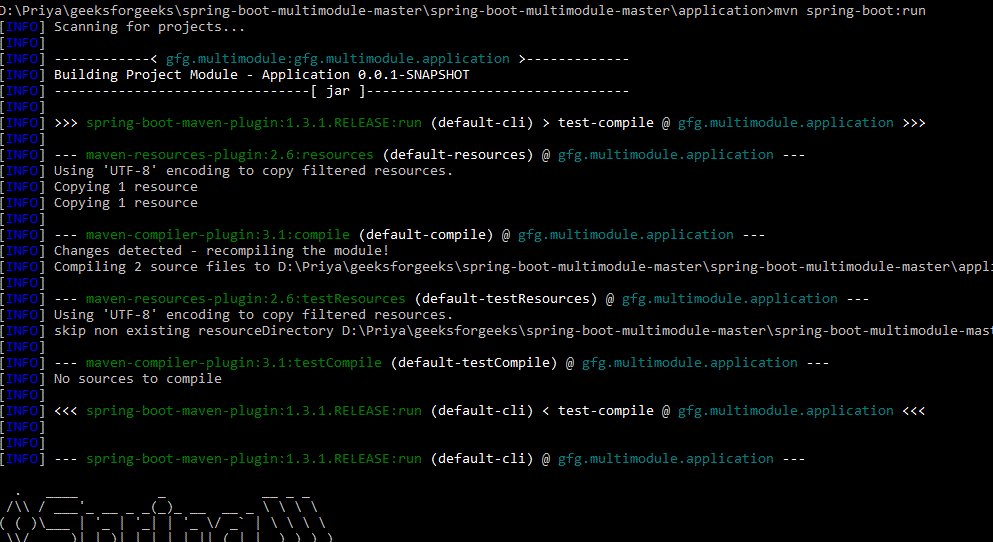
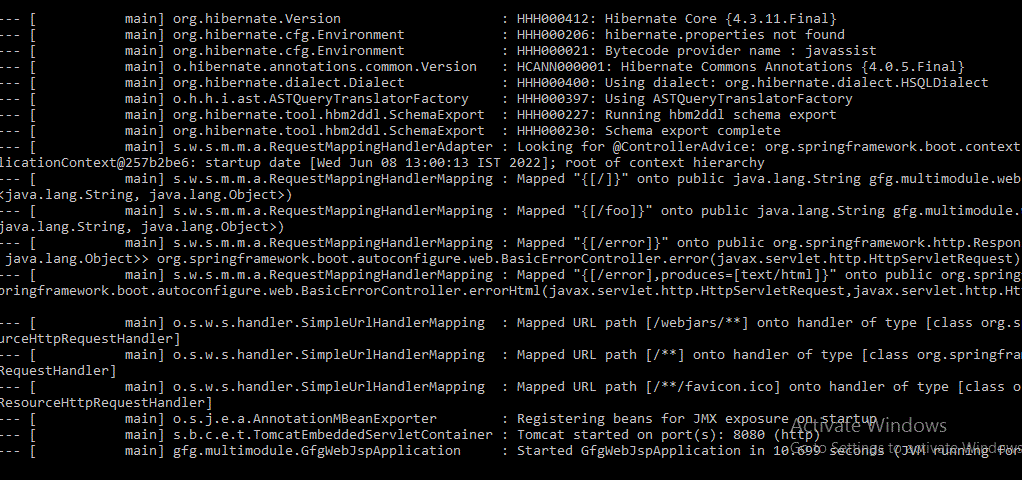
En el inicio exitoso (se otorga la dependencia de Tomcat y se ejecuta en el puerto 8080)
http://localhost:8080/
Podemos ver como
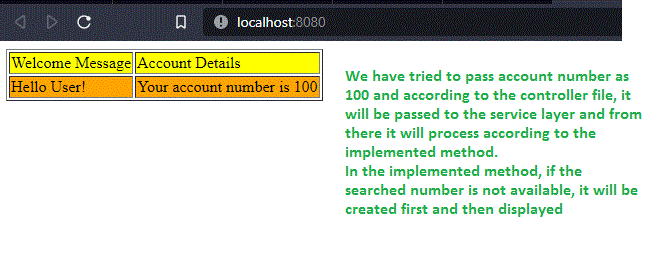
Al combinar diferentes módulos de proyecto, como un proyecto maven, debemos incluir todos los módulos y todas las dependencias requeridas deben proporcionarse, luego todo el proyecto se ejecuta con éxito y obtendremos el resultado como se indica arriba.
Publicación traducida automáticamente
Artículo escrito por priyarajtt y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA