Requisitos previos:
- Fundamentos de desarrollo de aplicaciones de Android para principiantes
- Guía para instalar y configurar Android Studio
- android | Comenzando con la primera aplicación/proyecto de Android
- android | Ejecutando tu primera aplicación de Android
En este artículo, aprenderemos cómo crear una aplicación usando Kotlin donde los memes se obtendrán de Reddit usando una API y se mostrarán en la pantalla. Estos memes también se pueden compartir usando otras aplicaciones como (WhatsApp, Facebook, etc.) con tus amigos. Aprenderemos cómo hacer llamadas a la API y cómo usar las bibliotecas Volley y Glide en nuestro proyecto. Tenga en cuenta que vamos a implementar este proyecto utilizando el lenguaje Kotlin. A continuación se muestra un video de muestra para tener una idea de lo que vamos a hacer en este artículo.
Implementación paso a paso
Paso 1: crear un nuevo proyecto
Para crear un nuevo proyecto en Android Studio, consulte Cómo crear/iniciar un nuevo proyecto en Android Studio . Tenga en cuenta que seleccione Kotlin como lenguaje de programación.
Paso 2: trabajar con el archivo activity_main.xml
Vaya a la aplicación > res > diseño > actividad_principal.xml y haga el siguiente diseño o agregue el siguiente código a ese archivo. A continuación se muestra el código para el archivo activity_main.xml.
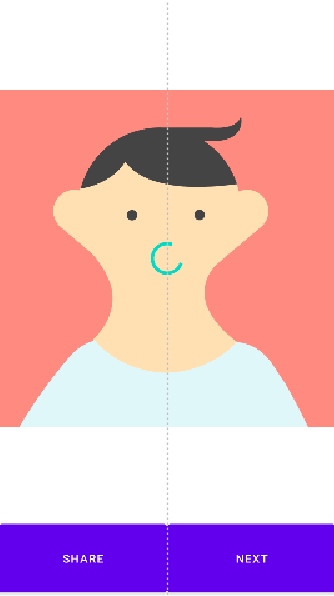
Diseño para la aplicación Meme Share
XML
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <!--Layout where image will be loaded--> <ImageView android:id="@+id/memeImageView" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintBottom_toTopOf="@id/shareButton" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" tools:srcCompat="@tools:sample/avatars"/> <!--Progress bar which gets disabled whenever meme is loaded--> <ProgressBar android:id="@+id/progressBar" android:layout_width="wrap_content" android:layout_height="wrap_content" app:layout_constraintBottom_toBottomOf="@id/memeImageView" app:layout_constraintLeft_toLeftOf="@id/memeImageView" app:layout_constraintRight_toRightOf="@id/memeImageView" app:layout_constraintTop_toTopOf="@id/memeImageView" /> <!--Next Button which loads another meme--> <Button android:id="@+id/nextButton" android:layout_width="0dp" android:layout_height="wrap_content" android:onClick="nextmeme" android:text="NEXT" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintLeft_toRightOf="@id/guideline" android:padding="32dp" tools:ignore="OnClick" /> <!--Share button which allows you to share memes--> <Button android:id="@+id/shareButton" android:layout_width="0dp" android:layout_height="wrap_content" android:text="SHARE" android:onClick="sharememe" android:padding="32dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toLeftOf="@id/guideline" tools:ignore="OnClick" /> <androidx.constraintlayout.widget.Guideline android:id="@+id/guideline" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical" app:layout_constraintGuide_percent="0.5"/> </androidx.constraintlayout.widget.ConstraintLayout>
Paso 3: Agregar dependencia y modificar AndroidManifest.xml
Busque una API que tenga muchos memes, por ejemplo, usaremos esta API . Para cargar los memes desde la API, usaremos la biblioteca Volley de la que obtendremos una URL del objeto JSON, para convertir esa URL en una imagen real usaremos la biblioteca Glide . Navegue a Gradle Scripts> build.gradle(Module : appname.app) y agregue las siguientes dependencias
Kotlin
implementation 'com.android.volley:volley:1.2.1' implementation 'com.github.bumptech.glide:glide:4.12.0' annotationProcessor 'com.github.bumptech.glide:compiler:4.11.0'
Vaya a la aplicación > manifiestos > AndroidManifest.xml y agregue permiso de Internet al manifiesto de su aplicación ; sin esto, no podremos conectarnos a Internet.
<uses-permission android:name="android.permission.INTERNET" />
Paso 4: trabajar con el archivo MainActivity.kt
Ahora, abra el archivo MainActivity.kt aquí, necesita crear tres funciones:-
cargarmeme()
Carga un meme de la API usando Volley y lo muestra en la pantalla de la aplicación.
Kotlin
private fun loadmeme() { // Initializing a progress bar which // gets disabled whenever image is loaded. val progressBar: ProgressBar =findViewById(R.id.progressBar) progressBar.visibility= View.VISIBLE val meme: ImageView =findViewById(R.id.memeImageView) // Instantiate the RequestQueue. val queue = Volley.newRequestQueue(this) val url = "https://meme-api.herokuapp.com/gimme" // Request a JSON object response from the provided URL. val jsonObjectRequest = JsonObjectRequest( Request.Method.GET, url, null, { response -> // Storing the url of meme from the API. presentImageUrl = response.getString("url") // Displaying it with the use of Glide. Glide.with(this).load(presentImageUrl).listener(object: RequestListener<Drawable> { override fun onLoadFailed( e: GlideException?, model: Any?, target: Target<Drawable>?, isFirstResource: Boolean ): Boolean { progressBar.visibility= View.GONE return false } override fun onResourceReady( resource: Drawable?, model: Any?, target: com.bumptech.glide.request.target.Target<Drawable>?, dataSource: com.bumptech.glide.load.DataSource?, isFirstResource: Boolean ): Boolean { progressBar.visibility= View.GONE return false } }).into(meme) } ) { Toast.makeText(this, "Something went wrong", Toast.LENGTH_LONG).show() } // Add the request to the RequestQueue. queue.add(jsonObjectRequest) }
siguientememe()
Cargará otro meme cada vez que hagamos clic en el botón SIGUIENTE en la pantalla de la aplicación.
Kotlin
fun nextmeme(view: View) { // Calling loadmeme() whenever // Next button is clicked. loadmeme() }
compartirmeme()
Nos permite compartir la URL del meme a otras plataformas como WhatsApp, Fb, etc. haciendo clic en el botón COMPARTIR en la pantalla de la aplicación.
Kotlin
fun sharememe(view: View) { // Creating an Intent to share // the meme with other apps. val intent= Intent(Intent.ACTION_SEND) intent.type="text/plain" intent.putExtra(Intent.EXTRA_TEXT, "Hey, Checkout this cool meme $presentImageUrl") val chooser = Intent.createChooser(intent, "Share this meme") startActivity(chooser) }
Código completo del archivo MainActivity.kt
Kotlin
package com.example.gfgmeme import android.content.Intent import android.graphics.drawable.Drawable import android.os.Bundle import android.view.View import android.widget.ImageView import android.widget.ProgressBar import android.widget.Toast import androidx.appcompat.app.AppCompatActivity import com.android.volley.Request import com.android.volley.toolbox.JsonObjectRequest import com.android.volley.toolbox.Volley import com.bumptech.glide.Glide import com.bumptech.glide.load.engine.GlideException import com.bumptech.glide.request.RequestListener import com.bumptech.glide.request.target.Target class MainActivity : AppCompatActivity() { var presentImageUrl: String?=null override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // Calling loadmeme() to display the meme. loadmeme() } private fun loadmeme() { // Initializing a progress bar which // gets disabled whenever image is loaded. val progressBar: ProgressBar =findViewById(R.id.progressBar) progressBar.visibility= View.VISIBLE val meme: ImageView =findViewById(R.id.memeImageView) // Instantiate the RequestQueue. val queue = Volley.newRequestQueue(this) val url = "https://meme-api.herokuapp.com/gimme" // Request a JSON object response from the provided URL. val jsonObjectRequest = JsonObjectRequest( Request.Method.GET, url, null, { response -> // Storing the url of meme from the API. presentImageUrl = response.getString("url") // Displaying it with the use of Glide. Glide.with(this).load(presentImageUrl).listener(object: RequestListener<Drawable> { override fun onLoadFailed( e: GlideException?, model: Any?, target: Target<Drawable>?, isFirstResource: Boolean ): Boolean { progressBar.visibility= View.GONE return false } override fun onResourceReady( resource: Drawable?, model: Any?, target: com.bumptech.glide.request.target.Target<Drawable>?, dataSource: com.bumptech.glide.load.DataSource?, isFirstResource: Boolean ): Boolean { progressBar.visibility= View.GONE return false } }).into(meme) } ) { Toast.makeText(this, "Something went wrong", Toast.LENGTH_LONG).show() } // Add the request to the RequestQueue. queue.add(jsonObjectRequest) } fun nextmeme(view: View) { // Calling loadmeme() whenever // Next button is clicked. loadmeme() } fun sharememe(view: View) { // Creating an Intent to share // the meme with other apps. val intent= Intent(Intent.ACTION_SEND) intent.type="text/plain" intent.putExtra(Intent.EXTRA_TEXT, "Hey, Checkout this cool meme $presentImageUrl") val chooser = Intent.createChooser(intent, "Share this meme") startActivity(chooser) } }
Producción:
Publicación traducida automáticamente
Artículo escrito por yashguptaaa333 y traducido por Barcelona Geeks. The original can be accessed here. Licence: CCBY-SA